Create catalog UI
There are three ways to create catalog UI:
Using own interface
When creating a directory in your own interface, you can use:
- Own data storage and any authorization option. In this case, implement the catalog UI on your side.
- Your own authorization and the Get Plans server-side method. Implement the catalog UI on your side after getting the list of plans.
- Xsolla Login and client-side API calls.
Xsolla Login and client-side API calls
To implement a catalog:- Get a list of subscription plans using client methods:
- if your project has subscription-based products configured, use the client-side method to get the subscription plans by products
- if your project does not have subscription-based products configured, the client-side method for getting the list of plans
- Implement the display of the received list of plans in the interface.
Client-side method to get the subscription plans by products
On the client side of your application, use an HTTP get request to implement getting the list of plans: https://subscriptions.xsolla.com/api/user/v1/projects/{project_id}/products/{productId}/plans
.
The request must contain an Authorization: Bearer <client_user_jwt>
header, where <client_user_jwt>
is user’s JSON Web Token (JWT) — a unique, Base64-encoded token encoded according to the Base64 standard. To get the token:
- Use the
Register new user andAuth by username and password API calls if your application uses login and password authorization. Use the
Auth via social network API call if your application uses authorization through social networks.
projectId
— project ID. You can find this parameter in your Publisher Account next to the name of the project.
productID
— subscription-based product ID. To get it, contact your Customer Success Manager or email to csm@xsolla.com.
Parameter | Type | Description |
---|---|---|
plan_id | array of integers | Plan ID. |
| array of strings | Plan external ID. Can be found in the Publisher Account in the Subscriptions > Subscription plans > your plan section or via the Get Plans API call. |
| integer | Limit for the number of elements on the page. 15 items are displayed by default. |
| integer | Number of the element from which the list is generated. The count starts from 0 by default. |
| string | Interface language in two-letter lowercase. Accepts ISO 639-1 values. If this parameter is not passed, the language is determined by the user’s IP address. Available values:
|
| string | The two-letter ISO 3166-1 alpha-2 designation is used to identify the user’s country. This parameter affects the choice of locale and currency. If this parameter is not passed, the country is determined by the user’s IP address. |
- curl
curl -X 'GET' \
'https://subscriptions.xsolla.com/api/user/v1/projects/{project_id}/products/{productId}/plans?country=RU ' \
-H 'accept: application/json' \
-H 'Authorization: Bearer client_user_jwt'
- javascript
{
"items": [
{
"plan_id": 54321,
"plan_external_id": "PlanExternalId",
"plan_group_id": "TestGroupId",
"plan_type": "all",
"plan_name": "Localized plan name",
"plan_description": "Localized plan description",
"plan_start_date": "2021-04-11T13:51:02+03:00",
"plan_end_date": "2031-04-11T13:51:02+03:00",
"trial_period": 7,
"period": {
"value": 1,
"unit": "month"
},
"charge": {
"amount": 4.99,
"setup_fee": 0.99,
"currency": "USD"
},
"promotion": {
"promotion_charge_amount": 3.99,
"promotion_remaining_charges": 3
}
}
],
"has_more": false
}
Client-side method for getting the list of plans
On the client side of your application, use an HTTP GET request to implement getting the list of plans: https://subscriptions.xsolla.com/api/user/v1/projects/{project_id}/plans
.
The request must contain an Authorization: Bearer <client_user_jwt>
header, where <client_user_jwt>
is user’s JSON Web Token (JWT) — a unique, Base64-encoded token encoded according to the Base64 standard. To get the token:
- Use the
Register new user andAuth by username and password API calls if your application uses login and password authorization. Use the
Auth via social network API call if your application uses authorization through social networks.
projectId
path parameter. You can find this parameter in your Publisher Account next to the name of the project.Specify as query parameters:
Parameter | Type | Description |
---|---|---|
plan_id | array of integers | Plan ID. |
| array of strings | Plan external ID. Can be found in the Publisher Account in the Subscriptions > Subscription plans > your plan section or via the Get Plans API call. |
| integer | Limit for the number of elements on the page. 15 items are displayed by default. |
| integer | Number of the element from which the list is generated. The count starts from 0 by default. |
| string | Interface language in two-letter lowercase. Accepts ISO 639-1 values. If this parameter is not passed, the language is determined by the user’s IP address. Available values:
|
| string | The two-letter ISO 3166-1 alpha-2 designation is used to identify the user’s country. This parameter affects the choice of locale and currency. If this parameter is not passed, the country is determined by the user’s IP address. |
- curl
curl -X 'GET' \
'https://subscriptions.xsolla.com/api/user/v1/projects/{project_id}/plans?country=RU ' \
-H 'accept: application/json' \
-H 'Authorization: Bearer client_user_jwt'
- javascript
{
"items": [
{
"plan_id": 54321,
"plan_external_id": "PlanExternalId",
"plan_group_id": "TestGroupId",
"plan_type": "all",
"plan_name": "Localized plan name",
"plan_description": "Localized plan description",
"plan_start_date": "2021-04-11T13:51:02+03:00",
"plan_end_date": "2031-04-11T13:51:02+03:00",
"trial_period": 7,
"period": {
"value": 1,
"unit": "month"
},
"charge": {
"amount": 4.99,
"setup_fee": 0.99,
"currency": "USD"
},
"promotion": {
"promotion_charge_amount": 3.99,
"promotion_remaining_charges": 3
}
}
],
"has_more": false
}
Using Xsolla Pay Station
- Implement getting the token via the server-side Create token API call. Pass the following parameters in the request:
user.id
— user ID in your authorization system.user.email
— user email. Must be valid according to the RFC 822 protocol.settings.project_id
— project ID. You can find this parameter in your Publisher Account next to the name of the project.
- Implement opening the payment UI in one of the following ways:
- javascript
{
"user": {
"name": {
"value": "j.smith@email.com"
},
"id": {
"value": "123a345b678c091d"
}
},
"settings": {
"project_id": 177226
}
}
An example of displaying the subscription catalog in the Xsolla Pay Station:
Using Xsolla Site Builder
Xsolla Site Builder allows you to create and set up your site for subscription sales. To do this, use the Web Shop template to create a site. You can read more about setting up roles in the Web Shop with user authentication instructions.
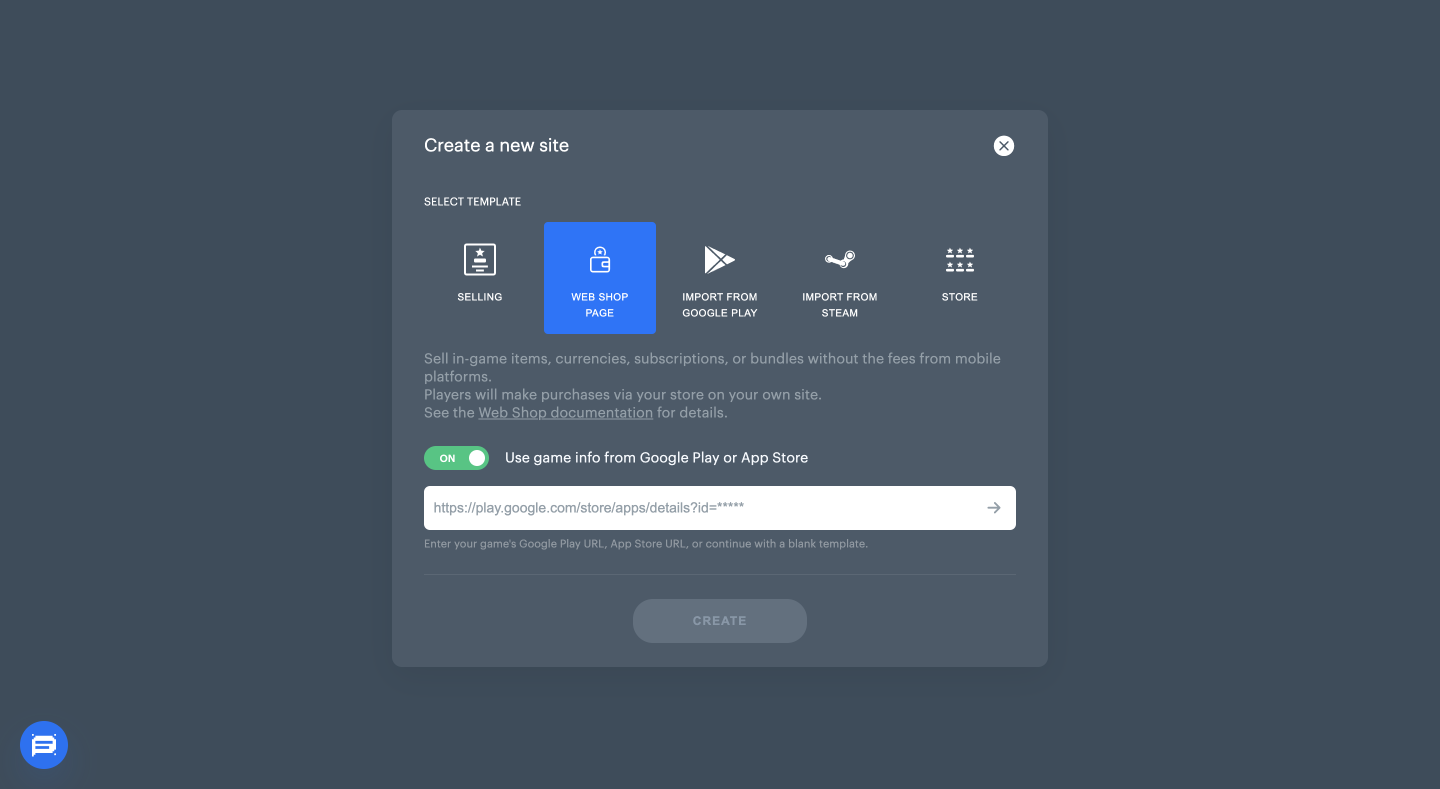
Found a typo or other text error? Select the text and press Ctrl+Enter.