Authentication
This instruction shows how to use SDK methods to implement:
- user sign-up
- resend request for a sign-up confirmation email
- user login
- user password reset
You can authenticate users with their username or email address. In the following examples we authenticate users with their username, whereas the email address is used to confirm sign-up and to reset the password.
The logics and interface in the examples are less complicated than they will be in your application. A possible authentication system implementation option is described in the demo project.
Implement user sign-up
This tutorial describes the implementation of the following logic:
Create page interface
Create a scene for a sign-up page and add the following elements on it:
- username field
- user email address field
- user password field
- sign-up button
The following picture shows the example of a page structure.
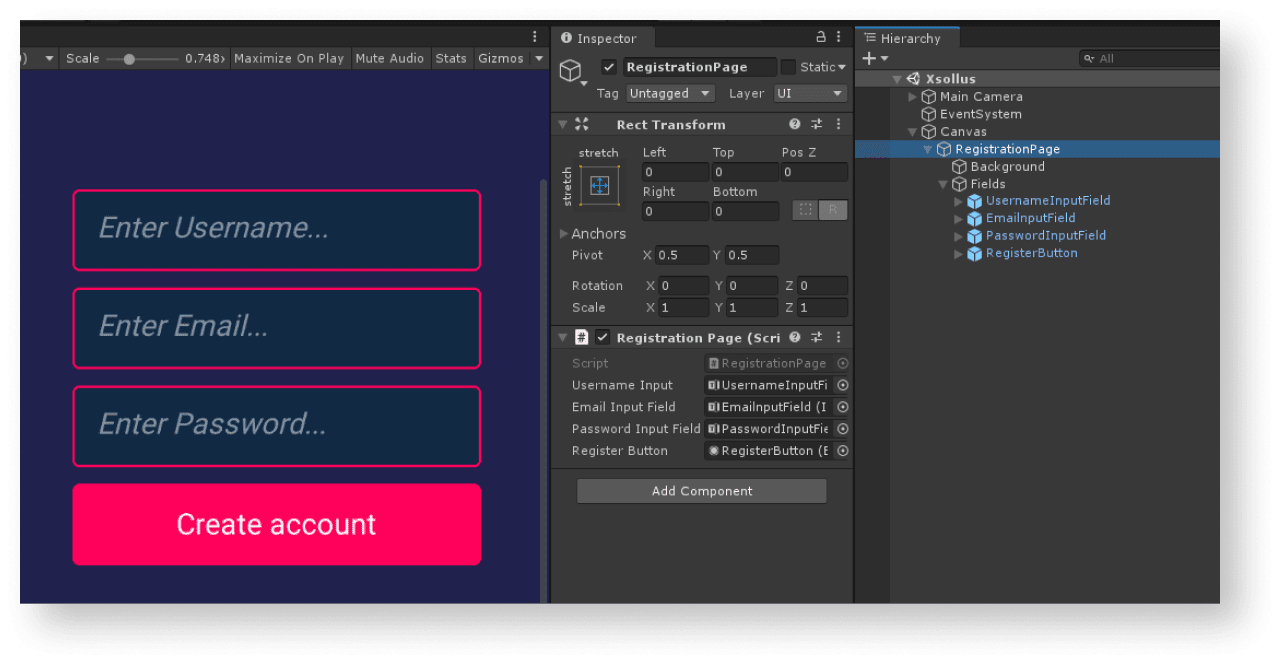
Create page controller
- Create a script
RegistrationPage
inherited from the MonoBehaviour base class. - Declare variables for the page interface elements and set values for them in the
Inspector panel. - Add logics to process clicking on the sign-up button:
- In the
Start
method, subscribe to a clicking event. - Add an anonymous method that is called after clicking the button.
- In this method, declare the
username
,email
, andpassword
variables and initialize them by the values from the fields on the page. - Call the
XsollaLogin.Instance.Registration
SDK method and pass theusername
,email
, andpassword
variables and the following methods to it:
- In the
OnSuccess
— called if sign-up is successfulOnError
— called if an error occurs
In the script’s examples, the OnSuccess
and OnError
methods call the standard Debug.Log method. The error code and description are passed in the error
parameter.
You can add other actions like opening a page with a resend request for a sign-up email or opening a login page if sign-up is successful.
Example of a script for a sign-up page:
- C#
using UnityEngine;
using UnityEngine.UI;
using Xsolla.Core;
using Xsolla.Login;
namespace Recipes
{
public class RegistrationPage : MonoBehaviour
{
// Declaration of variables for UI elements on the page
[SerializeField] private InputField UsernameInput;
[SerializeField] private InputField EmailInputField;
[SerializeField] private InputField PasswordInputField;
[SerializeField] private Button RegisterButton;
private void Start()
{
// Handling the button click
RegisterButton.onClick.AddListener(() =>
{
var username = UsernameInput.text;
var email = EmailInputField.text;
var password = PasswordInputField.text;
XsollaLogin.Instance.Registration(username, email, password, onSuccess: OnSuccess, onError: OnError);
});
}
private void OnSuccess()
{
UnityEngine.Debug.Log("Registration successful");
// Some actions
}
private void OnError(Error error)
{
UnityEngine.Debug.Log($"Registration failed. Description: {error.errorMessage}");
// Some actions
}
}
}
Set up sign-up confirmation email
After successful sign-up, a user receives a sign-up confirmation email to a specified address. You can customize emails sent to users in Publisher Account.
If you are developing an Android application, set up deep links to return a user to an application after they confirm sign-up.
Implement sign-up confirmation email resend request
This tutorial describes the implementation of the following logic:
Create page interface
Create a scene for a page with a request to resend a confirmation email and add the following elements to it:
- username/email field
- resend email button
The following picture shows an example of the page structure.
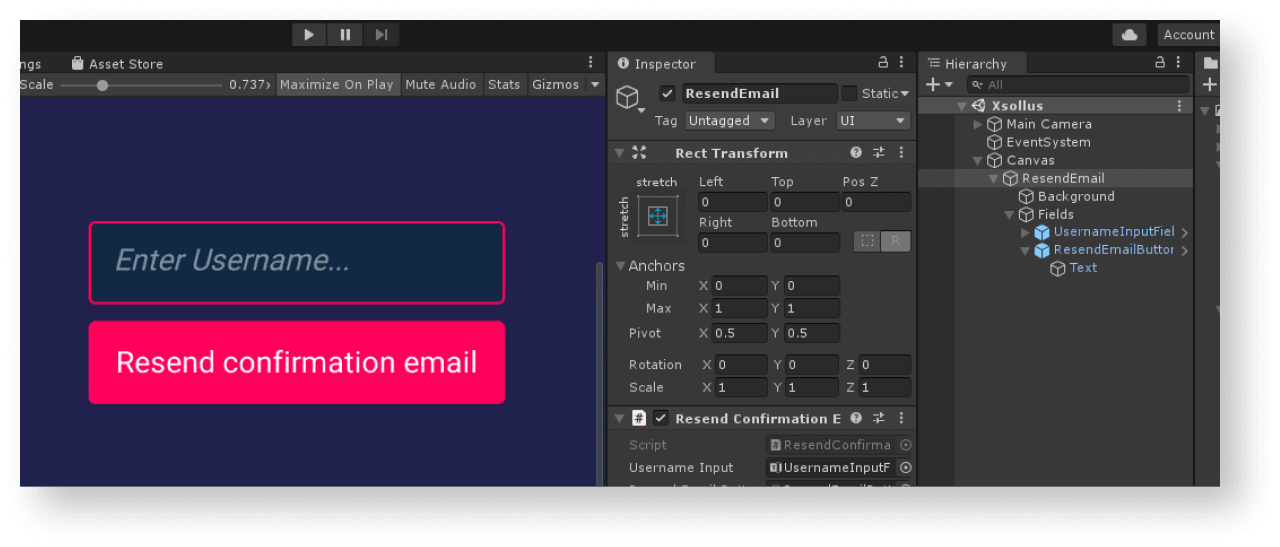
Create page controller
- Create a script
ResendConfirmationEmail
inherited from the MonoBehaviour base class. - Declare variables for page interface elements and set values for them in the
Inspector panel. - Add logics to process clicking on the resend email button:
- In the
Start
method, subscribe to a clicking event. - Add an anonymous method that is called after clicking the button.
- In this method, declare the
username
variable and initialize it by the values from the fields on the page. - Call the
XsollaLogin.Instance.ResendEmail
SDK method and pass theusername
variable andOnSuccess
andOnError
methods to it.
- In the
Example of a script for an email resend page:
- C#
using UnityEngine;
using UnityEngine.UI;
using Xsolla.Core;
using Xsolla.Login;
namespace Recipes
{
public class ResendConfirmationEmail : MonoBehaviour
{
// Declaration of variables for UI elements on the page
[SerializeField] private InputField UsernameInput;
[SerializeField] private Button ResendEmailButton;
private void Start()
{
// Handling the button click
ResendEmailButton.onClick.AddListener(() =>
{
var username = UsernameInput.text;
XsollaLogin.Instance.ResendConfirmationLink(username, onSuccess: OnSuccess, onError: OnError);
});
}
private void OnSuccess()
{
UnityEngine.Debug.Log("Resend confirmation email successful");
// Some actions
}
private void OnError(Error error)
{
UnityEngine.Debug.Log($"Resend confirmation email failed. Description: {error.errorMessage}");
// Some actions
}
}
}
If the request is successful, the user receives a sign-up confirmation email to the email address specified during sign-up.
Implement user login
This tutorial describes the implementation of the following logic:
Create page interface
Create a scene for a login page and add the following elements to it:
- username field
- password field
- remember me checkbox
- login button
The following picture shows an example of the page structure.
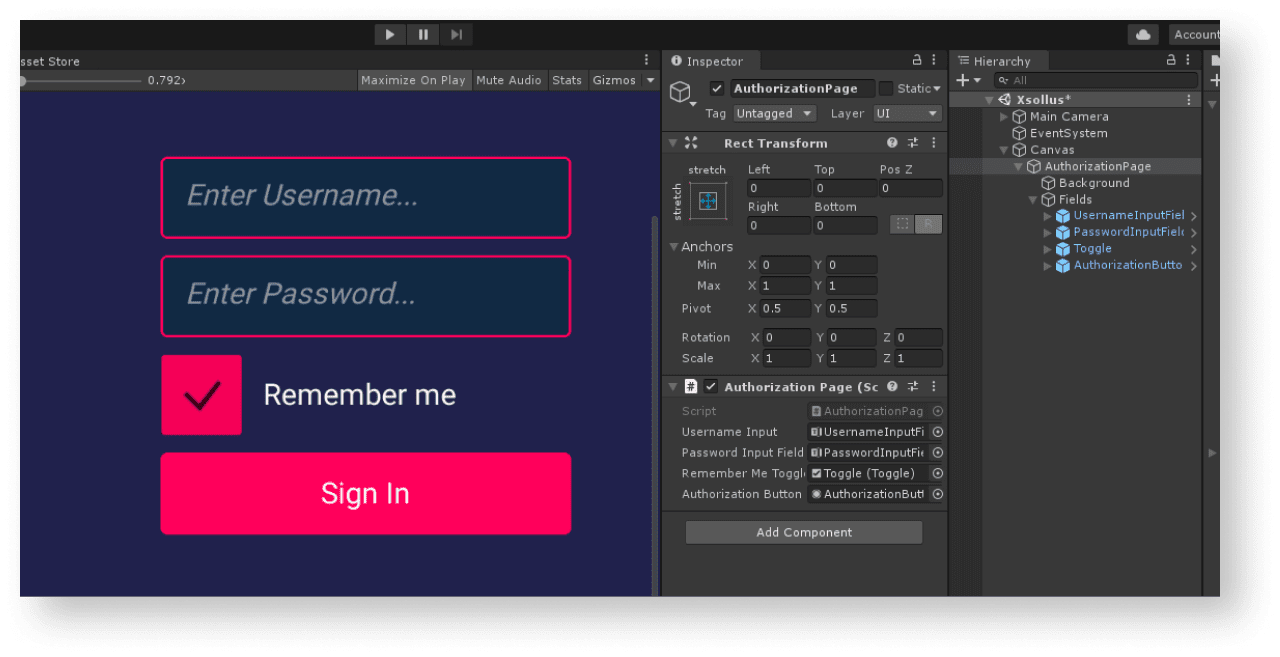
Create page controller
- Create a script
AutorizationPage
inherited from the MonoBehaviour base class. - Declare variables for page interface elements and set values for them in the
Inspector panel. - Add logics to process clicking on the login button:
- In the
Start
method, subscribe to a clicking event. - Add an anonymous method that is called after clicking the button.
- In this method, declare the
username
andpassword
variables and initialize them by the values from the fields on the page. Create arememberMe
variable and initialize it with a checkbox state to remember an account. - Call the
XsollaLogin.Instance.SignIn
SDK method and pass theusername
,password
, andrememberMe
variables andOnSuccess
andOnError
methods to it.
- In the
token
parameter. The authorization token is used in requests to Xsolla servers.Example of a script for a login page:
- C#
using UnityEngine;
using UnityEngine.UI;
using Xsolla.Core;
using Xsolla.Login;
namespace Recipes
{
public class AuthorizationPage : MonoBehaviour
{
// Declaration of variables for UI elements on the page
[SerializeField] private InputField UsernameInput;
[SerializeField] private InputField PasswordInputField;
[SerializeField] private Toggle RememberMeToggle;
[SerializeField] private Button AuthorizationButton;
private void Start()
{
// Handling the button click
AuthorizationButton.onClick.AddListener(() =>
{
var username = UsernameInput.text;
var password = PasswordInputField.text;
var rememberMe = RememberMeToggle.isOn;
XsollaLogin.Instance.SignIn(username, password, rememberMe, null, onSuccess: OnSuccess, onError: OnError);
});
}
private void OnSuccess(string token)
{
UnityEngine.Debug.Log($"Authorization successful. Token: {token}");
// Some actions
}
private void OnError(Error error)
{
UnityEngine.Debug.Log($"Authorization failed. Description: {error.errorMessage}");
// Some actions
}
}
}
Implement password reset
This tutorial describes the implementation of the following logic:
Create page interface
Create a scene for a password reset page and add the following elements to a page:
- username/email field
- password reset button
The following picture shows an example of the page structure.
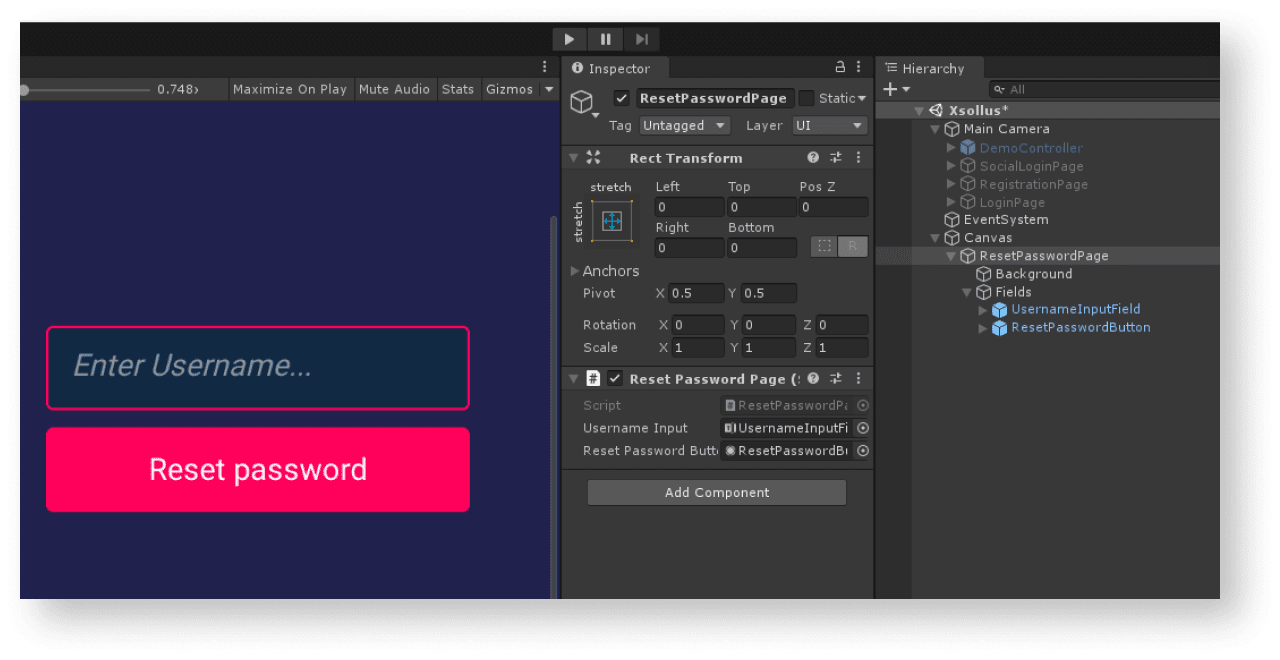
Create page controller
- Create a script
ResetPasswordPage
inherited from the MonoBehaviour base class. - Declare variables for page interface elements and set values for them in the
Inspector panel. - Add logics to process clicking on the password reset button:
- In the
Start
method, subscribe to a clicking event. - Add an anonymous method that is called after the button is clicked.
- In this method, declare the
username
variable and initialize it by the values from the fields on the page. - Call the
XsollaLogin.Instance.ResetPassword
SDK method and pass theusername
variables andOnSuccess
andOnError
methods to it.
- In the
Example of a script for a password reset page:
- C#
using UnityEngine;
using UnityEngine.UI;
using Xsolla.Core;
using Xsolla.Login;
namespace Recipes
{
public class ResetPasswordPage : MonoBehaviour
{
// Declaration of variables for UI elements on the page
[SerializeField] private InputField UsernameInput;
[SerializeField] private Button ResetPasswordButton;
private void Start()
{
// Handling the button click
ResetPasswordButton.onClick.AddListener(() =>
{
var username = UsernameInput.text;
XsollaLogin.Instance.ResetPassword(username, OnSuccess, OnError);
});
}
private void OnSuccess()
{
UnityEngine.Debug.Log("Password reset successful");
// Some actions
}
private void OnError(Error error)
{
UnityEngine.Debug.Log($"Password reset failed. Description: {error.errorMessage}");
// Some actions
}
}
}
After successful password reset request, the user receives an email with a password reset link. In Publisher Account > your Login project > General settings > URL > Callback URL, you can configure a URL address or a path a user is redirected to after successful authentication, email confirmation, or password reset.
This guide shows how you can use SDK methods to implement user sign-up and login via their social network account.
Unlike for user authentication via username/user email address and password, you don’t have to implement separate logics for user sign-up. If the user’s first login is via a social network, a new account is created automatically.
If you have implemented social login in your application as an alternative authentication method, the social network account automatically links to an existing user account if the following conditions are met:
- A user who signed up with username/email address and password logged into your application via a social network account.
- A social network returns an email address.
- User email address in a social network is the same as the email address used for sign-up in your application.
LinkSocialProvider
SDK method.This tutorial describes the implementation of the following logic:
The examples show how to set up user login via a Twitter account. You can set up all social networks in the same way.
The logics and interface in the examples are less complicated than they will be in your application. A possible authentication system implementation option is described in the demo project.
Create page interface
Create a scene for a login page and add the social login button to it. The following picture shows an example of the page structure.
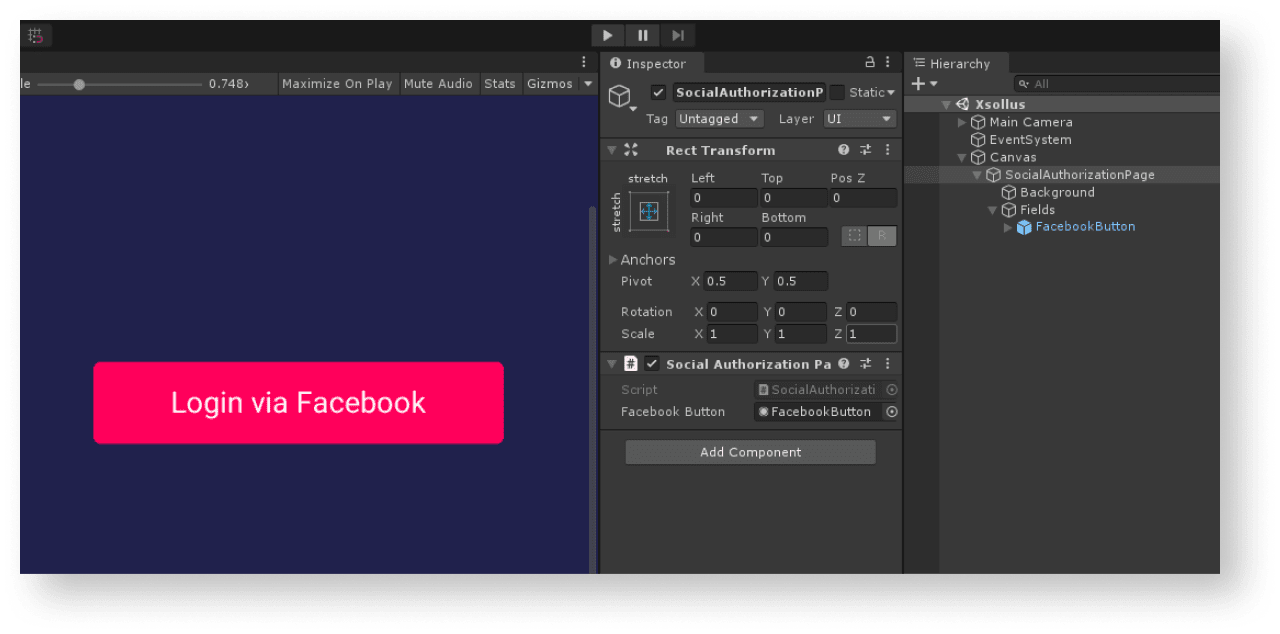
Create page controller
- Create a script
SocialAuthorizationPage
inherited from the MonoBehaviour base class. - Declare variables for the application login page interface elements and set values for them in the
Inspector panel. - Add logics to process clicking on the login button:
- In the
Start
method, subscribe to a clicking event. - Add an anonymous method that is called after clicking the button.
- To pass a login page URL, declare the
url
variable in an anonymous method. Initialize this variable by theGetSocialNetworkAuthUrl
SDK method by passing aFacebook
value in theSocialProvider
parameter. - To open a browser, call the
BrowserHelper.Instance.Open
method. To use a built-in browser, pass theurl
variable and atrue
value to the method.
- In the
- To get a token and close the browser, track the changes of the page URL after successful user sign-up:
- Declare a
singlePageBrowser
variable and initialize it via theBrowserHelper.Instance.GetLastBrowser
SDK method. - Subscribe to an active page URL changing event and set the
OnUrlChanged
method as a handler.
- Declare a
- To get a token and close the browser, track the changes of the page URL after successful user sign-up:
- Implement getting of the token:
- Use a
ParseUtils.TryGetValueFromUrl
utility method to parse a URL of an active page passed in theOnUrlChanged
method. - Add a check for an authentication code in an active page URL. The
ParseUtils.TryGetValueFromUrl
method passes an authentication code in thecode
variable. - To exchange an authentication code for a token, call the
ExchangeCodeToToken
SDK method and pass acode
variable and the following methods to it:OnSuccess
— called if sign-up is successfulOnError
— called if an error occurs
- Use a
In the script’s examples, the OnSuccess
and OnError
methods call the standard Debug.Log method. You can add other actions.
If a user successfully logs in, the authorization token is passed in the token
parameter. This token is used in requests to Xsolla servers. If an error occurs, its code and description are passed in the error
parameter.
- After you get the token, delete a game object with a browser.
Example of a script for a login page:
- C#
using UnityEngine;
using UnityEngine.UI;
using Xsolla.Core;
using Xsolla.Login;
namespace Recipes
{
public class SocialAuthorizationPage : MonoBehaviour
{
// Declaration of variables for UI elements on the page
[SerializeField] private Button FacebookButton;
private void Start()
{
// Handling the button click
FacebookButton.onClick.AddListener(() =>
{
// Opening browser
var url = XsollaLogin.Instance.GetSocialNetworkAuthUrl(SocialProvider.Facebook);
BrowserHelper.Instance.Open(url, true);
// Determining the end of authentication
BrowserHelper.Instance.InAppBrowser.AddUrlChangeHandler(OnUrlChanged);
});
}
// Getting token
private void OnUrlChanged(string url)
{
if (ParseUtils.TryGetValueFromUrl(url, ParseParameter.code, out var code))
{
XsollaLogin.Instance.ExchangeCodeToToken(code, OnSuccess, OnError);
Destroy(BrowserHelper.Instance.gameObject);
}
}
private void OnSuccess(string token)
{
UnityEngine.Debug.Log($"Authorization successful. Token: {token}");
// Some actions
}
private void OnError(Error error)
{
UnityEngine.Debug.Log($"Authorization failed. Description: {error.errorMessage}");
// Some actions
}
}
}
Found a typo or other text error? Select the text and press Ctrl+Enter.