Gestión de compras
This tutorial shows how to use the SDK methods to display the following items in an in-game store:
- virtual items
- groups of virtual items
- bundles
- packages of virtual currency
Before you start, configure items in Publisher Account:
- Configure virtual items and groups of virtual items.
- Configure packages of virtual currencies.
- Configure bundles.
This tutorial describes the implementation of the following logic:
The logics and interface in the examples are less complicated than they will be in your application. A possible item catalog in an in-game store implementation option is described in the demo project.
The example of every item in a catalog shows:
- item name
- item description
- item price
- image
You can also show other information about the item if this information is stored in an in-game store.
Implementar la visualización de artículos virtuales
Crear widget de artículo
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- item background image
- item name
- item description
- item price
- item image
The following picture shows an example of the widget structure.
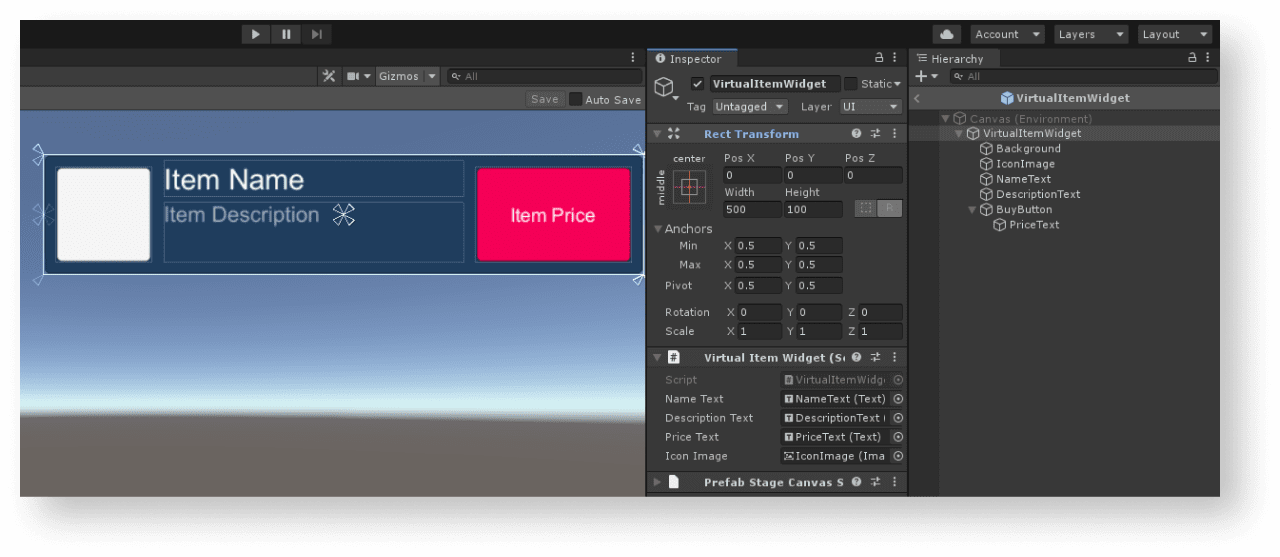
Crear script de widget de artículo
- Create a script
VirtualItemWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualItemWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Image IconImage;
}
}
Crear página para mostrar lista de artículos
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- item widgets display area
The following picture shows an example of the page structure.
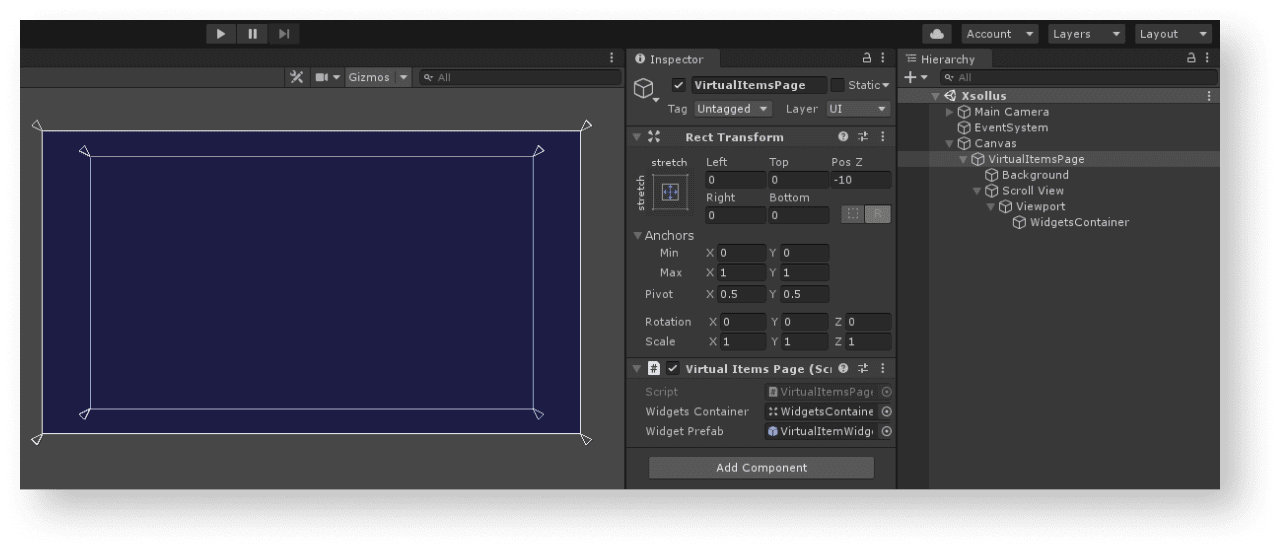
Crear controlador de página
- Create a script
VirtualItemsPage
inherited from theMonoBehaviour
base class. - Declare the following variables:
WidgetsContainer
— container for widgetsWidgetPrefab
— item widget prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aVirtualItemsPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel.
- Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:- a username or email address in the
username
parameter - a user password in the
password
parameter
- a username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logics for getting the list of items. In the
OnAuthenticationSuccess
method call theXsollaStore.Instance.GetCatalog
SDK method and pass to it:- a Project ID in the
projectId
parameter
- a Project ID in the
- the
OnItemsRequestSuccess
for successful operation of getting a list of items - the
OnError
callback method for an error - an offset based on the first item in the list in the
offset
parameter - the number of loaded items in the
limit
parameter
- the
offset
and limit
parameters are not required. Use them to implement pagination — a page-by-page display of items in the catalog. The maximum number of items on the page is 50. If the catalog has more than 50 items, pagination is necessary.- In the
OnItemsRequestSuccess
method, add logics for creating a widget for every received item:- Instantiate a prefab of item widget as a container child object.
- Attach the received
VirtualItemWidget
component to awidget
variable.
- Pass the following data to the item widget:
- Pass the
storeItem.name
variable value to the element with the item name. - Pass the
storeItem.description
variable value to the element with the item description. - Implement the following logics to display the item price:
- If the value of the
storeItem.price
variable doesn’t equalnull
, the item is sold for real currency. Specify the price in the{amount} {currency}
format and pass it to the widget element. - If the value of the
storeItem.virtual_prices
variable doesn’t equalnull
, the item is sold for virtual currency. Specify the price in the{name}: {amount}
format and pass it to the widget element.
- If the value of the
- Pass the
storeItem.virtual_prices
variable is an array of prices for the same item in different currencies. The example shows a price specified by default in the item settings in Store > Virtual items in Publisher Account.- To display an item image, use the
ImageLoader.Instance.GetImageAsync
utility method and pass to it:- Image URL.
- An anonymous function as a callback. In this function, add a received sprite as an item image.
- To display an item image, use the
Example of a page controller script:
- C#
uusing System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualItemsPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, OnAuthenticationSuccess, OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for catalog from store
XsollaStore.Instance.GetCatalog(XsollaSettings.StoreProjectId, OnItemsRequestSuccess, OnError, offset: 0, limit: 50);
}
private void OnItemsRequestSuccess(StoreItems storeItems)
{
// Iterating the items collection and assign values for appropriate ui elements
foreach (var storeItem in storeItems.items)
{
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<VirtualItemWidget>();
widget.NameText.text = storeItem.name;
widget.DescriptionText.text = storeItem.description;
if (storeItem.price != null)
{
var realMoneyPrice = storeItem.price;
widget.PriceText.text = $"{realMoneyPrice.amount} {realMoneyPrice.currency}";
}
else if (storeItem.virtual_prices != null)
{
var virtualCurrencyPrice = storeItem.virtual_prices.First(x => x.is_default);
widget.PriceText.text = $"{virtualCurrencyPrice.name}: {virtualCurrencyPrice.amount}";
}
ImageLoader.Instance.GetImageAsync(storeItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
The following picture shows the result of the script’s work.
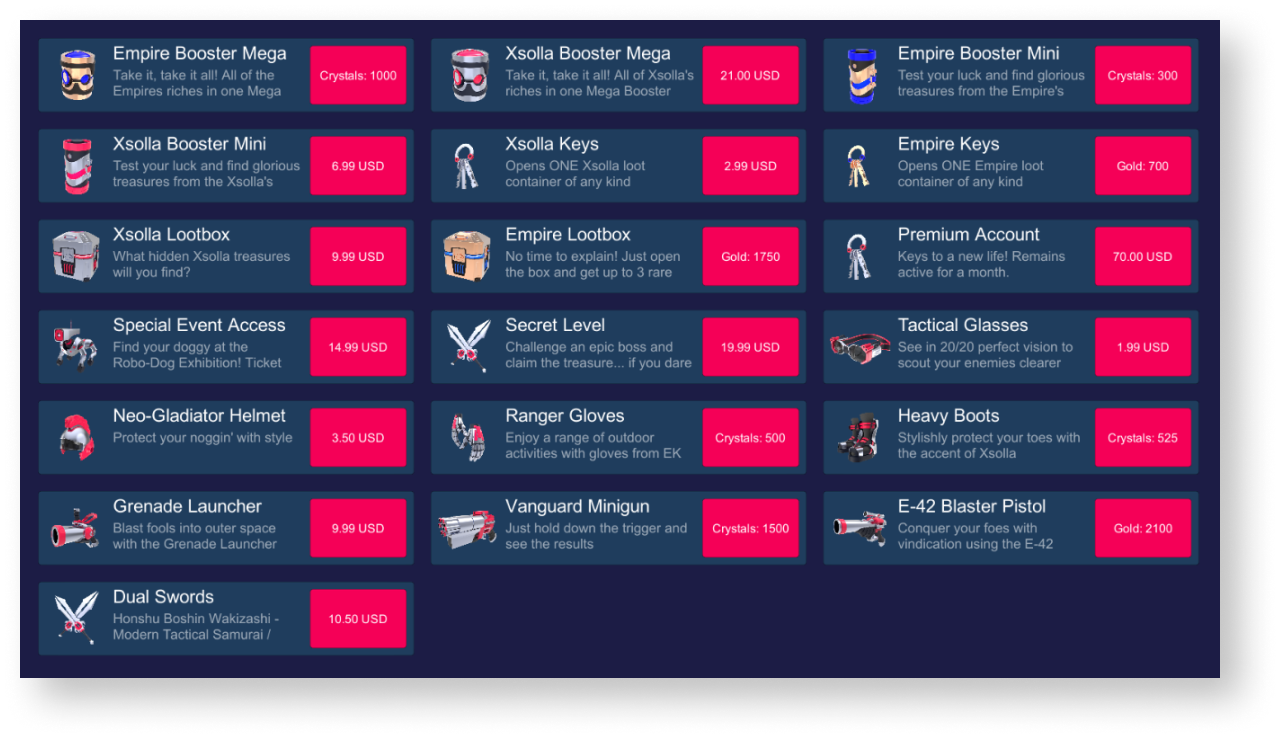
Implementar la visualización de grupos de artículos virtuales
Crear widget de artículo
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- item background image
- item name
- item description
- item price
- item image
The following picture shows an example of the widget structure.
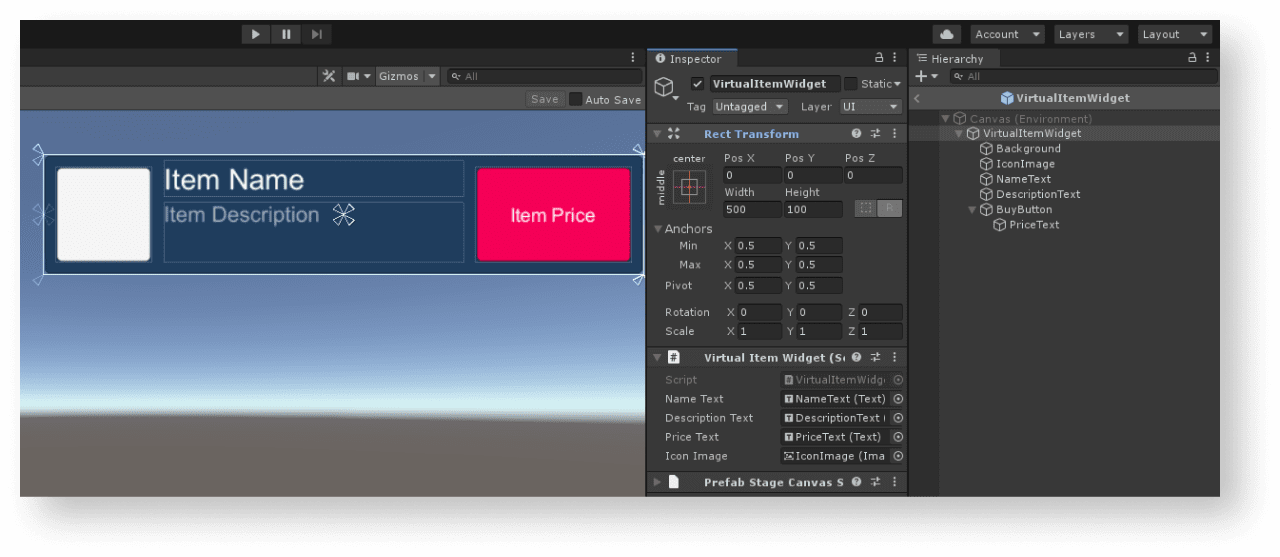
Crear script de widget de artículo
- Create a script
VirtualItemWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualItemWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Image IconImage;
}
}
Crear widget para el botón que abre grupos de artículos
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the button that allows displaying of the group of items as a child object for a prefab and configure its visuals.
The following picture shows an example of the widget structure.
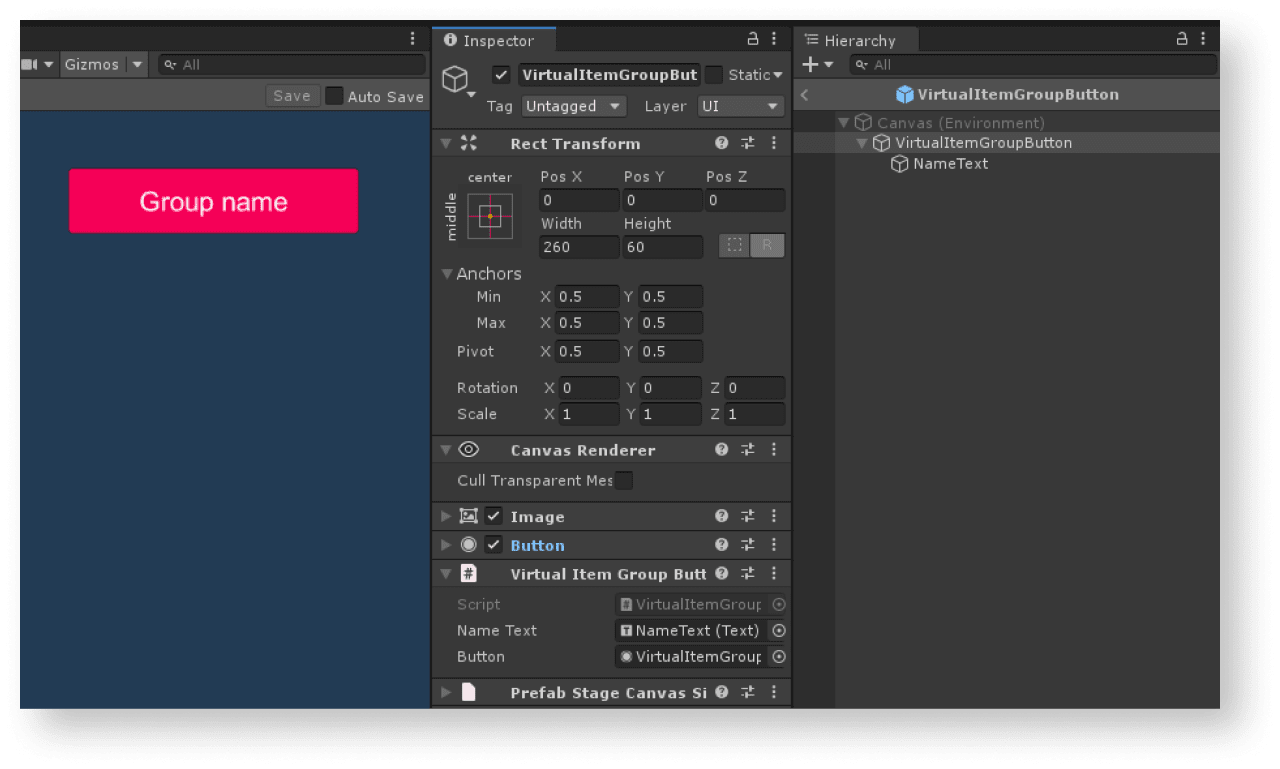
Crear script para el botón que abre grupos de artículos
- Create the
VirtualItemGroupButton
script inherited from theMonoBehaviour
base class. - Declare variables for the button that opens the group of items and set values for the variables in the
Inspector panel. - Add a script to the root object of a prefab:
- Select an object in the
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aVirtualItemGroupButton
script.
- Select an object in the
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualItemGroupButton : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Button Button;
}
}
Crear página para mostrar lista de artículos
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- item groups buttons display area
- item widgets display area
The following picture shows an example of the page structure.
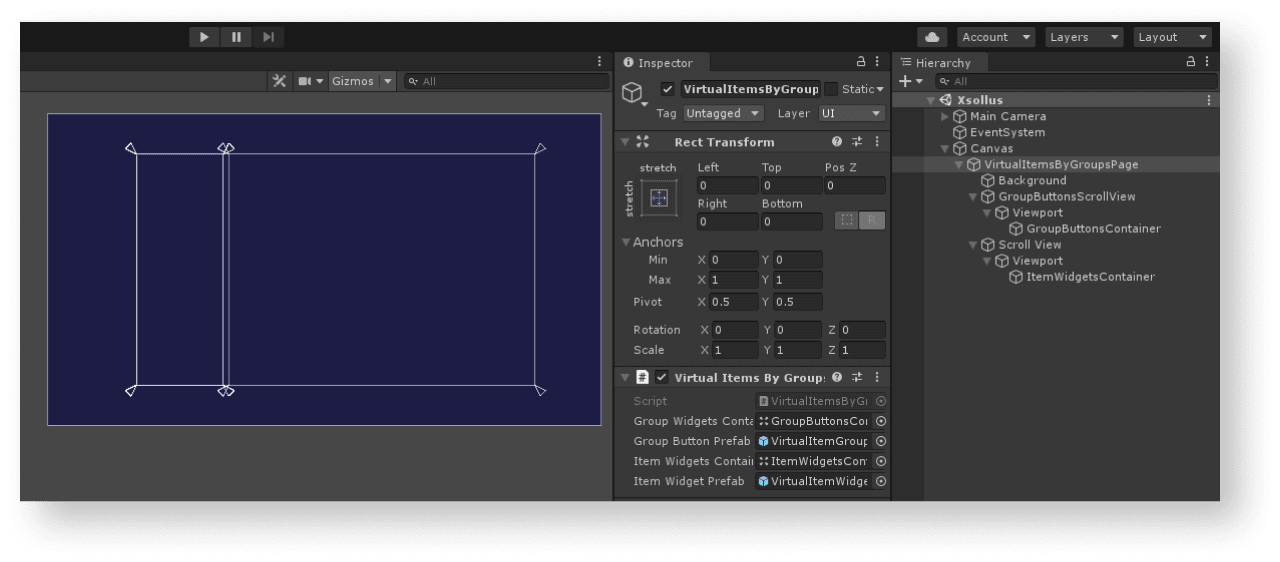
Crear controlador de página
- Create the
VirtualItemsByGroupsPage
script inherited from theMonoBehaviour
base class. - Declare variables:
GroupButtonsContainer
— container for group buttonsGroupButtonPrefab
— button prefabItemWidgetsContainer
— container for item widgetsWidgetPrefab
— item widget prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aVirtualItemsByGroupsPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel. - Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:- a username or email address in the
username
parameter - a user password in the
password
parameter
- a username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logics for getting the list of items. In the
OnAuthenticationSuccess
method call theXsollaStore.Instance.GetCatalog
SDK method and pass to it:- a Project ID in the
projectId
parameter
- a Project ID in the
- the
OnItemsRequestSuccess
for successful operation of getting a list of items - the
OnError
callback method for an error - an offset based on the first item in the list in the
offset
parameter - the number of loaded items in the
limit
parameter
- the
offset
and limit
parameters are not required. Use them to implement pagination — a page-by-page display of items in the catalog. The maximum number of items on the page is 50. If the catalog has more than 50 items, pagination is necessary.- In the
OnItemsRequestSuccess
method, add logics for forming a list of item groups:- Get the list of unique groups from a received item list. Add to it the
All
element that will show all items not dependent on their category. - Clear the buttons container by deleting all child objects. To do this, call the
DeleteAllChildren
method and pass a container object to it. - For every item group:
- Get the list of unique groups from a received item list. Add to it the
- Instantiate a prefab of item widget as a container child object.
- Set the received
VirtualItemGroupButton
component to thegroupButton
variable. - Pass the
groupName
variable value to the element with a group name. - Add an anonymous method to the action of clicking the button. In this method, call the
OnGroupSelected
method and pass the name of the item group and the list of items as parameters.
- To display all items call the
OnGroupSelected
method and passAll
as a group name.
- To display all items call the
- In the
OnGroupSelected
method, add logics for initial display of items:- Create the
itemsForDisplay
variable and assign all received items to it if the name of the item group hasAll
. Otherwise, link items that the group name matches with thegroupName
variable to theitemsForDisplay
variable. - Clear the buttons container by deleting all child objects. To do this, call the
DeleteAllChildren
method and pass a container object to it.
- Create the
- Add logics for creating a widget for every received item:
- Instantiate a prefab of item widget as a container child object.
- Attach the received
VirtualItemWidget
component to awidget
variable.
- Pass the following data to the item widget:
- Pass the
storeItem.name
variable value to the element with the item name. - Pass the
storeItem.description
variable value to the element with the item description. - Implement the following logics to display item price:
- Pass the
- If the value of the
storeItem.price
variable doesn’t equalnull
, the item is sold for real currency. Specify the price in the{amount} {currency}
format and pass it to the widget element. - If the value of the
storeItem.virtual_prices
variable doesn’t equalnull
, the item is sold for virtual currency. Specify the price in the{name}: {amount}
format and pass it to the widget element.
- If the value of the
storeItem.virtual_prices
variable is an array of prices for the same item in different currencies. The example shows a price specified by default in the item settings in Store > Virtual items in Publisher Account.- To display an item image, use the
ImageLoader.Instance.GetImageAsync
utility method and pass to it:- Image URL.
- An anonymous function as a callback. In this function, add a received sprite as an item image.
- To display an item image, use the
- C#
using System.Collections.Generic;
using System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualItemsByGroupsPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform GroupButtonsContainer;
public GameObject GroupButtonPrefab;
public Transform ItemWidgetsContainer;
public GameObject ItemWidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, onSuccess: OnAuthenticationSuccess, onError: OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for catalog from store
XsollaStore.Instance.GetCatalog(XsollaSettings.StoreProjectId, OnItemsRequestSuccess, OnError, offset: 0, limit: 50);
}
private void OnItemsRequestSuccess(StoreItems storeItems)
{
// Selecting the group’s name from items and order them alphabetical
var groupNames = storeItems.items
.SelectMany(x => x.groups)
.GroupBy(x => x.name)
.Select(x => x.First())
.OrderBy(x => x.name)
.Select(x => x.name)
.ToList();
// Add group name for “all groups”, which will mean show all items regardless of group affiliation
groupNames.Insert(0, "All");
// Clear container
DeleteAllChildren(GroupButtonsContainer);
// Iterating the group names and creating ui-button for each
foreach (var groupName in groupNames)
{
var buttonObj = Instantiate(GroupButtonPrefab, GroupButtonsContainer, false);
var groupButton = buttonObj.GetComponent<VirtualItemGroupButton>();
groupButton.NameText.text = groupName;
groupButton.Button.onClick.AddListener(() => OnGroupSelected(groupName, storeItems));
}
// Calling method for redraw page
OnGroupSelected("All", storeItems);
}
private void OnGroupSelected(string groupName, StoreItems storeItems)
{
// Declaring variable for items which will display on page
IEnumerable<StoreItem> itemsForDisplay;
if (groupName == "All")
{
itemsForDisplay = storeItems.items;
}
else
{
itemsForDisplay = storeItems.items.Where(item => item.groups.Any(group => group.name == groupName));
}
// Clear container
DeleteAllChildren(ItemWidgetsContainer);
// Iterating the items collection and assign values for appropriate ui elements
foreach (var storeItem in itemsForDisplay)
{
var widgetGo = Instantiate(ItemWidgetPrefab, ItemWidgetsContainer, false);
var widget = widgetGo.GetComponent<VirtualItemWidget>();
widget.NameText.text = storeItem.name;
widget.DescriptionText.text = storeItem.description;
if (storeItem.price != null)
{
var realMoneyPrice = storeItem.price;
widget.PriceText.text = $"{realMoneyPrice.amount} {realMoneyPrice.currency}";
}
else if (storeItem.virtual_prices != null)
{
var virtualCurrencyPrice = storeItem.virtual_prices.First(x => x.is_default);
widget.PriceText.text = $"{virtualCurrencyPrice.name}: {virtualCurrencyPrice.amount}";
}
ImageLoader.Instance.GetImageAsync(storeItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
// Utility method for delete all children of container
private static void DeleteAllChildren(Transform parent)
{
var childList = parent.Cast<Transform>().ToList();
foreach (var childTransform in childList)
{
Destroy(childTransform.gameObject);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
Example of a page controller script:
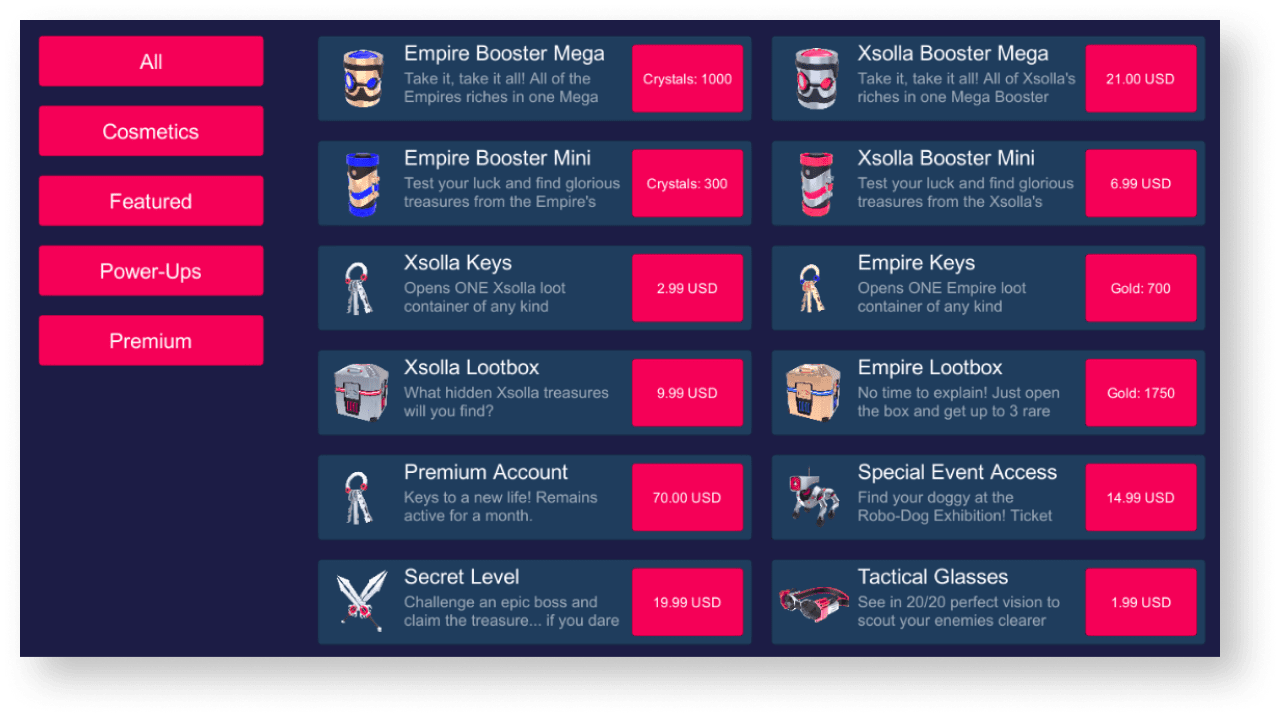
Implementar la visualización de lotes
Crear widget del lote
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- widget background image
- bundle name
- bundle description
- bundle price
- bundle content description (items and their quantity)
- bundle image
The following picture shows an example of the widget structure.
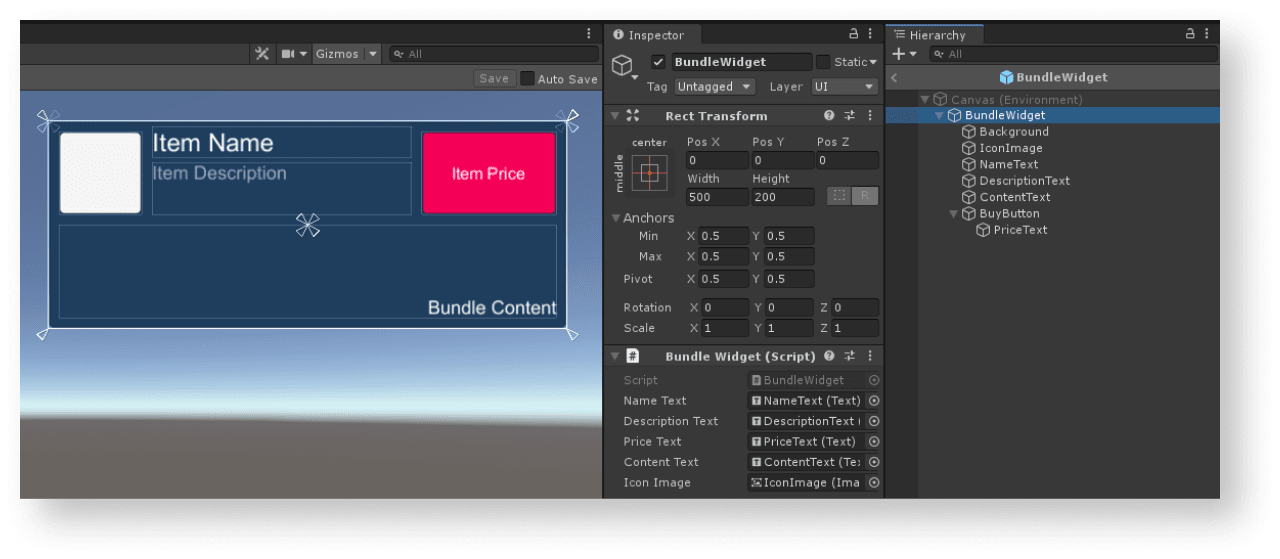
Crear script de widget
- Create a script
BundleWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class BundleWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Text ContentText;
public Image IconImage;
}
}
Crear página para mostrar lotes de artículos
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- bundle widgets display area
The following picture shows an example of the page structure.
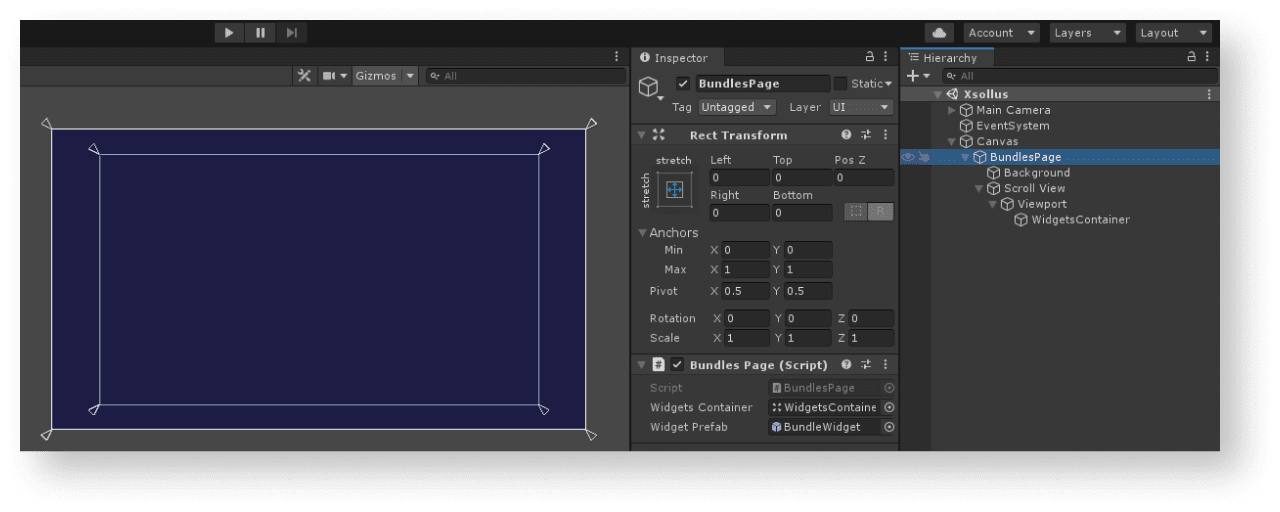
Crear controlador de página
- Create the
BundlesPage
script inherited from theMonoBehaviour
base class. - Declare variables:
WidgetsContainer
— container for widgetsWidgetPrefab
— bundle widget prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aBundlesPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel. - Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:- a username or email address in the
username
parameter - a user password in the
password
parameter
- a username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logics for getting the list of bundles. In the
OnAuthenticationSuccess
method call theXsollaStore.Instance.GetBundles
SDK method and pass to it:- a Project ID in the
projectId
parameter
- a Project ID in the
- the
OnItemsRequestSuccess
callback method for successful operation of getting a list of bundles - the
OnError
callback method for an error
- the
- In the
OnBundlesRequestSuccess
method, add logics for creating a widget for every received bundles:- Instantiate a prefab of item widget as a container child object.
- Attach the received
BundleWidget
component to awidget
variable.
- Pass the following data to the bundle widget:
- Pass the
bundleItem.name
variable value to the element with the item name. - Pass the
bundleItem.description
variable value to the element with the item description. - Implement the following logics to display bundle content:
- Use every item in a bundle to form a line that contains the item name and its quantity. The line should have a
{name} x {quantity}
format. - Group these lines into one line by using a new line character as a separator.
- Pass the new line to the widget element.
- Use every item in a bundle to form a line that contains the item name and its quantity. The line should have a
- Pass the
- Implement the following logics to display bundle price:
- If the value of the
bundleItem.price
variable doesn’t equalnull
, the bundle is sold for real currency. Specify the price in the{amount} {currency}
format and pass it to the widget element. - If the value of the
bundleItem.virtual_prices
variable doesn’t equalnull
, the bundle is sold for virtual currency. Specify the price in the{name}: {amount}
format and pass it to the widget element.
- If the value of the
- Implement the following logics to display bundle price:
bundleItem.virtual_prices
variable is an array of prices for the same bundle in different currencies. The example shows a price specified by default in the item settings in Store > Bundles in Publisher Account.- To display an item image, use the
ImageLoader.Instance.GetImageAsync
utility method and pass to it:- Image URL.
- An anonymous function as a callback. In this function, add a received sprite as a bundle image.
- To display an item image, use the
Example of a page controller script:
- C#
using System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class BundlesPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, onSuccess: OnAuthenticationSuccess, onError: OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for bundles from store
XsollaStore.Instance.GetBundles(XsollaSettings.StoreProjectId, OnBundlesRequestSuccess, OnError);
}
private void OnBundlesRequestSuccess(BundleItems bundleItems)
{
// Iterating the bundles collection and assign values for appropriate ui elements
foreach (var bundleItem in bundleItems.items)
{
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<BundleWidget>();
widget.NameText.text = bundleItem.name;
widget.DescriptionText.text = bundleItem.description;
var bundleContent = bundleItem.content.Select(x => $"{x.name} x {x.quantity}");
widget.ContentText.text = string.Join("\n", bundleContent);
if (bundleItem.price != null)
{
var realMoneyPrice = bundleItem.price;
widget.PriceText.text = $"{realMoneyPrice.amount} {realMoneyPrice.currency}";
}
else if (bundleItem.virtual_prices != null)
{
var virtualCurrencyPrice = bundleItem.virtual_prices.First(x => x.is_default);
widget.PriceText.text = $"{virtualCurrencyPrice.name}: {virtualCurrencyPrice.amount}";
}
ImageLoader.Instance.GetImageAsync(bundleItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
The following picture shows the result of the script’s work.
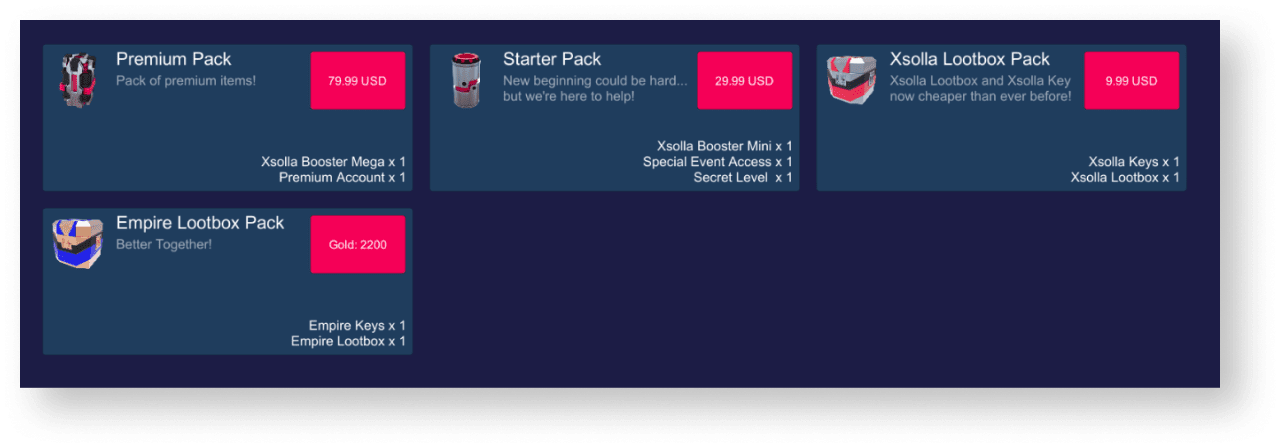
Implementar la visualización de paquetes de monedas virtuales
Crear un widget para el paquete de moneda virtual
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- widget background image
- package name
- package description
- package price
- package image
The following picture shows an example of the widget structure.
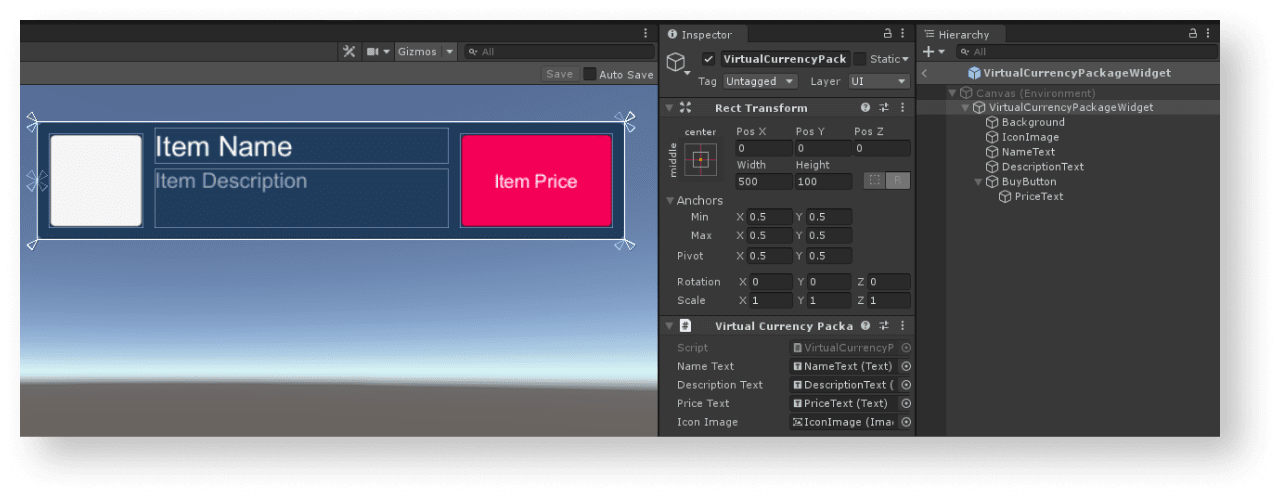
Crear script de widget
- Create a script
VirtualCurrencyPackageWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualCurrencyPackageWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Image IconImage;
}
}
Crear una página para mostrar la lista de paquetes de monedas virtuales
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- virtual currency package widgets display area
The following picture shows an example of the page structure.
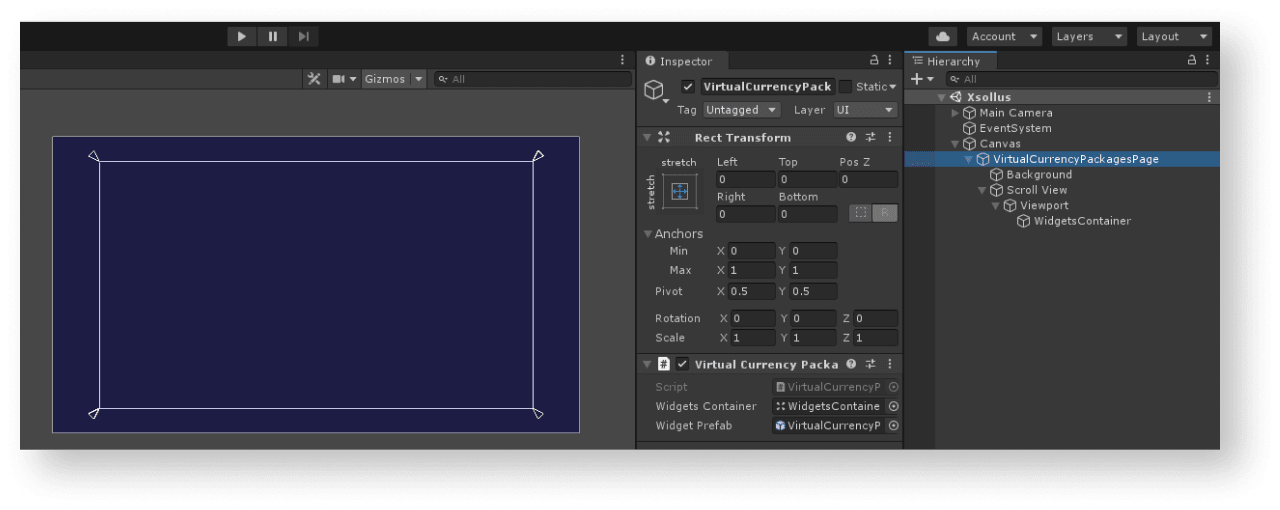
Crear controlador de página
- Create the
VirtualCurrencyPackagesPage
script inherited from theMonoBehaviour
base class. - Declare variables:
WidgetsContainer
— container for widgetsWidgetPrefab
— virtual currency package prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aVirtualCurrencyPackagesPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel. - Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:
- username or email address in the
username
parameter - user password in the
password
parameter
- username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logics for getting the list of items. In the
OnAuthenticationSuccess
method call theXsollaStore.Instance.GetVirtualCurrencyPackagesList
SDK method and pass to it:- Project ID in the
projectId
parameter
- Project ID in the
- the
OnItemsRequestSuccess
for successful operation of getting a list of items - the
OnError
callback method for an error
- the
- In the
OnPackagesRequestSuccess
method, add logics for creating a widget for every received package:- Instantiate a prefab of item widget as a container child object.
- Attach the received
VirtualCurrencyPackageWidget
component to awidget
variable.
- Pass the following data to the package widget:
- Pass the
packageItem.name
variable value to the element with the package name. - Pass the
packageItem.description
variable value to the element with the package description. - Implement the following logics to display package price:
- Pass the
- If the value of the
packageItem.price
variable doesn’t equalnull
, the package is sold for real currency. Specify the price in the{amount} {currency}
format and pass it to the widget element. - If the value of the
packageItem.virtual_prices
variable doesn’t equalnull
, the package is sold for virtual currency. Specify the price in the{name}: {amount}
format and pass it to the widget element.
- If the value of the
packageItem.virtual_prices
variable is an array of prices for the same package in different currencies. The example shows a price specified by default in the package settings in Store > Virtual currency > Packages in Publisher Account.- To display an item image, use the
ImageLoader.Instance.GetImageAsync
utility method and pass to it:- Image URL.
- An anonymous function as a callback. In this function, add a received sprite as an item image.
- To display an item image, use the
Example of a page controller script:
- C#
using System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualCurrencyPackagesPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, onSuccess: OnAuthenticationSuccess, onError: OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for packages from store
XsollaStore.Instance.GetVirtualCurrencyPackagesList(XsollaSettings.StoreProjectId, OnPackagesRequestSuccess, OnError);
}
private void OnPackagesRequestSuccess(VirtualCurrencyPackages packageItems)
{
// Iterating the packages collection and assign values for appropriate ui elements
foreach (var packageItem in packageItems.items)
{
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<VirtualCurrencyPackageWidget>();
widget.NameText.text = packageItem.name;
widget.DescriptionText.text = packageItem.description;
if (packageItem.price != null)
{
var realMoneyPrice = packageItem.price;
widget.PriceText.text = $"{realMoneyPrice.amount} {realMoneyPrice.currency}";
}
else if (packageItem.virtual_prices != null)
{
var virtualCurrencyPrice = packageItem.virtual_prices.First(x => x.is_default);
widget.PriceText.text = $"{virtualCurrencyPrice.name}: {virtualCurrencyPrice.amount}";
}
ImageLoader.Instance.GetImageAsync(packageItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
The following picture shows the result of the script’s work.
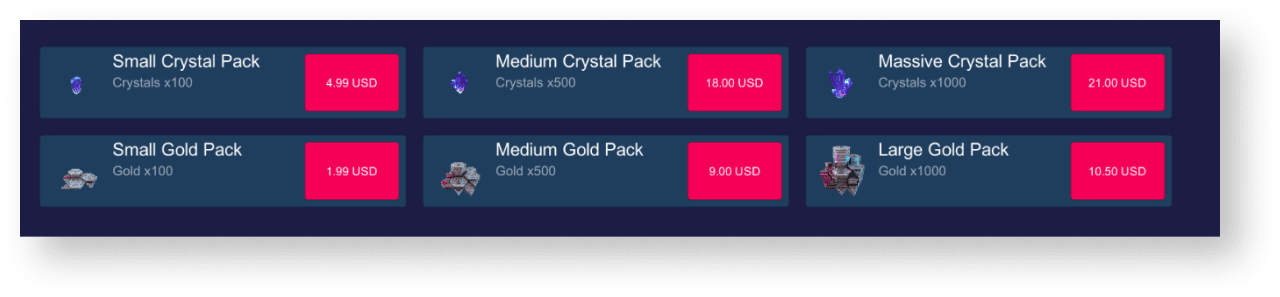
This instruction shows how to use the SDK methods to implement selling of virtual items for real currency.
Before you start, implement a display of virtual items in a catalog. In the following example, we describe how to implement purchasing of virtual items. Configuration for other item types is similar.
This tutorial describes the implementation of the following logic:
The logic and interface in the examples are less complicated than they will be in your application. A possible implementation option for selling items for real currency and displaying a catalog of items is described in the demo project.
Completar widget de artículos
Add a purchase button to the item widget and configure its visuals.
The following picture shows an example of the widget structure.
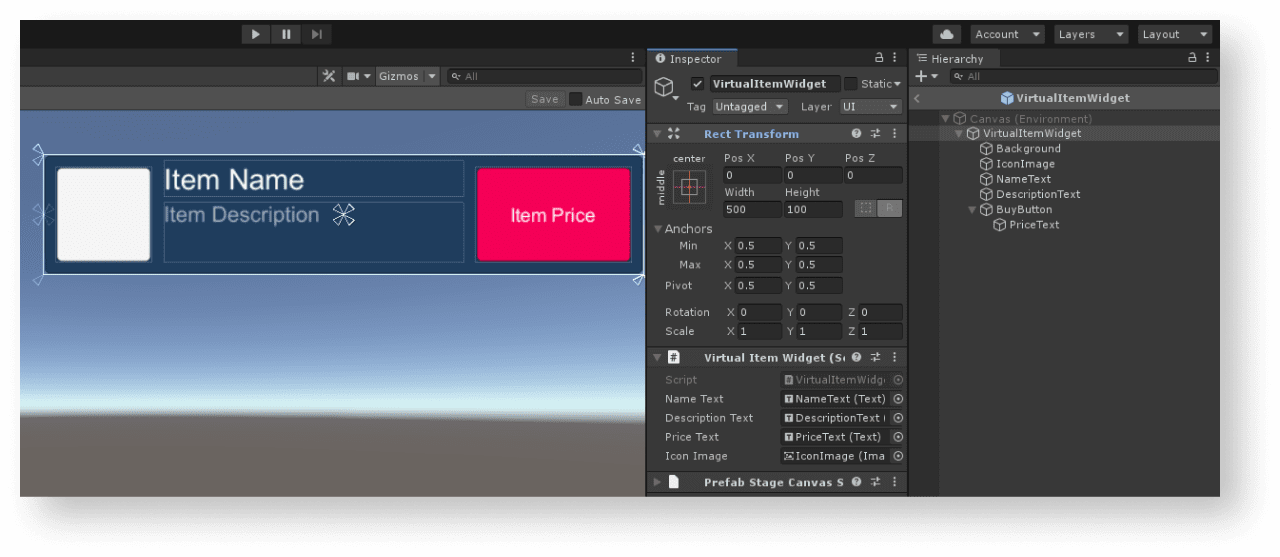
Script completo de widget de artículos
- Open the
VirtualItemWidget
script. - Declare variables for the purchase button and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualItemWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Image IconImage;
public Button BuyButton;
}
}
Controlador de página completo para mostrar la lista de artículos
- Open the
VirtualItemsPage
script. - In the
OnAuthenticationSuccess
method, pass the authorization token to theXsollaStore.Instance.Token
variable.
- A JWT received during user authorization via the
XsollaLogin.Instance.SignIn
SDK method. - Pay Station access token received during user authorization via the
XsollaLogin.Instance.GetUserAccessToken
SDK method. Use this token if you have implemented your own authorization system.
- Add logic for processing clicking on the virtual item purchase button:
- In the
OnItemsRequestSuccess
method, subscribe to the button-clicking event. - Add an anonymous method that is called after the button is clicked.
- In this method, call the
XsollaStore.Instance.ItemPurchase
SDK method to form an order and pass to it:
- In the
- a Project ID in the
projectId
parameter - an item identifier in the
itemSku
parameter - the
OnOrderCreateSuccess
method to process a successful forming of the item purchase order - the
OnError
callback method for an error
- a Project ID in the
- Implement opening of a payment page. To do this, add the
OnOrderCreateSuccess
method and call in it:- the
XsollaStore.Instance.OpenPurchaseUi
SDK method, to open a payment page - the
TrackOrderStatus
coroutine, to track changes in the order status
- the
- In the
TrackOrderStatus
coroutine, implement getting the info about the order status one time per second. To do this, use theXsollaStore.Instance.CheckOrderStatus
SDK method and pass to it:- a Project ID in the
projectId
parameter - an order number from payment details in the
orderId
parameter - an anonymous method for processing successful receiving of order status info
- an anonymous method for error processing
- a Project ID in the
- In the method for processing successful receiving of order status info, implement the callback of an
OnPurchaseSuccess
method during the payment for the order (payment statusdone
orpaid
). - In the
OnPurchaseSuccess
method, implement the processing of a successful virtual item purchase.
In the script example, we call the Debug.Log base method if the item purchase is successful. You can add other actions like inventory display, etc.
Implementation of logic for adding purchased items to the inventory isn’t required — it’s done automatically.
- If you use a built-in browser for opening a payment page, close this browser.
Example of a script for a page:
- C#
using System.Collections;
using System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualItemsPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, onSuccess: OnAuthenticationSuccess, onError: OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for catalog from store
Token.Instance = Token.Create(token);
XsollaStore.Instance.GetCatalog(XsollaSettings.StoreProjectId, OnItemsRequestSuccess, OnError, offset: 0, limit: 50);
}
private void OnItemsRequestSuccess(StoreItems storeItems)
{
// Iterating the items collection and assign values for appropriate ui elements
foreach (var storeItem in storeItems.items)
{
if (storeItem.price == null)
continue;
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<VirtualItemWidget>();
widget.NameText.text = storeItem.name;
widget.DescriptionText.text = storeItem.description;
var realMoneyPrice = storeItem.price;
widget.PriceText.text = $"{realMoneyPrice.amount} {realMoneyPrice.currency}";
ImageLoader.Instance.GetImageAsync(storeItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
widget.BuyButton.onClick.AddListener(() => { XsollaStore.Instance.ItemPurchase(XsollaSettings.StoreProjectId, storeItem.sku, OnOrderCreateSuccess, OnError); });
}
}
private void OnOrderCreateSuccess(PurchaseData purchaseData)
{
XsollaStore.Instance.OpenPurchaseUi(purchaseData);
StartCoroutine(TrackOrderStatus(purchaseData));
}
private IEnumerator TrackOrderStatus(PurchaseData purchaseData)
{
var isDone = false;
while (!isDone)
{
XsollaStore.Instance.CheckOrderStatus
(
XsollaSettings.StoreProjectId,
purchaseData.order_id,
status =>
{
if (status.status == "paid" || status.status == "done")
{
isDone = true;
OnPurchaseSuccess();
}
},
error => { OnError(error); }
);
yield return new WaitForSeconds(1f);
}
}
private void OnPurchaseSuccess()
{
UnityEngine.Debug.Log($"Purchase successful");
BrowserHelper.Instance.Close();
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
This instruction shows how to use the SDK methods to implement selling of virtual items for virtual currency.
Before you start, implement a display of virtual items in a catalog. In the following example, we describe how to implement purchasing of virtual items. Configuration for other item types is similar.
This tutorial describes the implementation of the following logic:
The logic and interface in the examples are less complicated than they will be in your application. A possible implementation option for selling items for real currency and displaying a catalog of items is described in the demo project.
Completar widget de artículos
Add a purchase button to the item widget and configure its visuals.
The following picture shows an example of the widget structure.
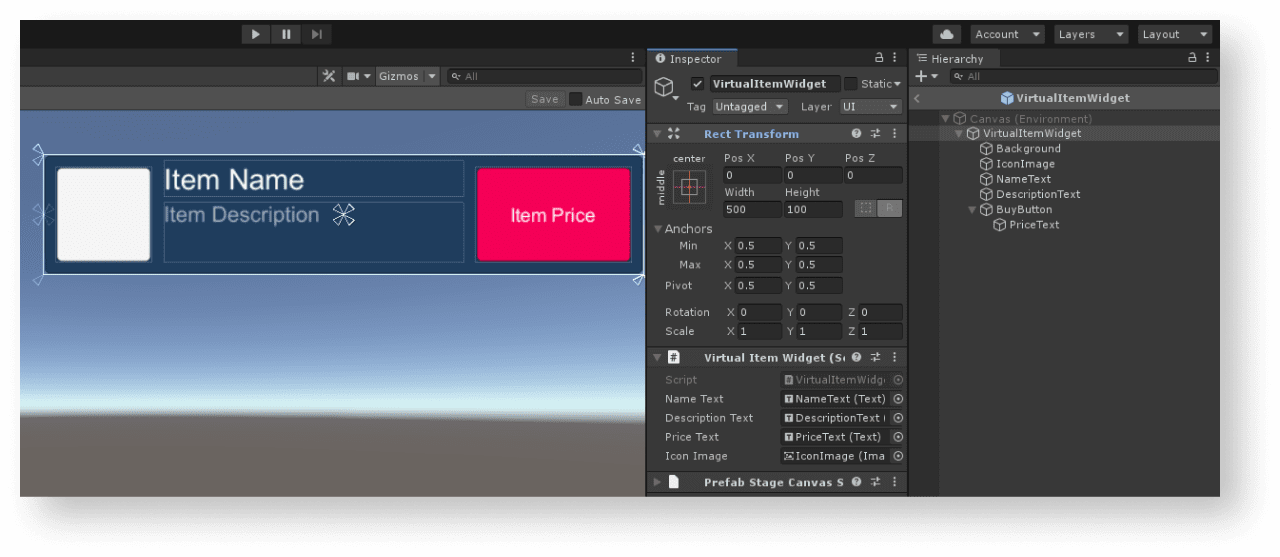
Script completo de widget de artículos
- Open the
VirtualItemWidget
script. - Declare variables for the purchase button and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualItemWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text PriceText;
public Image IconImage;
public Button BuyButton;
}
}
Controlador de página completo para mostrar la lista de artículos
- Open the
VirtualItemsPage
script. - In the
OnAuthenticationSuccess
method, pass the authorization token to theXsollaStore.Instance.Token
variable.
- A JWT received during user authorization via the
XsollaLogin.Instance.SignIn
SDK method. - Pay Station access token received during user authorization via the
XsollaLogin.Instance.GetUserAccessToken
SDK method. Use this token if you have implemented your own authorization system
- Add logic for processing clicking on the virtual item purchase button:
- In the
OnItemsRequestSuccess
method, subscribe to the button-clicking event. - Add an anonymous method that is called after the button is clicked.
- In this method, call the
XsollaStore.Instance.ItemPurchase
SDK method to form an order and pass to it:
- In the
- a Project ID in the
projectId
parameter - an item identifier in the
itemSku
parameter - the
OnOrderCreateSuccess
method to process a successful forming of the item purchase order - the
OnError
callback method for an error
- a Project ID in the
- In the
OnOrderCreateSuccess
method, implement the order status check process. To do this, use theXsollaStore.Instance.CheckOrderStatus
SDK method and pass to it:- a Project ID in the
projectId
parameter - an order number from payment details in the
orderId
parameter - an anonymous method for processing successful receiving of order status info
- an anonymous method for error processing
- a Project ID in the
- In the method for processing successful receiving of order status info, implement the callback of an
OnPurchaseSuccess
method during the payment for the order (payment statusdone
orpaid
). - In the
OnPurchaseSuccess
method, implement the processing of a successful virtual item purchase.
In the script example, we call the Debug.Log base method if the item purchase is successful. You can add other actions like inventory display, virtual currency balance change, etc.
Implementation of logic for adding purchased items to the inventory isn’t required — it’s done automatically.
Example of a script for a page:
- C#
using System.Linq;
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualItemsPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, onSuccess: OnAuthenticationSuccess, onError: OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for catalog from store
Token.Instance = Token.Create(token);
XsollaStore.Instance.GetCatalog(XsollaSettings.StoreProjectId, OnItemsRequestSuccess, OnError, offset: 0, limit: 50);
}
private void OnItemsRequestSuccess(StoreItems storeItems)
{
// Iterating the items collection and assign values for appropriate ui elements
foreach (var storeItem in storeItems.items)
{
if (storeItem.virtual_prices.Length == 0)
continue;
var widget = Instantiate(WidgetPrefab, WidgetsContainer, false).GetComponent<VirtualItemWidget>();
widget.NameText.text = storeItem.name;
widget.DescriptionText.text = storeItem.description;
var defaultPrice = storeItem.virtual_prices.First(x => x.is_default);
widget.PriceText.text = $"{defaultPrice.name}: {defaultPrice.amount}";
ImageLoader.Instance.GetImageAsync(storeItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
widget.BuyButton.onClick.AddListener(() =>
{
var price = storeItem.virtual_prices.First(x => x.is_default);
XsollaStore.Instance.ItemPurchaseForVirtualCurrency(XsollaSettings.StoreProjectId, storeItem.sku, price.sku, OnOrderCreateSuccess, OnError);
});
}
}
private void OnOrderCreateSuccess(PurchaseData purchaseData)
{
XsollaStore.Instance.CheckOrderStatus
(
XsollaSettings.StoreProjectId,
purchaseData.order_id,
status =>
{
if (status.status == "paid" || status.status == "done")
{
OnPurchaseSuccess();
}
},
error =>
{
OnError(error);
}
);
}
private void OnPurchaseSuccess()
{
UnityEngine.Debug.Log($"Purchase successful");
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
This tutorial shows how to use the SDK methods to display the balance of virtual currency in your app.
The logics and interface in the examples are less complicated than they will be in your application. A possible item catalog in an in-game store implementation option is described in the demo project.
Crear widget para la visualización del saldo
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- widget background image
- virtual currency name
- virtual currency quantity
- virtual currency image
The following picture shows an example of the widget structure.
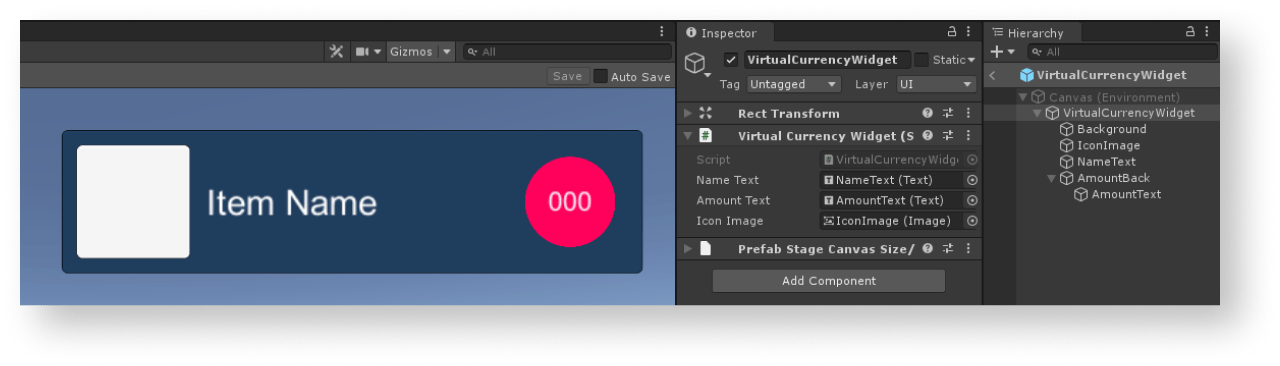
Crear un script de widget para mostrar el saldo
- Create a script
VirtualCurrencyWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class VirtualCurrencyWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text AmountText;
public Image IconImage;
}
}
Crear página con lista de monedas virtuales
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- widgets display area
The following picture shows an example of the page structure.
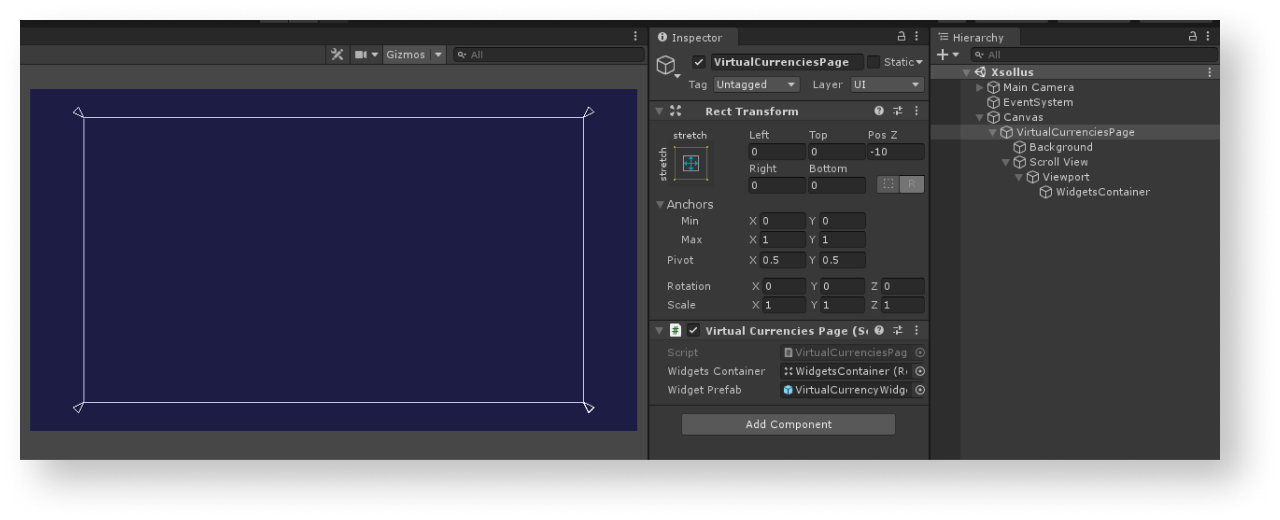
Crear controlador para la página con una lista de monedas virtuales
- Create a script
VirtualCurrenciesPage
inherited from theMonoBehaviour
base class. - Declare the following variables:
WidgetsContainer
— container for widgetsWidgetPrefab
— balance display widget prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select aVirtualCurrenciesPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel. - Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:- a username or email address in the
username
parameter - a user password in the
password
parameter
- a username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logics of getting a list of virtual currencies. To do this, in the
OnAuthenticationSuccess
method:- Pass the authorization token to the
XsollaStore.Instance.Token
variable.
- Pass the authorization token to the
- A JWT received during user authorization via the
XsollaLogin.Instance.SignIn
SDK method. - Pay Station access token received during user authorization via the
XsollaLogin.Instance.GetUserAccessToken
SDK method. Use this token if you have implemented your own authorization system.
- Call the
XsollaStore.Instance.GetVirtualCurrencyBalance
SDK method and pass to it:- the Project ID in the
projectId
parameter
- the Project ID in the
- Call the
- the
OnBalanceRequestSuccess
method for successful operation of getting a list of items - the
OnError
callback method for an error
- the
- In the
OnBalanceRequestSuccess
method, add logics for creating a widget for every received virtual currency:- Instantiate a prefab of item widget as a container child object.
- Attach the received
VirtualCurrencyWidget
component to awidget
variable.
- Pass the following data to the balance widget:
- Pass the
balanceItem.name
variable value to the element with the virtual currency name. - Pass the
balanceItem.amount.ToString()
variable value to the element with the quantity of the virtual currency. - Implement the following logics to display the item price. To show a virtual currency image, use the
ImageLoader.Instance.GetImageAsync
utility method, and pass to it:- The image URL.
- An anonymous callback function. In this function, set the received sprite as a virtual currency image.
- Pass the
Example of the page controller script:
- C#
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class VirtualCurrenciesPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, OnAuthenticationSuccess, OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for virtual currencies
Token.Instance = Token.Create(token);
XsollaStore.Instance.GetVirtualCurrencyBalance(XsollaSettings.StoreProjectId, OnBalanceRequestSuccess, OnError);
}
private void OnBalanceRequestSuccess(VirtualCurrenciesBalance balance)
{
// Iterating the virtual currencies list and assign values for appropriate ui elements
foreach (var balanceItem in balance.items)
{
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<VirtualCurrencyWidget>();
widget.NameText.text = balanceItem.name;
widget.AmountText.text = balanceItem.amount.ToString();
ImageLoader.Instance.GetImageAsync(balanceItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
The following picture shows the result of the script’s work.
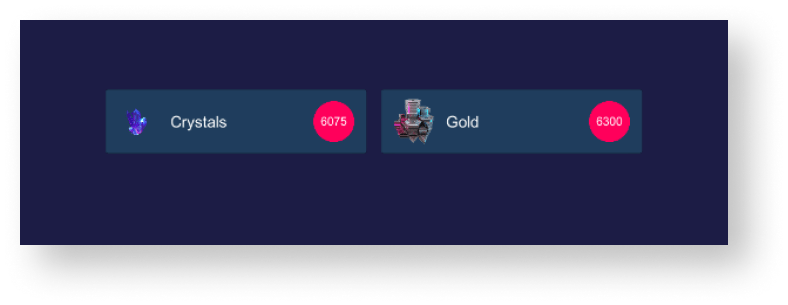
This tutorial shows how to use the SDK methods to display items in the user inventory.
The logics and interface in the examples are less complicated than they will be in your application. A possible inventory implementation option is described in the demo project.
Crear widget de artículo
- Create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Convert the created game object in a prefab by dragging a game object from a
Hierarchy panel to aProject panel. - Select a created prefab and click
Open Prefab in theInspector panel. - Add the following UI elements as prefab child objects and configure their visuals:
- item background image
- item name
- item description
- item quantity
- item image
The following picture shows an example of the widget structure.
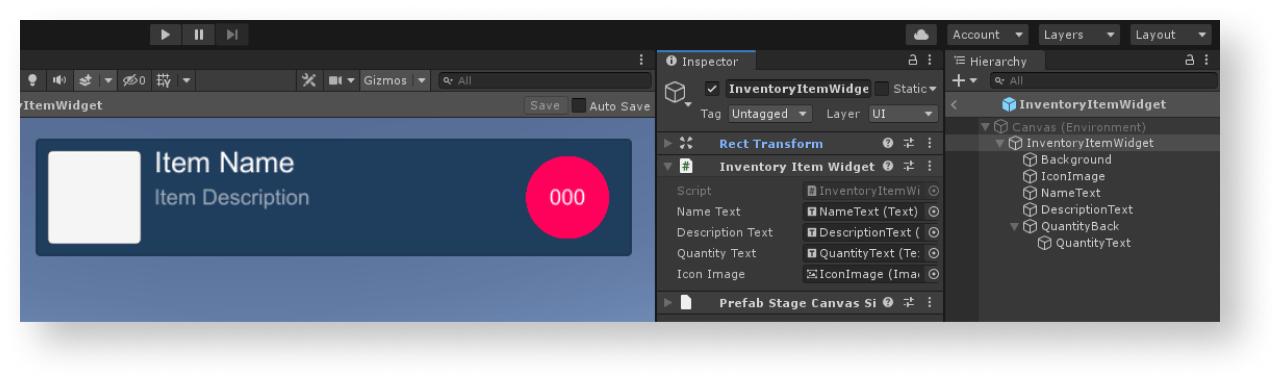
Crear script de widget de artículo
- Create a script
InventoryItemWidget
inherited from the MonoBehaviour base class. - Declare variables for the item widget interface elements and set values for them in the
Inspector panel.
Example of the widget script:
- C#
using UnityEngine;
using UnityEngine.UI;
namespace Recipes
{
public class InventoryItemWidget : MonoBehaviour
{
// Declaration of variables for UI elements
public Text NameText;
public Text DescriptionText;
public Text QuantityText;
public Image IconImage;
}
}
Crear página para mostrar el inventario
- On the scene, create an empty game object. To do this, go to the main menu and select
GameObject > Create Empty . - Add the following UI elements as prefab child objects and configure their visuals:
- page background image
- item widgets display area
The following picture shows an example of the page structure.
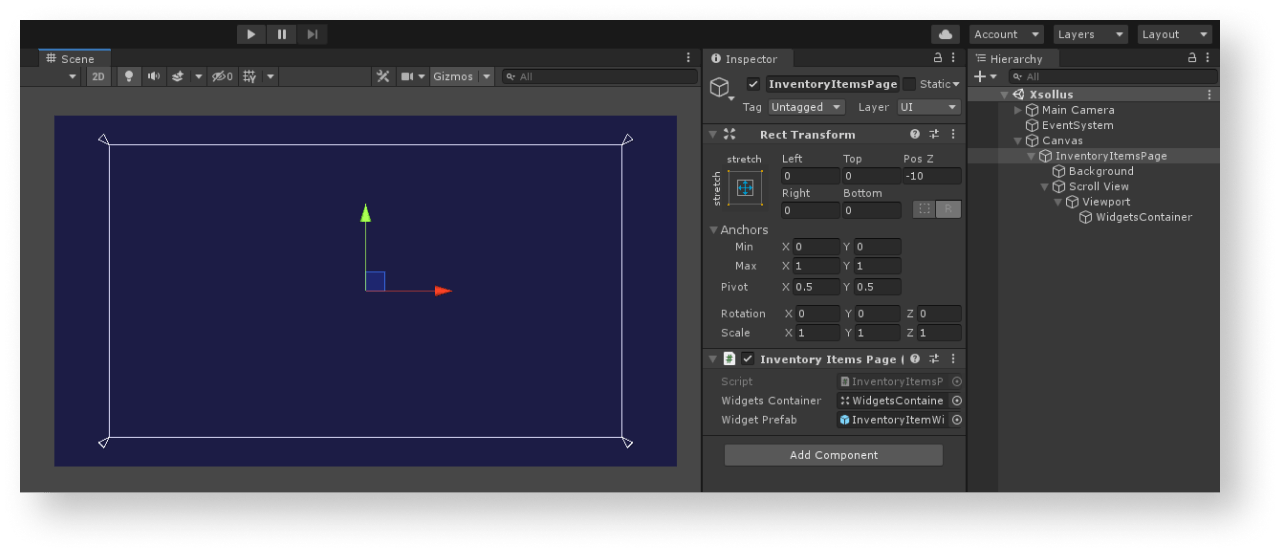
Crear controlador de página
- Create a script
InventoryItemsPage
inherited from theMonoBehaviour
base class. - Declare the following variables:
WidgetsContainer
— container for item widgetsWidgetPrefab
— item widget prefab
- Attach a script to a page game object:
- Select an object in a
Hierarchy panel. - In the
Inspector panel, clickAdd Component and select anInventoryItemsPage
script.
- Select an object in a
- Set values for variables in the
Inspector panel. - Add login logics by calling an
XsollaLogin.Instance.SignIn
SDK method in theStart
method and pass to it:- a username or email address in the
username
parameter - a user password in the
password
parameter
- a username or email address in the
xsolla
, password: xsolla
).- a flag in the
rememberUser
parameter for remembering an account - the
OnAuthenticationSuccess
callback method for successful user login - the
OnError
callback method for an error
- a flag in the
- Add logic for getting the list of items in the inventory. To do this, in the
OnAuthenticationSuccess
method:- Pass an authorization token to the
XsollaStore.Instance.Token
variable.
- Pass an authorization token to the
- A JWT received during user authorization via the
XsollaLogin.Instance.SignIn
SDK method. - Pay Station access token received during user authorization via the
XsollaLogin.Instance.GetUserAccessToken
SDK method. Use this token if you have implemented your own authorization system.
- Call the
XsollaStore.Instance.GetInventoryItems
SDK method and pass to it:- a Project ID in the
projectId
parameter
- a Project ID in the
- Call the
- the
OnItemsRequestSuccess
for successful operation of getting a list of items - the
OnError
callback method for an error
- the
- For every received item in the
OnItemsRequestSuccess
method, add logic for creating a widget:- Use the
InventoryItem.IsVirtualCurrency
method, to add a check to make sure a received item isn’t a virtual currency.
- Use the
- Instantiate a prefab of an item widget as a container child object.
- Attach the received
InventoryItemWidget
component to awidget
variable.
- Pass the following data to the item widget:
- Pass the
inventoryItem.name
variable value to the element with the item name. - Pass the
inventoryItem.description
variable value to the element with the item description. - Pass the
inventoryItem.amount.ToString()
to the element with the item quantity. - To display an item image, use the
ImageLoader.Instance.GetImageAsync
utility method and pass to it:- Image URL
- An anonymous function as a callback. In this function, add a received sprite as an item image.
- Pass the
Example of the page controller script:
- C#
using UnityEngine;
using Xsolla.Core;
using Xsolla.Login;
using Xsolla.Store;
namespace Recipes
{
public class InventoryItemsPage : MonoBehaviour
{
// Declaration of variables for containers and widget prefabs
public Transform WidgetsContainer;
public GameObject WidgetPrefab;
private void Start()
{
// Starting the authentication process
XsollaLogin.Instance.SignIn("xsolla", "xsolla", true, null, OnAuthenticationSuccess, OnError);
}
private void OnAuthenticationSuccess(string token)
{
// After successful authentication starting the request for virtual currencies
Token.Instance = Token.Create(token);
XsollaStore.Instance.GetInventoryItems(XsollaSettings.StoreProjectId, OnItemsRequestSuccess, OnError);
}
private void OnItemsRequestSuccess(InventoryItems inventoryItems)
{
// Iterating the item list and assign values for appropriate ui elements
foreach (var inventoryItem in inventoryItems.items)
{
if (inventoryItem.IsVirtualCurrency())
continue;
var widgetGo = Instantiate(WidgetPrefab, WidgetsContainer, false);
var widget = widgetGo.GetComponent<InventoryItemWidget>();
widget.NameText.text = inventoryItem.name;
widget.DescriptionText.text = inventoryItem.description;
widget.QuantityText.text = inventoryItem.quantity.ToString();
ImageLoader.Instance.GetImageAsync(inventoryItem.image_url, (url, sprite) => widget.IconImage.sprite = sprite);
}
}
private void OnError(Error error)
{
UnityEngine.Debug.LogError($"Error message: {error.errorMessage}");
}
}
}
The following picture shows the result of the script’s work.
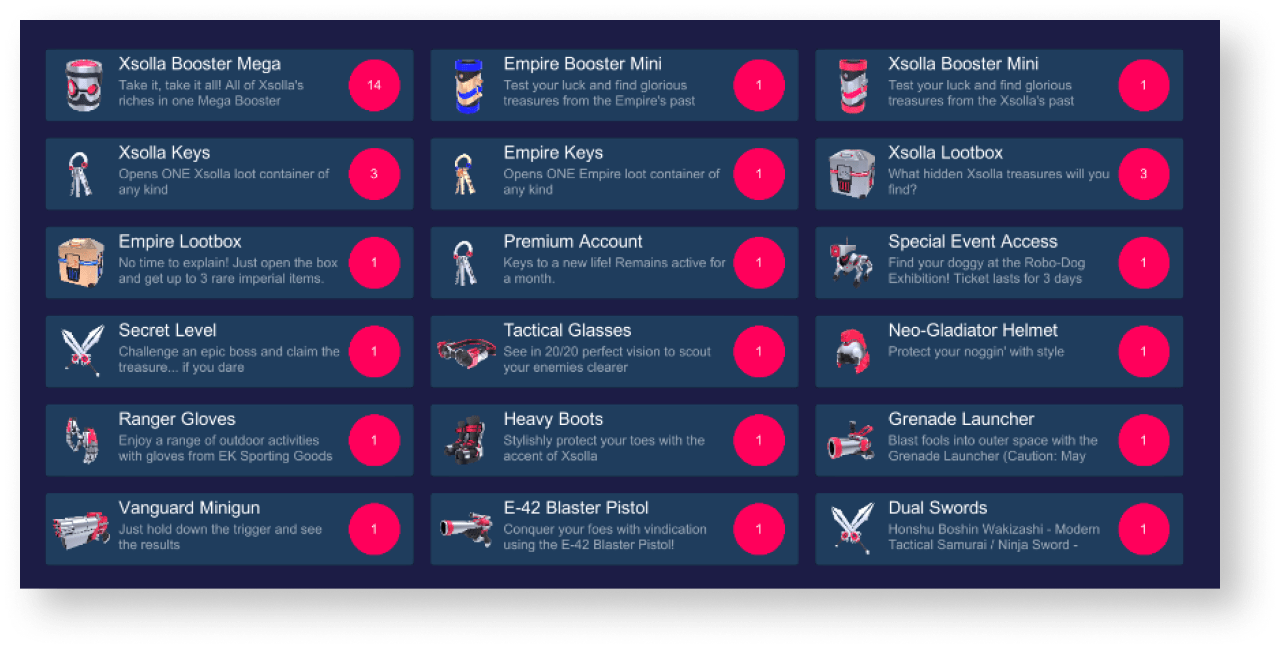
¿Has encontrado una errata u otro error de texto? Selecciona el texto y pulsa Ctrl+Intro.