Set up selling game keys
You can sell game keys via a direct link, store UI, or widget.
Item | Selling method |
---|---|
One copy of a game (game key). | Direct link, widget, or store interface. When selling through the store interface, use the quick purchase method without creating a cart. |
Several copies of the game (game keys) or several games in a cart. | Store interface. Use the Site Builder or integrate the In-Game Store API. |
You can sell game keys to authorized and unauthorized users.
Authorization allows you to:
- set limits on the sale of game keys for user
- personalize item catalogs and promotional offers
- use the entitlement system
- store user data in the Xsolla payment interface
You can authorize users using the Login product or your own authorization system. Detailed setup information is provided in these instructions.
Access to the game is granted automatically after purchasing the game key. However, game platforms may set their own activation key rules.
You can limit the display time of the game key package in the catalog, e.g., during seasonal sales. To do this, pass the start and end date of the availability period per ISO 8601 in the periods
object of the corresponding API call:
To limit the number of game keys available for purchase by the user, follow the instructions.
Selling via direct link
The following link opens the payment UI:
- curl
https://purchase.xsolla.com/pages/buy?type={YOUR-ITEM-TYPE}&project_id={YOUR_PROJECT_ID}&sku={YOUR-ITEM-SKU}
Buying game keys via a direct link for real currency is possible only after signing a license agreement with Xsolla. To do this, in Publisher Account, go to the Agreements & Taxes > Agreements section, complete the agreement form, and wait for the confirmation. It may take up to 3 business days to review the agreement.
To test payments, you can use the test environment by adding the mode=sandbox
parameter to the link.
Add the following data to this link:
YOUR-ITEM-TYPE
— item type:game
— game;game_key
— game on a specific platform.bundle
— bundle.
YOUR-PROJECT-ID
— ID of your project from the Project settings > General settings > Project ID section in Publisher Account.YOUR-ITEM-SKU
— game key package SKU. To sell a game on a specific platform, use the Get games list (usually this SKU looks likeunit_name_drm_sku
) to get the SKU.
- Payment UI style: theme (dark or the default light theme), size, and other parameters. Specify the
ui_settings
parameters in the URL and pass asettings.ui
JSON-object with Base64 encoding as the value. Example of the URL with UI settings:
- curl
https://purchase.xsolla.com/pages/buy?type={YOUR-ITEM-TYPE}&project_id={YOUR_PROJECT_ID}&sku={YOUR-ITEM-SKU}&ui_settings=ewoJCQkic2l6ZSI6ICJzbWFsbCIsCgkJCSJ0aGVtZSI6ICJkYXJrIgoJCX0=
- Token for passing user data. Used when selling game keys to authenticated users only. This token depends on the authentication method. Example of the URL with a token:
- curl
https://purchase.xsolla.com/pages/buy?type={YOUR-ITEM-TYPE}&project_id={YOUR_PROJECT_ID}&sku={YOUR_ITEM_SKU}&xsolla_login_token={ACCESS_TOKEN}
- The
mode=sandbox
parameter for payment tests. You can use test bank cards to complete payments.
- Example of the URL for testing:
- curl
https://purchase.xsolla.com/pages/buy?type={YOUR-ITEM-TYPE}&project_id={YOUR_PROJECT_ID}&sku={YOUR-ITEM-SKU}&mode=sandbox
Selling via store UI
You can sell game keys through the store interface. To create a store, you can:
- use Site Builder
- create your own version of the store and integrate the In-Game Store API
To sell game keys packages using the In-Game Store API:
- To display a catalog, use the Get games list method.
Implement the purchase of game keys:
- For a quick purchase of one key, use the Create order with specified item method. In response to this method, you will receive a token that must be used to open the payment interface.
- To purchase several game keys, use the cart management methods:
- Update cart item from current cart to add a game key to the cart.
- Get current user’s cart to get a list of game keys in the cart.
- Create an order with all items from the current cart to pay for game keys in the cart. In response to this method, you will receive a token that must be used to open the payment interface.
items.unit_items.sku
parameter from the request to get the list of games.Selling via widget
You can add a widget to your page to sell game keys and customize it. To copy the widget code, go to the Widget customization section after creating the keys package in your Publisher Account.
If a game is sold on a single platform, the widget will display the game price specific to that platform.
Example: Buy now for $10.
If a game is sold on multiple platforms, the widget will display the lowest price among the platforms.
Example: Get from $10.
In the order creation window, the user can see prices for all platforms and make a choice.
You can also display the price for a specific platform in the widget by specifying the platform SKU in the drm parameter.
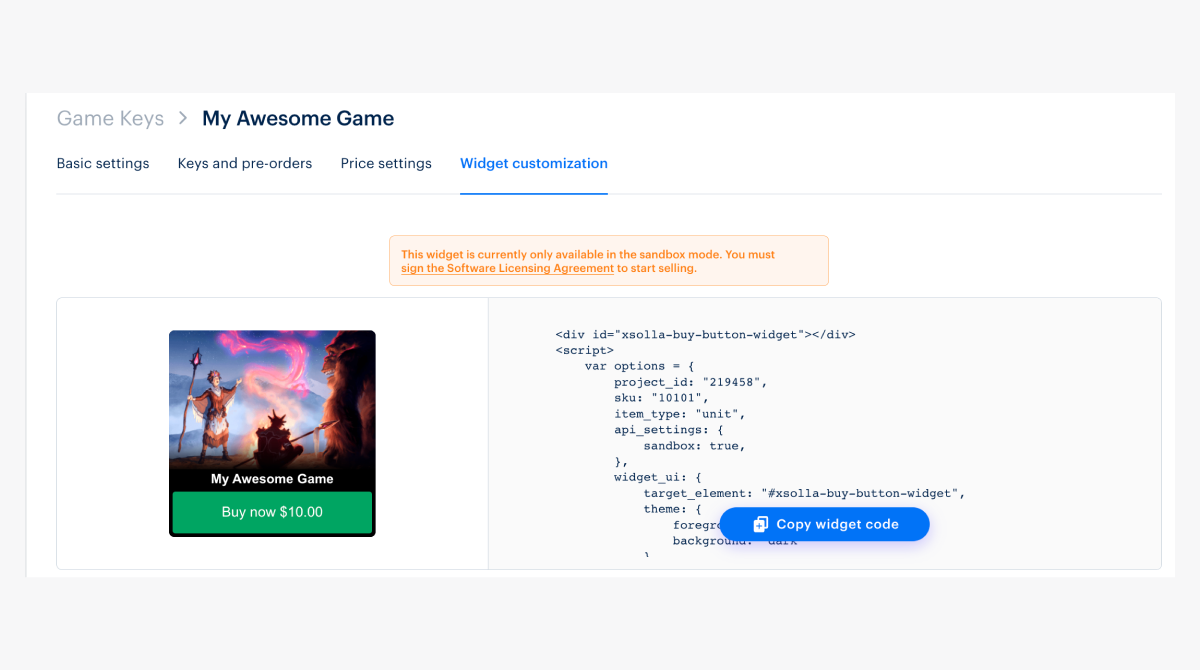
Widget code example:
<div id="xsolla-buy-button-widget"></div>
<script>
var options = {
project_id: "101010",
sku: "my_key",
user: {
auth: "9qs9VyCIQQXBlzJQcfETIKWZDvhi4Sz1"
},
drm: "steam",
item_type: "unit",
api_settings: {
sandbox: true,
},
widget_ui: {
target_element: "#xsolla-buy-button-widget",
theme: {
foreground: "green",
background: "light"
},
},
payment_widget_ui: {
lightbox: {
height: '700px',
spinner: 'round'
}
}
};
var s = document.createElement('script');
s.type = "text/javascript";
s.async = true;
s.src = "https://cdn.xsolla.net/embed/buy-button/3.1.8/widget.min.js";
s.addEventListener('load', function (e) {
var widgetInstance = XBuyButtonWidget.create(options);
}, false);
var head = document.getElementsByTagName('head')[0];
head.appendChild(s);
</script>
<style>
#xsolla-buy-button-widget {
/* place code for button positioning here */
margin: 10px
}
/* Styles the button itself */
.x-buy-button-widget.x-buy-button-widget__tiny
.x-buy-button-widget-payment-button {
background-color: #ff005b;
color: black;
}
</style>
Widget parameters
Parameter | Type | Description |
---|---|---|
project_id | integer | ID of the project in which game keys or bundles with game keys, in-game items or bundles with items, are uploaded. |
item_type | string | Item type. Can take values of virtual_good , virtual_currency , game_key , unit . The unit type is used when there are multiple platforms for distributing the game. |
sku | string | Unique item ID. |
drm | string | Distribution platform SKU, for example, steam . Allows displaying the price for a specific platform. |
api_settings | object | Environment and host configuration settings:
|
user | object | An object with user data. |
user.auth | string | User authorization token: JSON Web Token or Pay Station access token. |
user.locale | string | User locale. Determines the language of the button text and payment interface. It is used a two-letter language code based on ISO_639-1. |
widget_ui.theme | object | The color theme of the widget, determining its appearance. It can take values {foreground:[‘blue’,‘red’,‘green’,‘gold’], background:[’light’,‘dark’]} |
widget_ui.template | string | Template. Possible values:
|
widget_ui.target_element | string | Element of the page, where the widget should be rendered (jQuery selector should be used, for example #widget-example ). Required |
Parameters that determine the appearance of the payment interface
Parameter | Type | Description |
---|---|---|
payment_ui | object | Payment interface appearance parameters. |
payment_widget_ui | object | An object with parameters that determine the appearance of the payment interface. |
payment_widget_ui.lightbox | object | An object with options for the modal window in which the payment interface is opened. |
payment_widget_ui.lightbox.width | string | Lightbox frame width. If null , depends on Pay Station width. Default is null . |
payment_widget_ui.lightbox.height | string | Lightbox frame height. If null , depends on Pay Station height. Default is 100% . |
payment_widget_ui.lightbox.zIndex | integer | Defines arrangement order. Default is 1000 . |
payment_widget_ui.lightbox.overlayOpacity | integer | Opacity of the widget’s background (0 — completely transparent, 1 — completely opaque). The default value is 60% (.6 ). |
payment_widget_ui.lightbox.overlayBackground | string | Overlay background color. Default is #000000 . |
payment_widget_ui.lightbox.contentBackground | string | Frame background color. Default is #ffffff . Note that these color changes do not affect the Pay Station iframe itself, only the settings of the lightbox that hold it. |
payment_widget_ui.lightbox.spinner | string | Type of animated loading indicator. Can be xsolla or round . Default is xsolla . |
payment_widget_ui.lightbox.spinnerColor | string | Spinner color. No default value. |
payment_widget_ui.childWindow | object | Settings for the child window in which the payment interface is opened. Works for the mobile version. |
payment_widget_ui.childWindow.target | string | The property that determines where the child window should be opened. It can take values of _blank , _self , _parent . The default value is — _blank . |
Widget methods
var widgetInstance = XBuyButtonWidget.create(options)
— create the widget instance and render it on the page.widgetInstance.on(event, handler)
— attaches an event handler function for the event to the widget.event (string)
— event type.handler (function)
— a function to execute when the event is triggered.
widgetInstance.off(event, handler)
— removes an event handler.event (string)
— event type.handler (function)
— a handler function previously attached for the event.
List of events:
Parameter | Description |
---|---|
init | Widget initialized. |
open | Widget opened. |
load | Payment UI (Pay Station) loaded. |
close | Payment UI (Pay Station) closed. |
status | User is on the status page. |
status-invoice | User is on the status page; payment in progress. |
status-delivering | Event when the user was moved on the status page, payment was completed, and we’re sending payment notification. |
status-done | User is on the status page; payment credited to the user’s account. |
status-troubled | Event when the user was moved on the status page, but the payment failed. |
You can access the list of events using XBuyButtonWidget.eventTypes
object.
Button customization
- Open your project in Publisher Account.
- In the side menu, click Store.
- In the Game Keys pane, click Configure.
- Select a game key and go to the Widget customization tab.
- In the Customize block, select the background color.
theme
object in code so that the parameter background
has an empty string as a value.- When you add the widget code to your page, it includes inherited styles. Add the styles below to override these styles.
style
tag below the script
tag that you got from the Widget customization tab for CSS inheritance/priority reasons.- css
/* This should be used for button positioning but note this technically repositions the entire widget */
#xsolla-buy-button-widget {
/* place code for button positioning here */
}
/* Styles the button itself */
.x-buy-button-widget.x-buy-button-widget__tiny
.x-buy-button-widget-payment-button {
background-color: #ff005b;
color: black;
}
/* Button on hover */
.x-buy-button-widget.x-buy-button-widget__tiny
.x-buy-button-widget-payment-button:hover {
background-color: #ff005b;
}
/* The following are style overrides to leave you with just the button */
/* space immediately surrounding button */
.x-buy-button-widget-button-block.x-buy-button-widget-button-block__light {
background-color: white;
}
/* space above button (including game title area) */
.x-buy-button-widget.x-buy-button-widget__tiny
.x-buy-button-widget-game-logo {
height: 200px;
background-image: none !important;
background-color: white;
}
/* Game title (located right above button) */
.x-buy-button-widget-game-name.x-buy-button-widget-game-name__light {
display: none !important;
}
- The id/class names above and the code snippet are used in conjunction with the copied widget code (the image at step 5). For this reason, it is important that you implement the copied widget code.
- You can use the code above as is or modify the code based on your scenario. The code comments (lines 1, 3, 5, 11, 16, 18, 22, 29) are there to help determine what each CSS rule targets and assist in future styling. For example, if you want just the button (and not the entire widget), you’ll need to substitute the background color of your page in the places below where the color is
white
— lines 20 and 27.
How to create multiple buttons or SKUs
You can refer to Xsolla Pay2Play Widget Simple Integration 4 buttons for a code example on how this is accomplished using the Pay2Play widget.
The structure is similar to the Buy Button widget code. An example of a migration:
- javascript
<div id="xsolla-buy-button-widget"></div>
<div id="xsolla-buy-button-widget-2"></div>
<script>
var options = {
project_id: "191204",
sku: "789",
item_type: "unit",
api_settings: {
sandbox: false,
},
widget_ui: {
target_element: "#xsolla-buy-button-widget",
theme: {
foreground: "gold",
background: "",
},
},
payment_widget_ui: {
lightbox: {
height: "700px",
spinner: "round",
},
},
};
// options for second buy button - note the different SKU and target_element
var options2 = {
project_id: "191204",
sku: "123",
item_type: "unit",
api_settings: {
sandbox: false,
},
widget_ui: {
target_element: "#xsolla-buy-button-widget-2",
theme: {
foreground: "gold",
background: "",
},
},
payment_widget_ui: {
lightbox: {
height: "700px",
spinner: "round",
},
},
};
var s = document.createElement("script");
s.type = "text/javascript";
s.async = true;
s.src = "https://cdn.xsolla.net/embed/buy-button/3.1.4/widget.min.js";
// one event listener per widget instance. repeat for more buttons, passing in different SKUs
s.addEventListener(
"load",
function (e) {
var widgetInstance = XBuyButtonWidget.create(options);
},
false
);
s.addEventListener(
"load",
function (e) {
var widgetInstance2 = XBuyButtonWidget.create(options2);
},
false
);
var head = document.getElementsByTagName("head")[0];
head.appendChild(s);
</script>
- Lines 1 and 2 — one
div
per button, each with a unique ID. - Starting on line 26 are the options for the second button (laid out in the
options2
object). You will need a set ofoptions
like the ones above for each Buy button. Note the differentsku
(line28) andtarget_element
(line 34, targeting thediv
on line 2).
Found a typo or other text error? Select the text and press Ctrl+Enter.