アプリケーション側でSDKを統合する
- ログインシステム、インゲームストアなど、アプリケーションのページのインターフェイスをデザインします。
- SDKメソッドを使って、ユーザー認証、ストア表示、購入などのロジックをアプリケーションに実装できます。
<xsollaExtention>/assets/scripts/samples
ディレクトリにあります。ユーザー名/メールアドレスとパスワードによるユーザーの新規登録とログイン
この説明では、SDKのメソッドを使用して実装する方法を示します:
ユーザーの認証は、ユーザー名またはメールアドレスで行うことができます。以下の例では、ユーザー名でユーザーを認証し、メールアドレスは登録の確認やパスワードのリセットに使用します。
ユーザー登録を実装する
このチュートリアルでは、以下のロジックの実装について説明します:ページのインターフェースを作成する
新規登録ページのシーンを作り、そこに以下の要素を追加します:
- ユーザー名フィールド
- ユーザーEメールアドレスフィールド
- ユーザーパスワードフィールド
- 新規登録ボタン
ページ構造の例:
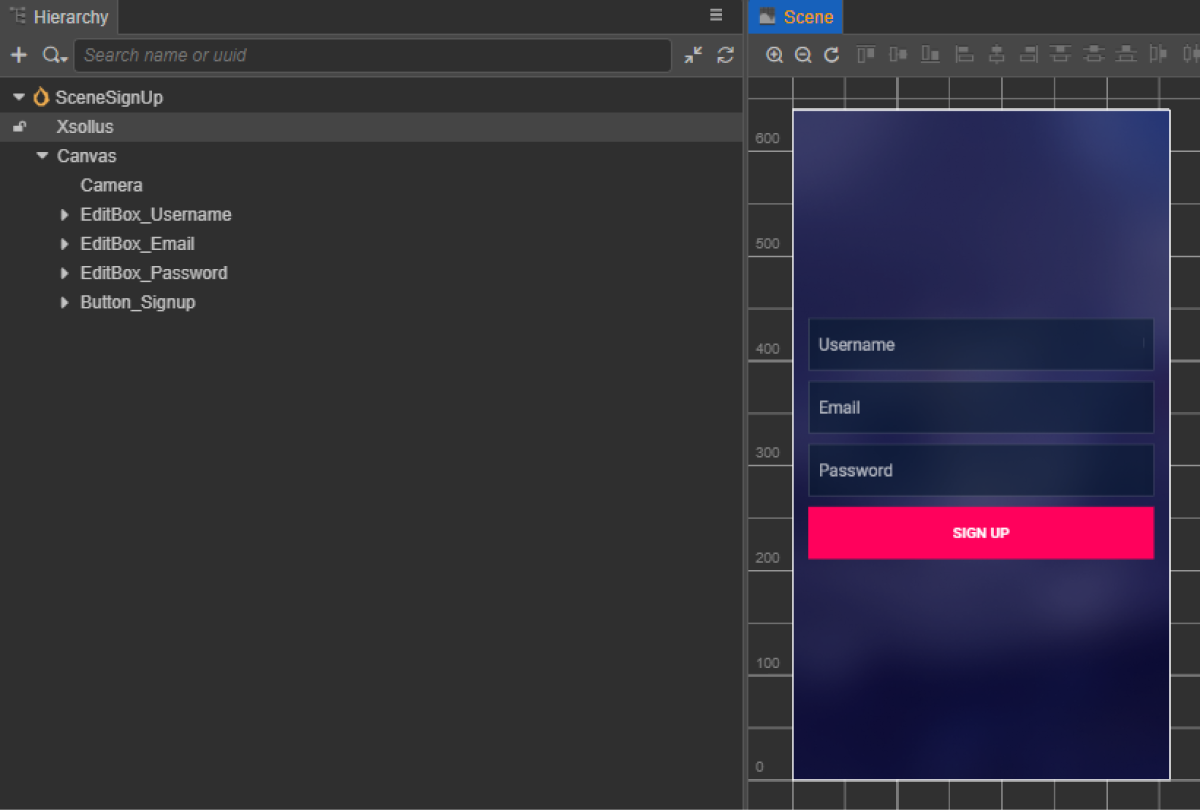
登録スクリプトコンポーネントを作成する
- RegistrationComponentを作成し、次のプロパティを追加します:
usernameEditBox
emailEditBox
passwordEditBox
signUpButton
— 任意。コードを使用してコールバック関数をボタンにバインドするときに使用されます
- スクリプトの例に示すように、
SignUpButton
をクリックしたときに呼び出されるメソッドをRegistrationComponent
クラスに追加し、クリックイベントを処理するロジックを追加します。 - RegistrationComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、シーン要素を
RegistrationComponent
のプロパティにバインドします:
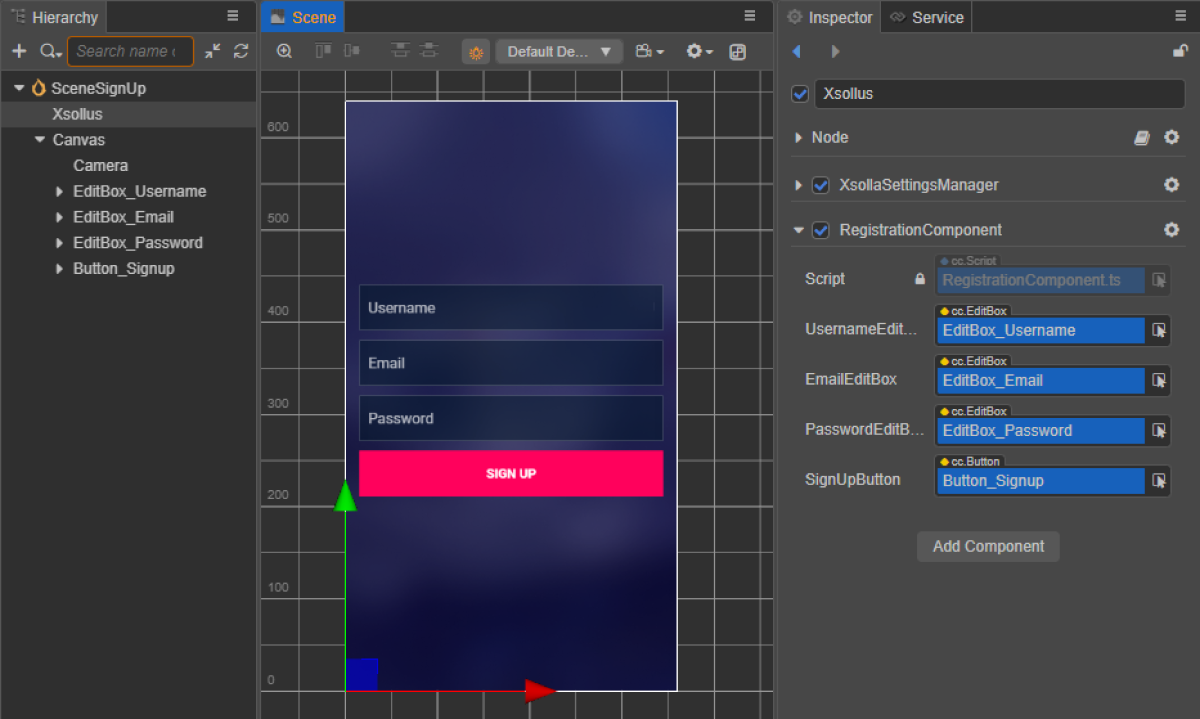
- 以下のいずれかの方法で、新規登録ボタンにコールバックをバインドします:
- 下の図に示すように、
Inspector パネルを使用します。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
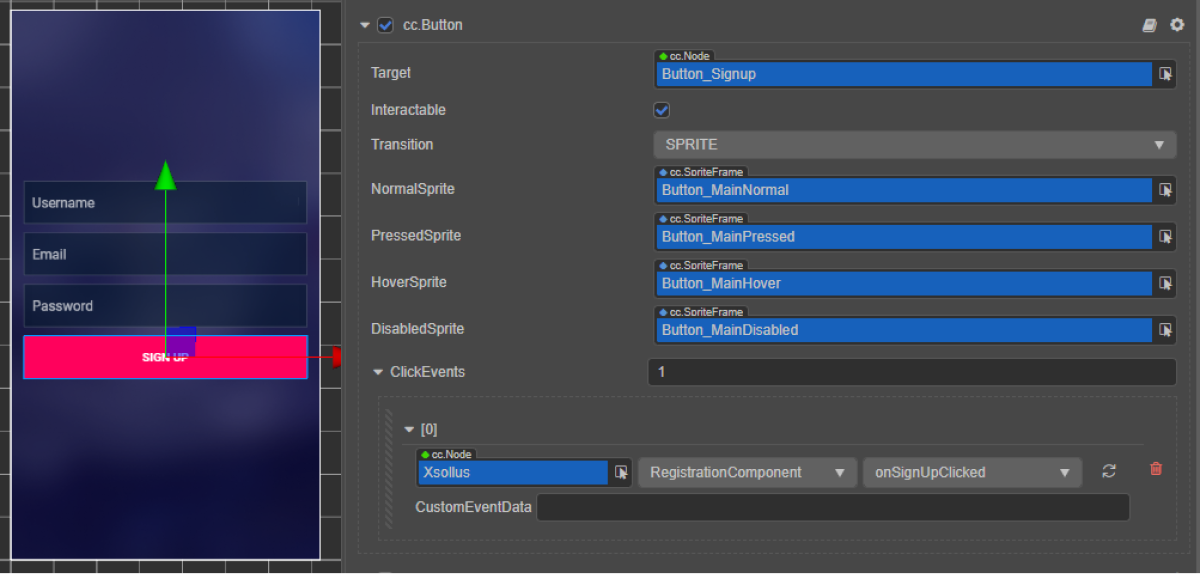
コード経由でコールバックをバインドします:
- typescript
start() {
this.signUpButton.node.on(Button.EventType.CLICK, this.onSignUpClicked, this);
}
スクリプトの例では、onComplete
とonError
メソッドは標準のconsole.log
メソッドを呼び出します。エラーが発生した場合、エラーコードと説明がerror
パラメータで渡されます。
登録メールの再送リクエストのページを開いたり、登録が成功した場合にログインページを開くなど、他のアクションを追加することができます。
- typescript
import { _decorator, Button, Component, EditBox } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
const { ccclass, property } = _decorator;
@ccclass('RegistrationComponent')
export class RegistrationComponent extends Component {
@property(EditBox)
usernameEditBox: EditBox;
@property(EditBox)
emailEditBox: EditBox;
@property(EditBox)
passwordEditBox: EditBox;
@property(Button)
signUpButton: Button;
start() {
this.signUpButton.node.on(Button.EventType.CLICK, this.onSignUpClicked, this);
}
onSignUpClicked() {
XsollaAuth.registerNewUser(this.usernameEditBox.string, this.passwordEditBox.string, this.emailEditBox.string, 'xsollatest', null, null, token => {
if(token != null) {
console.log(`Successful login. Token - ${token.access_token}`);
}
else {
console.log('Thank you! We have sent you a confirmation email');
}
}, err => {
console.log(err);
});
}
}
登録の確認メールを設定する
登録に成功すると、ユーザーは指定したアドレスに登録確認メールを受け取ります。アドミンページで、ユーザーに送られるメールはカスタマイズできます。
モバイルアプリケーションを開発している場合、ユーザーが登録を確認した後、アプリケーションに戻るためのディープリンクをセットアップします。
登録確認メールの再送依頼を実装する
このチュートリアルでは、以下のロジックの実装について説明します:ページのインターフェースを作成する
確認メールの再送信依頼があるページのシーンを作り、そこに以下の要素を追加します:
- ユーザー名/メールアドレスフィールド
- メール再送信ボタン
ページ構造の例:
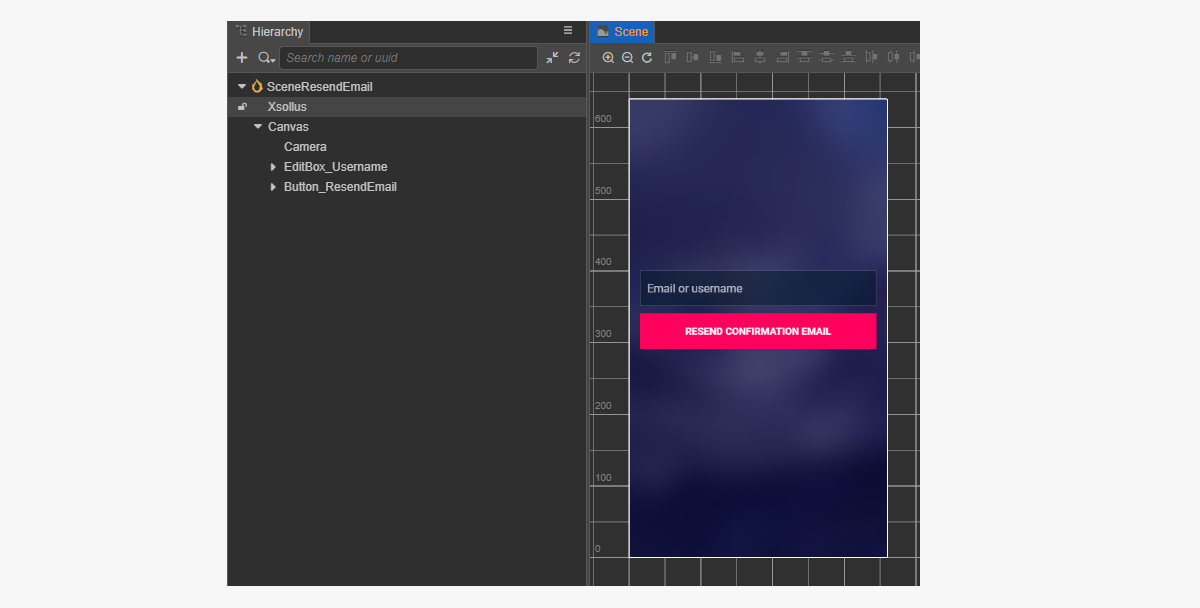
メール再送スクリプトコンポーネントを作成する
- ResendConfirmationEmailComponentを作成し、次のプロパティを追加します:
usernameTextBox
resendEmailButton
— 任意。コードを使用してコールバック関数をボタンにバインドするときに使用されます
- スクリプトの例に示すように、
ResendEmail
をクリックしたときに呼び出されるメソッドをResendConfirmationEmailComponent
クラスに追加し、クリックイベントを処理するロジックを追加します。 - ResendConfirmationEmailComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、シーン要素を
ResendConfirmationEmailComponent
のプロパティにバインドします:
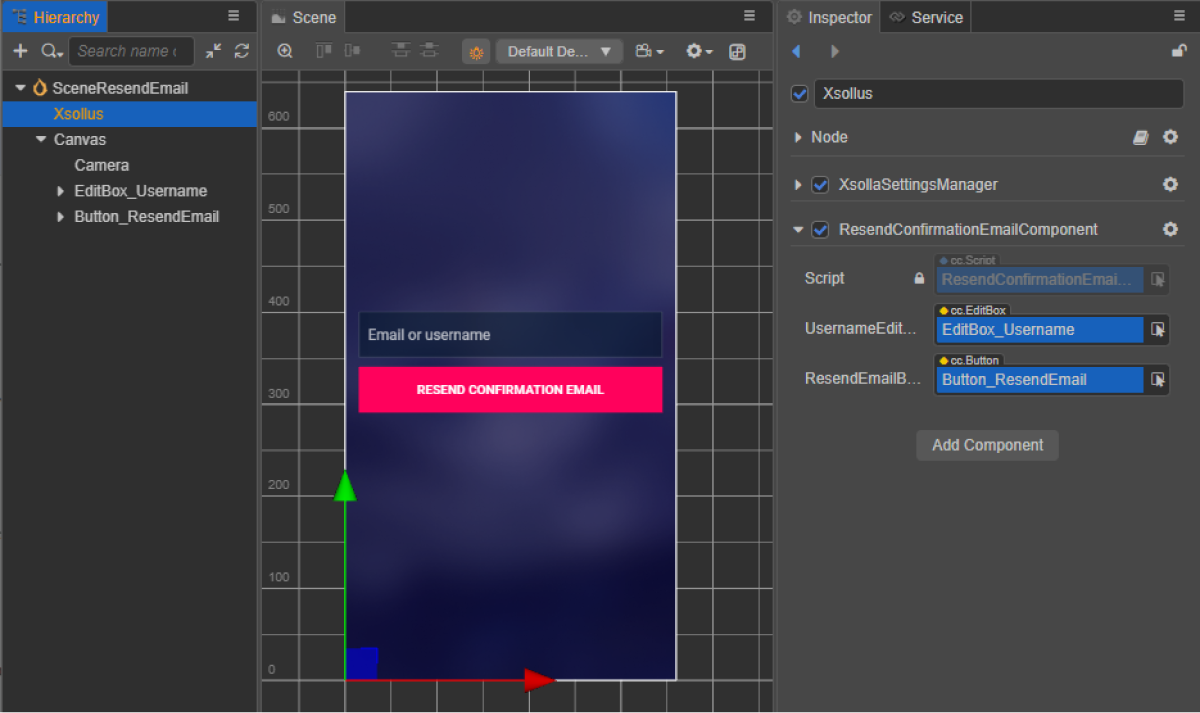
- 以下のいずれかの方法でコールバックを新規登録確認メールのリクエストボタンにバインドします:
- 下の図に示すように、
Inspector パネルを使用する - 以下のコードブロックを、確認メールを再送するリクエストとともにページのスクリプトに挿入する
- 下の図に示すように、
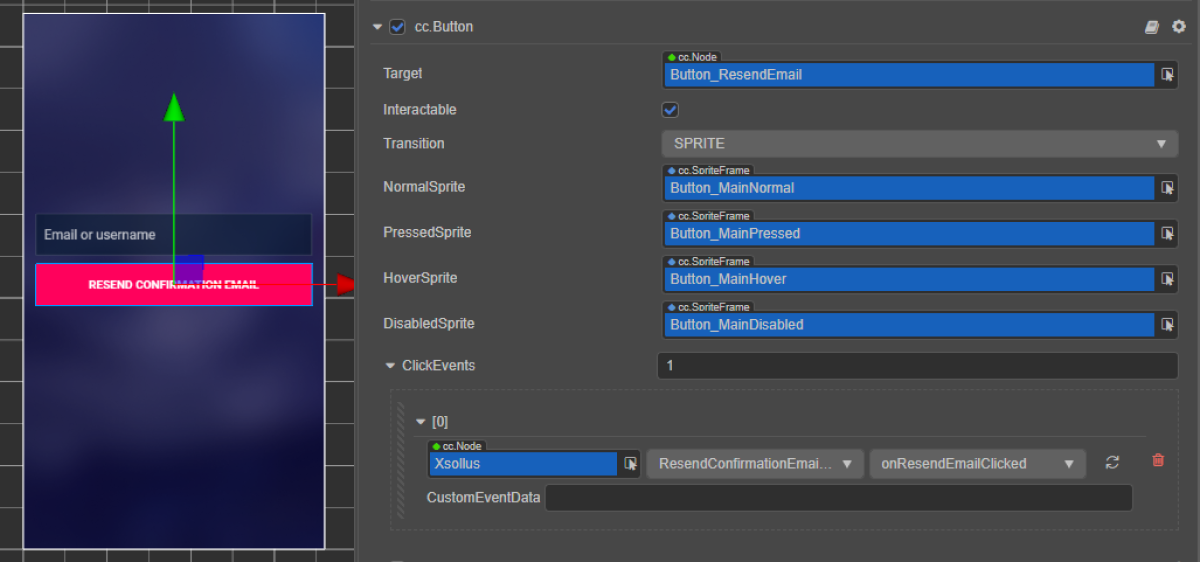
コード経由でコールバックをバインドします:
- typescript
start() {
this.resendEmailButton.node.on(Button.EventType.CLICK, this.onResendEmailClicked, this);
}
リクエストが成功した場合、ユーザーは登録時に指定したメールアドレスに登録確認メールを受け取ります。
スクリプトの例では、onComplete
とonError
メソッドは標準のconsole.log
メソッドを呼び出します。他のアクションを追加することができます。
エラーが発生した場合、エラーコードと説明がerror
パラメータで渡されます。
- typescript
import { _decorator, Button, Component, EditBox } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
const { ccclass, property } = _decorator;
@ccclass('ResendConfirmationEmailComponent')
export class ResendConfirmationEmailComponent extends Component {
@property(EditBox)
usernameEditBox: EditBox;
@property(Button)
resendEmailButton: Button;
start() {
this.resendEmailButton.node.on(Button.EventType.CLICK, this.onResendEmailClicked, this);
}
onResendEmailClicked() {
XsollaAuth.resendAccountConfirmationEmail(this.usernameEditBox.string, 'xsollatest', null, () => {
console.log('A verification link has been successfully sent to your email');
}, err => {
console.log(err);
});
}
}
ユーザーログインを実装する
このチュートリアルでは、以下のロジックの実装について説明します:ページのインターフェースを作成する
ログインページのシーンを作成し、そこに以下の要素を追加します:
- ユーザー名フィールド
- パスワードフィールド
- ログイン状態を保存するトグル
- ログインボタン
ページ構造の例:
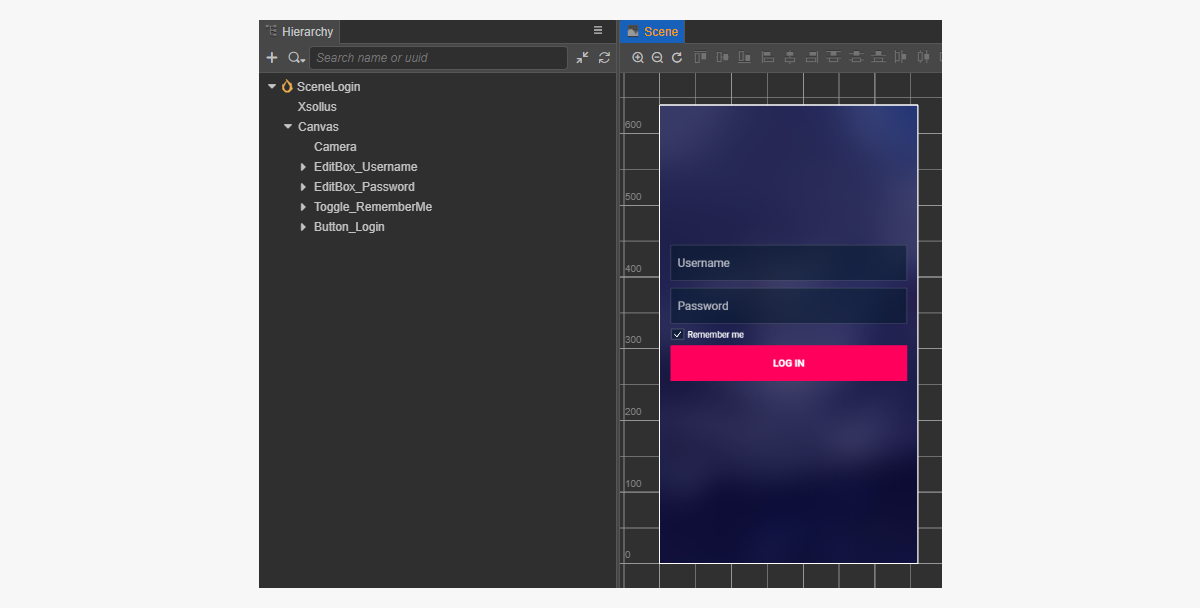
ログインスクリプトコンポーネントを作成する
- LoginComponentを作成し、次のプロパティを追加します:
usernameEditBox
passwordEditBox
remeberMeToggle
loginButton
— 任意。コードを使用してコールバック関数をボタンにバインドするときに使用されます
- スクリプトの例に示すように、
LoginButton
をクリックしたときに呼び出されるメソッドをLoginComponent
クラスに追加し、クリックイベントを処理するロジックを追加します。 - LoginComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、シーン要素を
LoginComponent
のプロパティにバインドします:

- 以下のいずれかの方法でコールバックをログインボタンにバインドします:
- 下の図に示すように、
Inspector パネルを使用します。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
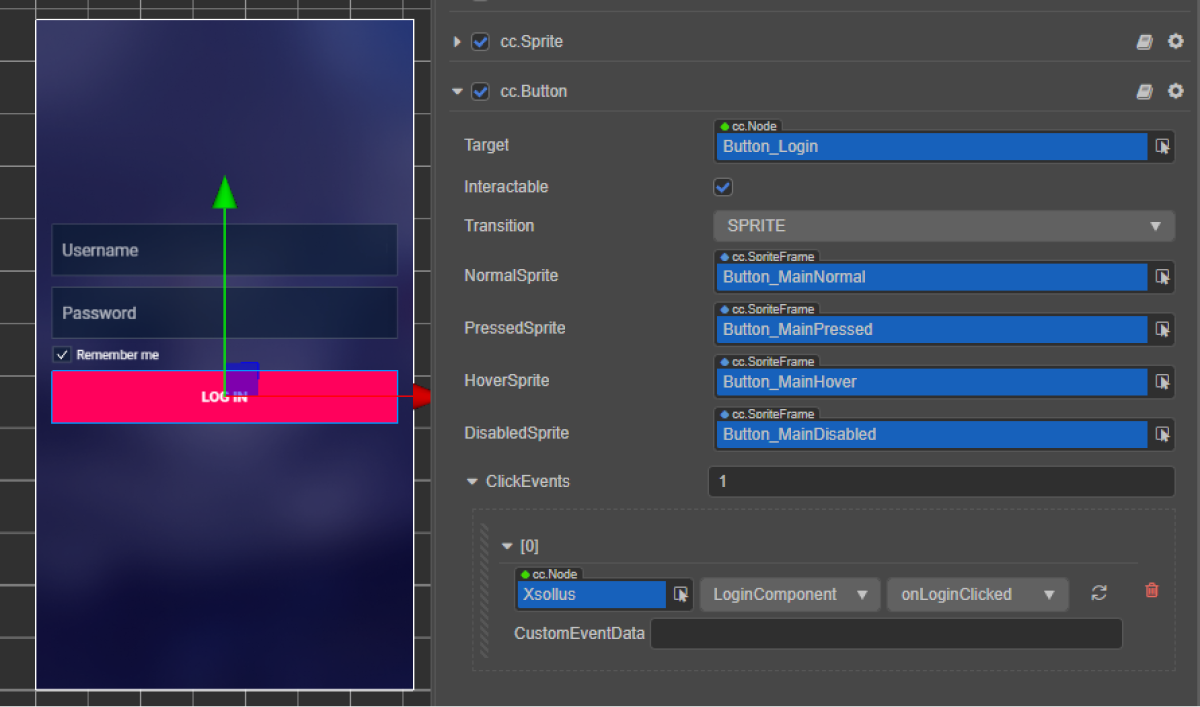
コード経由でコールバックをバインドします:
- typescript
start() {
this.loginButton.node.on(Button.EventType.CLICK, this.onLoginClicked, this);
}
スクリプトの例では、onComplete
とonError
メソッドは標準のconsole.log
メソッドを呼び出します。他のアクションを追加することができます。
エラーが発生した場合、エラーコードと説明がerror
パラメータで渡されます。
- typescript
import { _decorator, Button, Component, EditBox, Toggle } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
const { ccclass, property } = _decorator;
@ccclass('LoginComponent')
export class LoginComponent extends Component {
@property(EditBox)
usernameEditBox: EditBox;
@property(EditBox)
passwordEditBox: EditBox;
@property(Toggle)
remeberMeToggle: Toggle;
@property(Button)
loginButton: Button;
start() {
this.loginButton.node.on(Button.EventType.CLICK, this.onLoginClicked, this);
}
onLoginClicked() {
XsollaAuth.authByUsernameAndPassword(this.usernameEditBox.string, this.passwordEditBox.string, this.remeberMeToggle.isChecked, token => {
console.log('Successful login. Token - ${token.access_token}');
}, err => {
console.log(err);
});
}
}
パスワードリセットを実装する
このチュートリアルでは、以下のロジックの実装について説明します:ページのインターフェースを作成する
パスワードのリセットページのシーンを作成し、以下を追加します:
- ユーザー名フィールド
- パスワードリセットボタン
ページ構造の例:
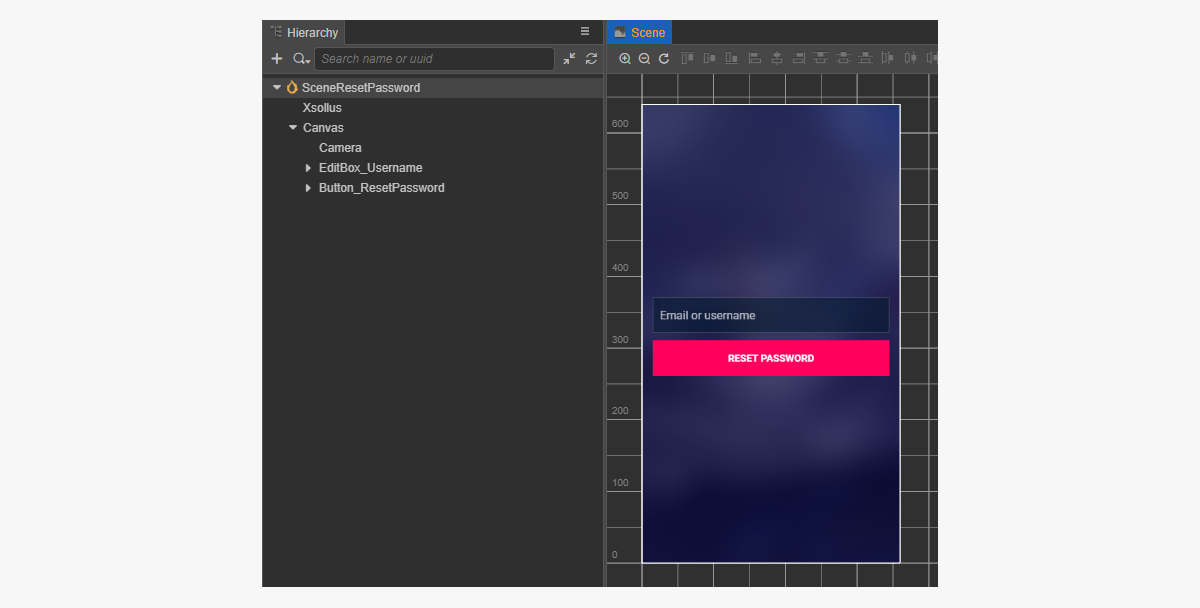
パスワードリセットスクリプトコンポーネントを作成する
- ResetPasswordComponentを作成し、次のプロパティを追加します:
usernameEditBox
resetPasswordButton
— 任意。コードを使用してコールバック関数をボタンにバインドするときに使用されます
- スクリプトの例に示すように、
ResetPassword
をクリックしたときに呼び出されるメソッドをResetPasswordComponent
クラスに追加し、クリックイベントを処理するロジックを追加します。 - ResetPasswordComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、シーン要素を
ResetPasswordComponent
のプロパティにバインドします:
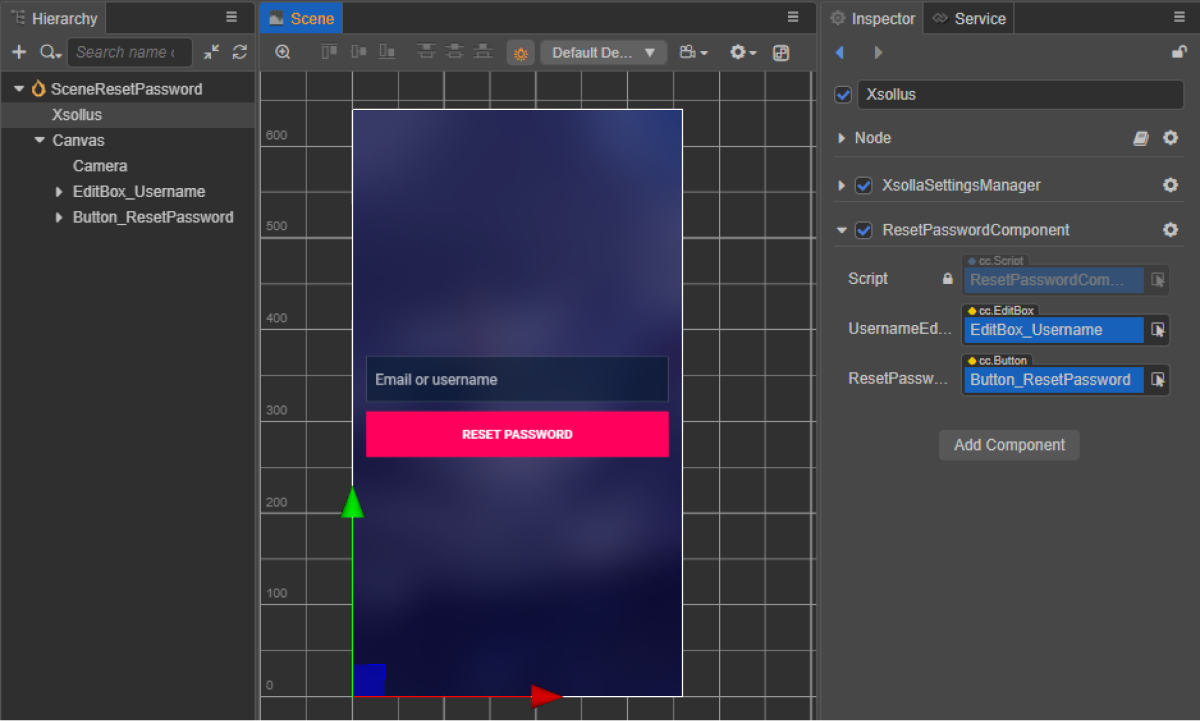
- 以下のいずれかの方法でコールバックをパスワードリセットボタンにバインドします:
- 下の図に示すように、
Inspector パネルを使用します。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
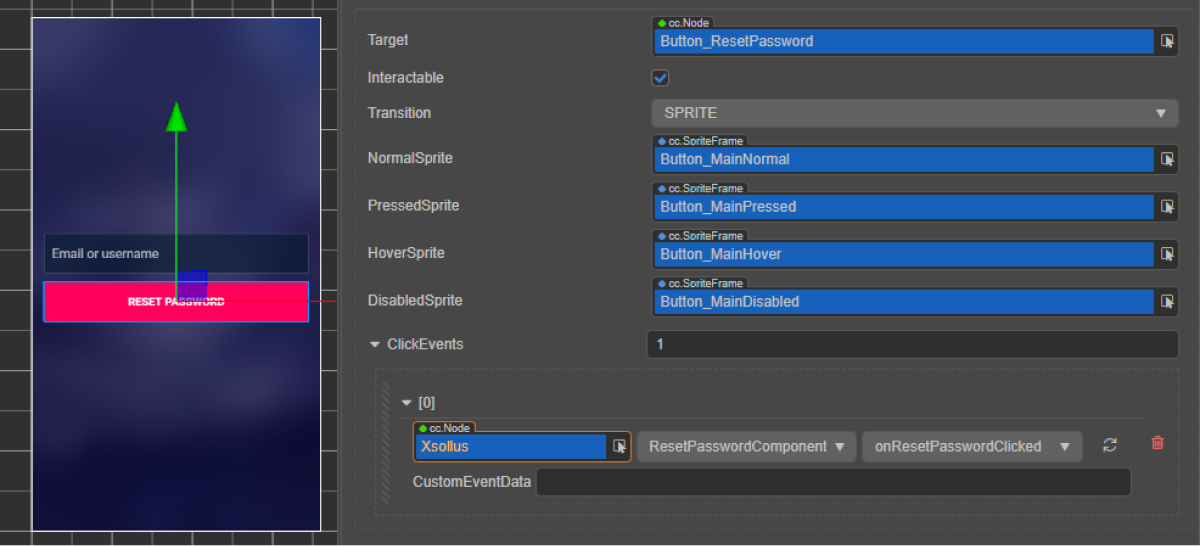
コード経由でコールバックをバインドします:
- typescript
start() {
this.resetPasswordButton.node.on(Button.EventType.CLICK, this.onResetPasswordClicked, this);
}
スクリプトの例では、onComplete
とonError
メソッドは標準のconsole.log
メソッドを呼び出します。他のアクションを追加することができます。
エラーが発生した場合、エラーコードと説明がerror
パラメータで渡されます。
- typescript
import { _decorator, Button, Component, EditBox } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
const { ccclass, property } = _decorator;
@ccclass('ResetPasswordComponent')
export class ResetPasswordComponent extends Component {
@property(EditBox)
usernameEditBox: EditBox;
@property(Button)
resetPasswordButton: Button;
start() {
this.resetPasswordButton.node.on(Button.EventType.CLICK, this.onResetPasswordClicked, this);
}
onResetPasswordClicked() {
XsollaAuth.resetPassword(this.usernameEditBox.string, null, () => {
console.log('Follow the instructions we sent to your email');
}, err => {
console.log(err);
});
}
}
ソーシャルログイン
このガイドでは、SDKメソッドを使用して、ユーザーのサインアップとソーシャルネットワークアカウントによるログインを実装する方法を紹介します。
ユーザー名/ユーザーのメールアドレスとパスワードによるユーザー認証とは異なり、ユーザーのサインアップのために別のロジックを実装する必要はありません。ユーザーの初回ログインがソーシャルネットワーク経由の場合、新しいアカウントが自動的に作成されます。
別の認証方法としてアプリケーションにソーシャルログインを実装している場合、以下の条件を満たすと、ソーシャルネットワークアカウントは既存のユーザーアカウントに自動的にリンクします。
- ユーザー名/メールアドレスとパスワードでサインアップしたユーザーが、ソーシャルネットワークアカウントを介してアプリケーションにログインしました。
- ソーシャルネットワークでは、メールアドレスを返します。
- ソーシャルネットワークでのユーザーのメールアドレスは、アプリケーションで登録に使用したメールアドレスと同じです。
このチュートリアルでは、以下のロジックの実装について説明します:
この例では、Facebookアカウントによるユーザーログインを設定しています。すべてのソーシャルネットワークを同じ方法で設定できます。
この例では、SDKメソッドの基本的な概要を提供します。アプリケーションは通常、より複雑なインターフェースとロジックを必要とします。
ページのインターフェースを作成する
ソーシャルログインページのシーンを作成し、それにソーシャルログインボタンを追加します。
ページ構造の例:
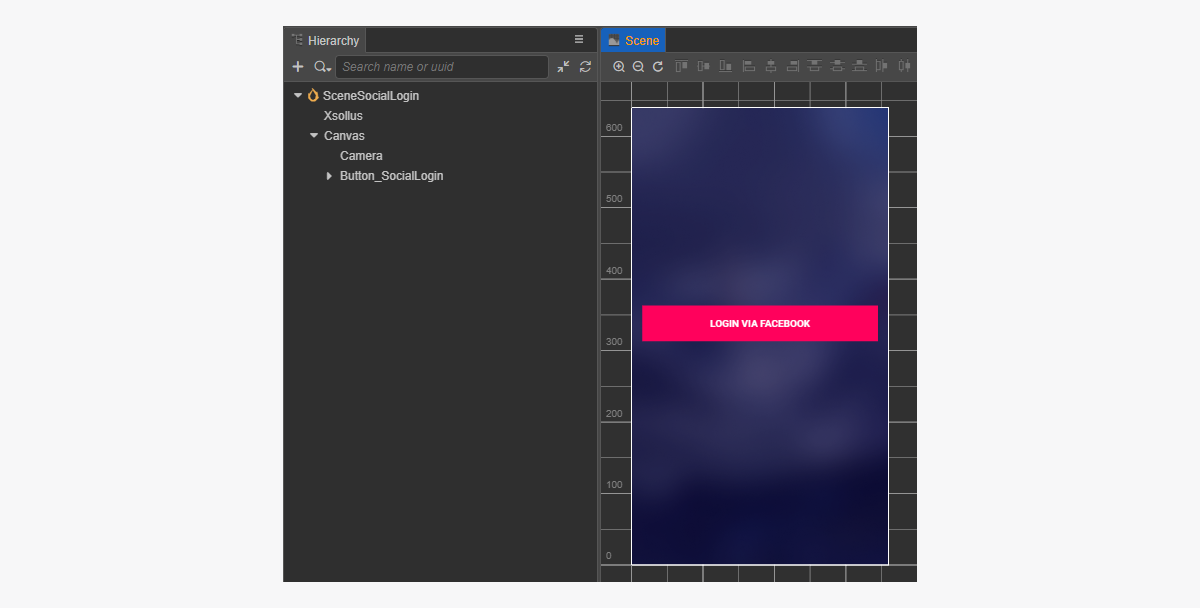
ソーシャルログインスクリプトコンポーネントを作成する
- SocialLoginComponentを作成します。コードを使用してソーシャルログインボタンにコールバック関数をバインドするには、
socialLoginButton
属性を追加します。 - スクリプトの例に示すように、
SocialLogin
をクリックしたときに呼び出されるメソッドをSocialLoginComponent
クラスに追加し、クリックイベントを処理するロジックを追加します。
- SocialLoginComponentをシーン上のノードに追加します。SDK初期化で追加した
XsollaSettingsManager
コンポーネントを使用して、新しいノードを追加するか、既存のノードを使用することができます。 - 図に示すように、
SocialLogin
ボタンをSocialLoginComponent
のsocialLoginButton
属性にバインドします。
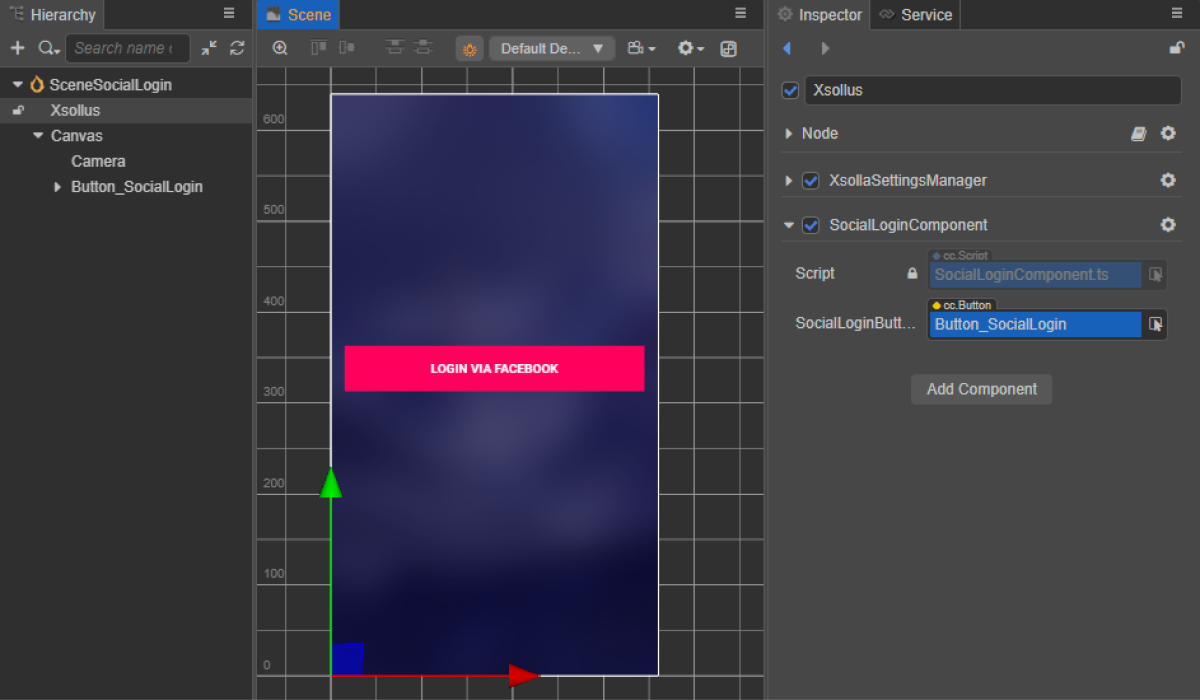
- 以下のいずれかの方法で、ソーシャルログインボタンにコールバックをバインドします:
- 下の図に示すように、
Inspector パネルを使用します。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
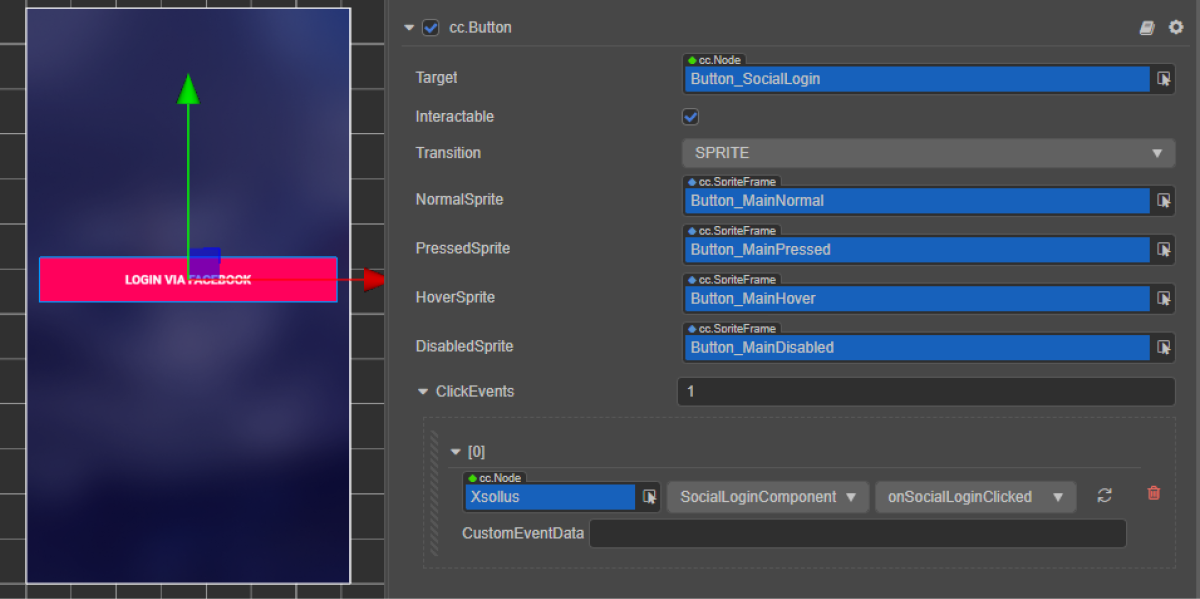
コード経由でコールバックをバインドします:
- typescript
start() {
this.socialLoginButton.node.on(Button.EventType.CLICK, this.onSocialLoginClicked, this);
}
スクリプトの例では、onComplete
、onCanceled
及びonError
メソッドは標準のconsole.log
メソッドを呼び出します。エラーが発生した場合、エラーコードと説明がerror
パラメータで渡されます。
登録メールの再送リクエストのページを開いたり、登録が成功した場合にログインページを開くなど、他のアクションを追加することができます。
- typescript
import { _decorator, Button, Component } from 'cc';
import { Token, XsollaAuth } from '../../api/XsollaAuth';
const { ccclass, property } = _decorator;
namespace authorization {
@ccclass('SocialLoginComponent')
export class SocialLoginComponent extends Component {
@property(Button)
socialLoginButton: Button;
start() {
this.socialLoginButton.node.on(Button.EventType.CLICK, this.onSocialLoginClicked, this);
}
onSocialLoginClicked() {
XsollaAuth.authSocial('facebook', (token: Token) => {
console.log(`Successful social authentication. Token - ${token.access_token}`);
}, () => {
console.log('Social auth was canceled');
}, (error:string) => {
console.log(error);
});
}
}
}
アイテムカタログの表示
このチュートリアルでは、SDKメソッドを使って、インゲームストアに以下のアイテムを表示する方法を紹介します:
- 仮想アイテム
- バンドル
- 仮想通貨のパッケージ
始める前に、アドミンページの項目を設定します:
このチュートリアルでは、以下のロジックの実装について説明します:
カタログ内のすべてのアイテムの例は次のとおり:
- 名称
- 説明
- 価格
- 画像
また、アイテムに関する他の情報がインゲームストアに保存されている場合は、その情報を表示することもできます。
仮想アイテムの表示を実装する
アイテムウィジェットを作成する
- プレハブを作成します。これを行うには、フォルダーのコンテキストメニューから
Create > Node Prefab を選択します。 - 作成したプレハブを開きます。
- 以下の画像に示すように、
UITransform
コンポーネントをプレハブのルートに追加し、コンテンツサイズを設定します。
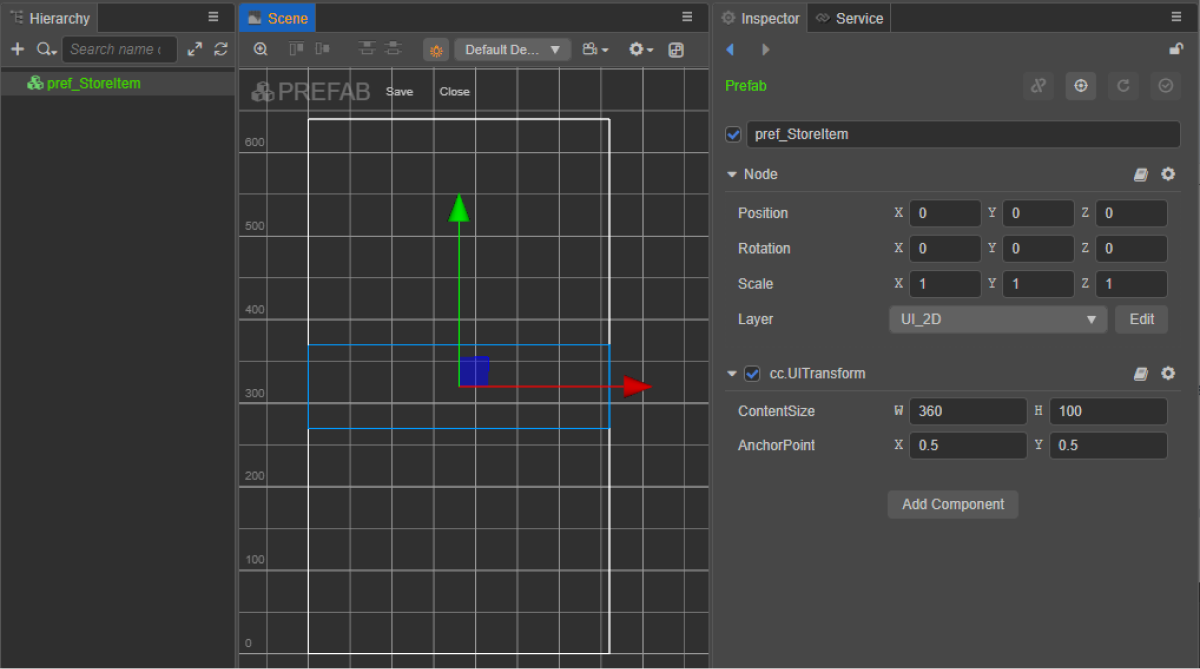
- 以下のUI要素をプレハブ子オブジェクトとして追加し、視覚化を構成します:
- ウィジェットの背景画像
- アイテム名
- アイテム説明
- アイテム価格
- アイテムイメージ
ウィジェット構造の例:
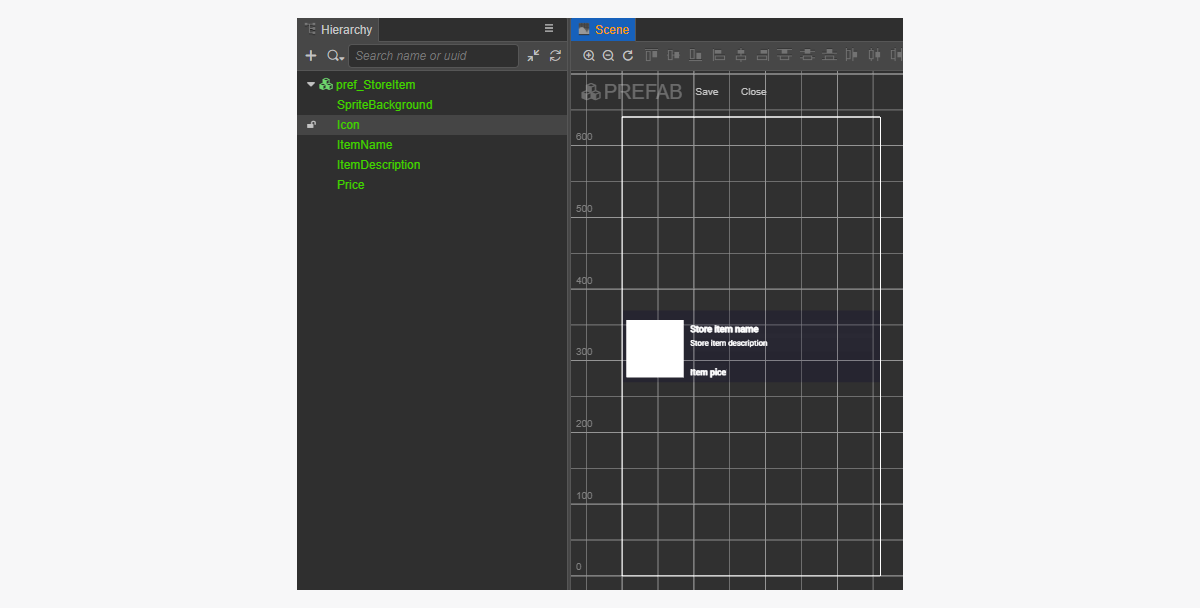
アイテムウィジェットスクリプトを作成する
StoreItemComponent
を作成し、以下のプロパティを追加します:iconSprite
itemNameLabel
itemDescriptionLabel
priceLabel
- スクリプトの例に示すように、
init
メソッドと初期化ロジックをStoreItemComponent
クラスに追加します。 - StoreItemComponentコンポーネントをプレハブのルートノードにアタッチします。
- 図に示すように、プレハブ要素を
StoreItemComponent
のプロパティにバインドします:
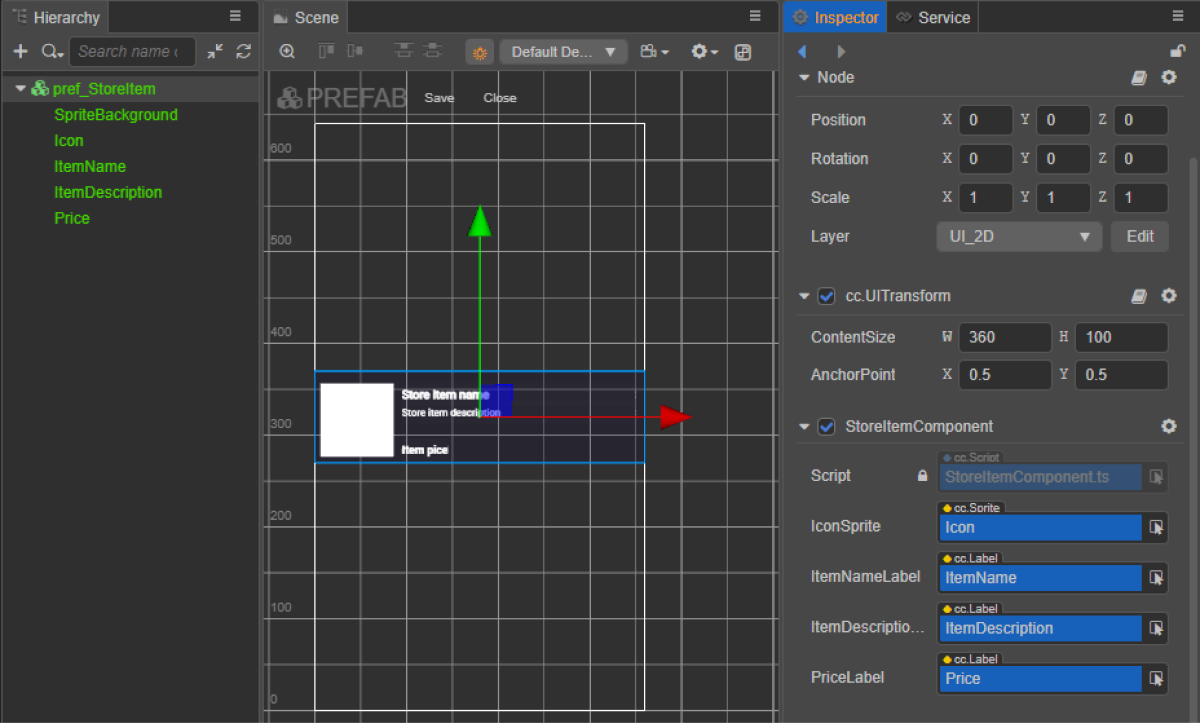
ウィジェットスクリプト(StoreItemComponent)の例:
- typescript
import { _decorator, assetManager, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { StoreItem } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
const { ccclass, property } = _decorator;
@ccclass('StoreItemComponent')
export class StoreItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
itemNameLabel: Label;
@property(Label)
itemDescriptionLabel: Label;
@property(Label)
priceLabel: Label;
private _data: StoreItem;
init(data: StoreItem) {
this._data = data;
this.itemNameLabel.string = data.name;
this.itemDescriptionLabel.string = data.description;
if (data.virtual_prices.length > 0) {
this.priceLabel.string = data.virtual_prices[0].amount.toString() + ' ' + data.virtual_prices[0].name;
} else {
this.priceLabel.string = parseFloat(data.price.amount) + ' ' + data.price.currency;
}
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
}
}
ページのインターフェースを作成する
アイテムカタログページのシーンを作成し、それにScrollView
要素を追加します。
ページ構造の例:
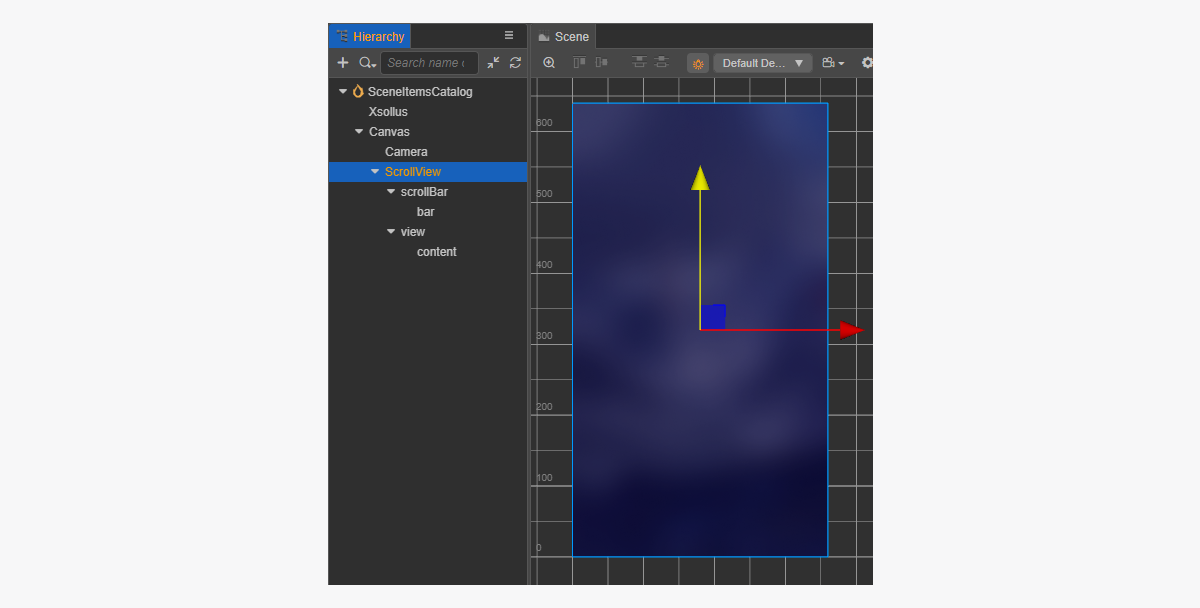
ScrollView
を作成したStoreItem
プレハブと一致させるには、そのサイズを設定します:
ScrollView
ノードと内部のview
ノードのContentSize
パラメータの値を変更します。Layout
コンポーネントをcontent
ノードにバインドして設定します。この例では、次の設定が選択されています:Type == vertical
ResizeMode == Container
アイテムカタログスクリプトコンポーネントを作成する
- ItemsCatalogComponentを作成し、以下のプロパティを追加します:
itemsScrollView
storeItemPrefab
- スクリプトの例に示すように、
start ライフサイクル関数と初期化ロジックをItemsCatalogComponent
クラスに追加します。 - ItemsCatalogComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、プレハブ要素を
ItemsCatalogComponent
のプロパティにバインドします:
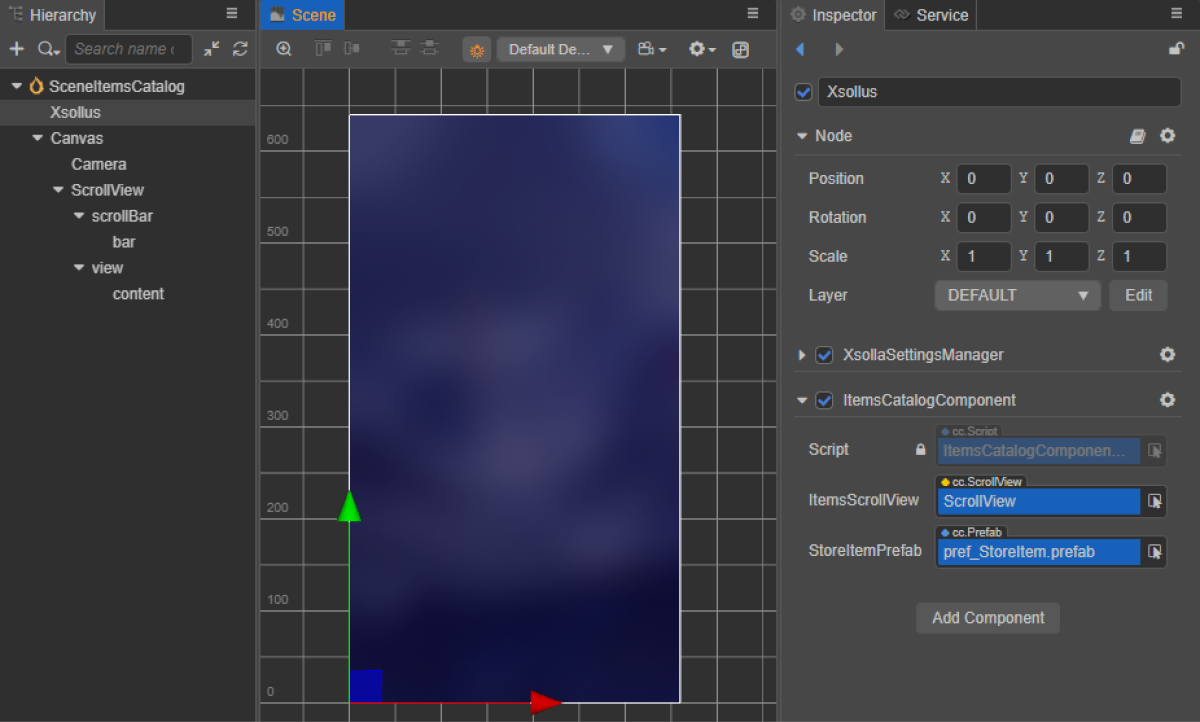
クラススクリプト(ItemsCatalogComponent)の例:
- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { StoreItemComponent } from './StoreItemComponent';
const { ccclass, property } = _decorator;
@ccclass('ItemsCatalogComponent')
export class ItemsCatalogComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
storeItemPrefab: Prefab;
start() {
XsollaCatalog.getCatalog(null, null, [], itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let storeItem = instantiate(this.storeItemPrefab);
this.itemsScrollView.content.addChild(storeItem);
storeItem.getComponent(StoreItemComponent).init(itemsData.items[i]);
}
});
}
}
スクリプトの動作結果の例:
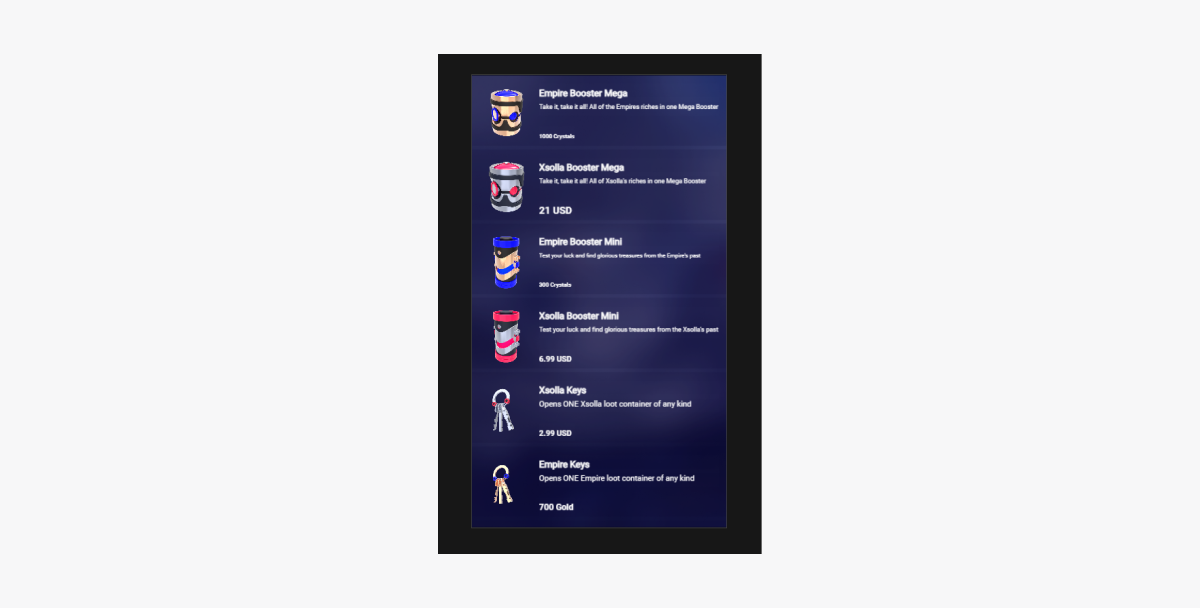
バンドルの表示の実装
バンドルウィジェットを作成する
- プレハブを作成します。これを行うには、フォルダーのコンテキストメニューから
Create > Node Prefab を選択します。 - 作成したプレハブを開きます。
- 以下の画像に示すように、
UITransform
コンポーネントをプレハブのルートに追加し、コンテンツサイズを設定します。
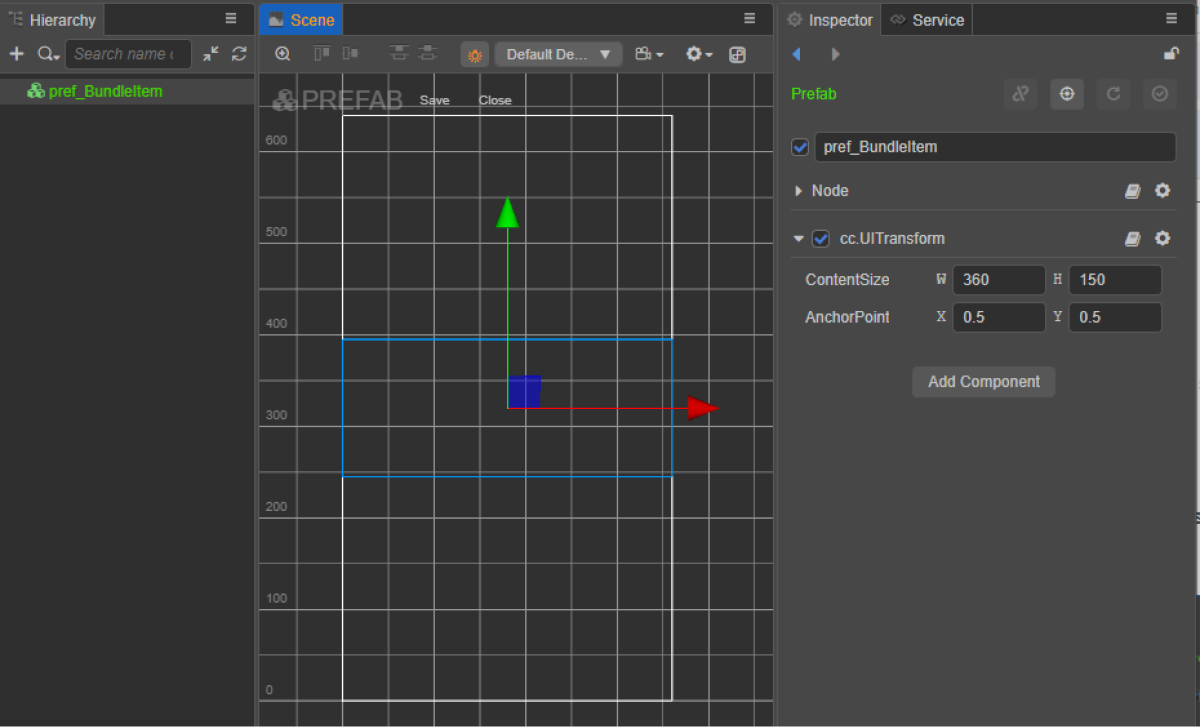
- 以下のUI要素をプレハブ子オブジェクトとして追加し、視覚化を構成します:
- ウィジェットの背景画像
- バンドル名
- バンドルの説明
- バンドル価格
- バンドルイメージ
- バンドルコンテンツの説明(アイテムおよびその数量)
ウィジェット構造の例:
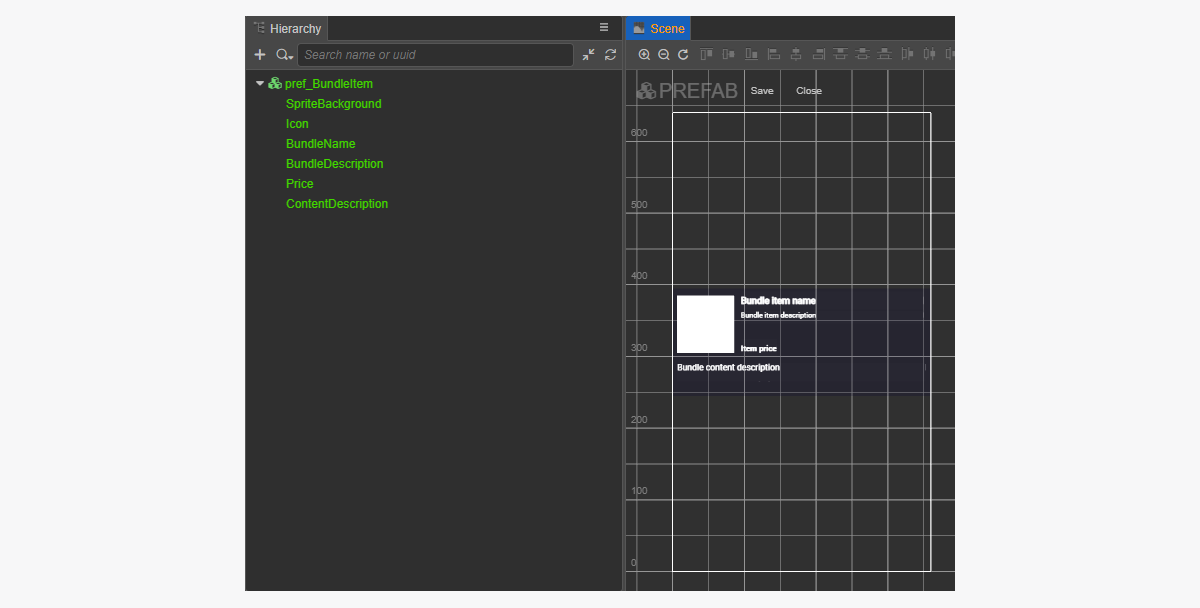
バンドルウィジェットスクリプトを作成する
- BundleItemComponentを作成し、以下のプロパティを追加します:
iconSprite
bundleNameLabel
bundleDescriptionLabel
priceLabel
contentDescriptionlabel
- スクリプトの例に示すように、
init
メソッドと初期化ロジックをBundleItemComponent
クラスに追加します。 - BundleItemComponentをプレハブのルートノードにアタッチします。
- 図に示すように、プレハブ要素を
BundleItemComponent
のプロパティにバインドします:
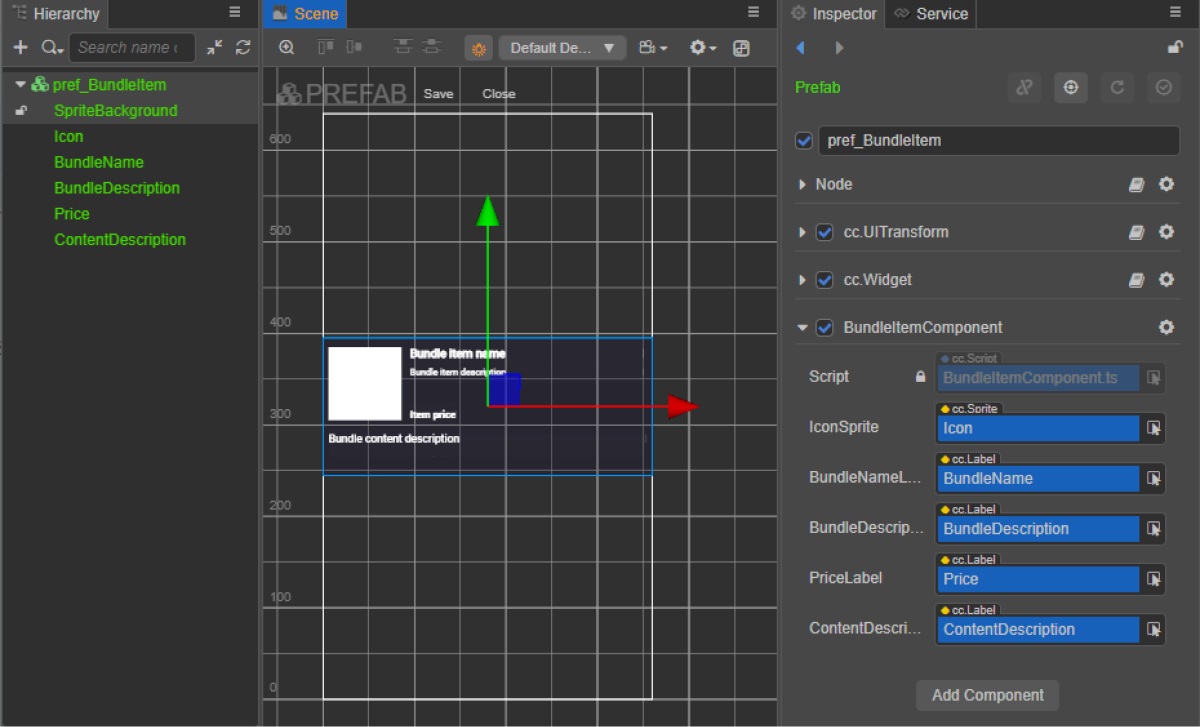
ウィジェットスクリプト(BundleItemComponent)の例:
- typescript
import { _decorator, assetManager, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { StoreBundle } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
const { ccclass, property } = _decorator;
@ccclass('BundleItemComponent')
export class BundleItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
bundleNameLabel: Label;
@property(Label)
bundleDescriptionLabel: Label;
@property(Label)
priceLabel: Label;
@property(Label)
contentDescriptionLabel: Label;
init(data: StoreBundle) {
this.bundleNameLabel.string = data.name;
this.bundleDescriptionLabel.string = data.description;
if (data.virtual_prices.length > 0) {
this.priceLabel.string = data.virtual_prices[0].amount.toString() + ' ' + data.virtual_prices[0].name;
} else {
this.priceLabel.string = parseFloat(data.price.amount) + ' ' + data.price.currency;
}
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
this.contentDescriptionLabel.string = 'This bundle includes '+ data.content.length + ' items: ';
var bandles = data.content.map(bundle => bundle.name).join(', ');
this.contentDescriptionLabel.string += bandles;
}
}
ページのインターフェースを作成する
バンドルカタログページのシーンを作成し、それにScrollView
要素を追加します。
ページ構造の例:
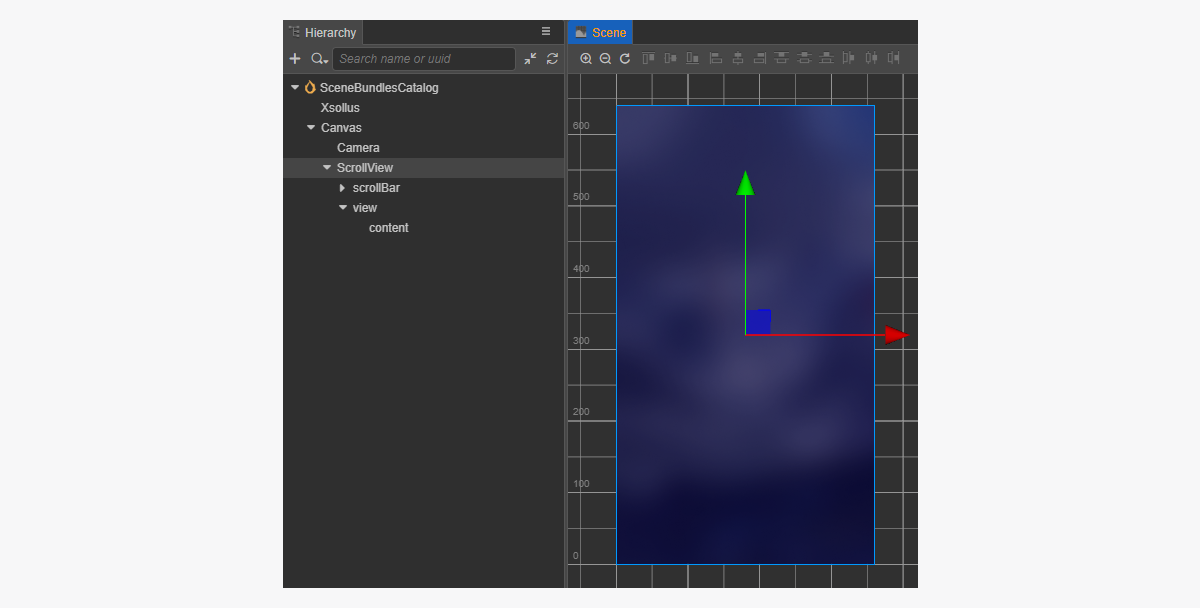
ScrollView
を作成したBundleItem
プレハブと一致させるには、そのサイズを設定します:
ScrollView
ノードと内部のview
ノードのContentSize
パラメータの値を変更します。Layout
コンポーネントをcontent
ノードにバインドして設定します。この例では、次の設定が選択されています:Type == vertical
ResizeMode == Container
バンドルカタログスクリプトコンポーネントを作成する
- BundlesCatalogComponentを作成し、以下のプロパティを追加します:
itemsScrollView
bundleItemPrefab
- スクリプトの例に示すように、
start ライフサイクル関数と初期化ロジックをBundlesCatalogComponent
クラスに追加します。 - BundlesCatalogComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、プレハブ要素を
BundlesCatalogComponent
のプロパティにバインドします:
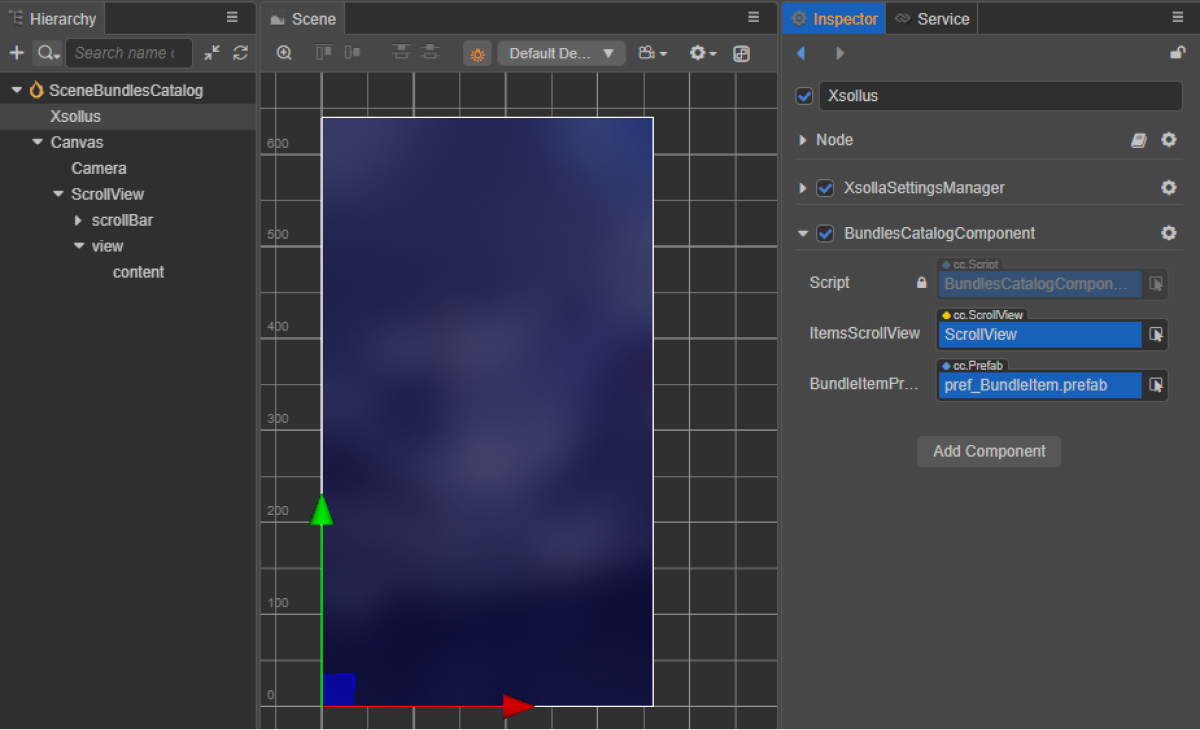
クラススクリプト(BundlesCatalogComponent)の例:
- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { BundleItemComponent } from './BundleItemComponent';
const { ccclass, property } = _decorator;
@ccclass('BundlesCatalogComponent')
export class BundlesCatalogComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
bundleItemPrefab: Prefab;
start() {
XsollaCatalog.getBundleList(null, null, [], itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let bundleItem = instantiate(this.bundleItemPrefab);
this.itemsScrollView.content.addChild(bundleItem);
bundleItem.getComponent(BundleItemComponent).init(itemsData.items[i]);
}
});
}
}
スクリプトの動作結果の例:
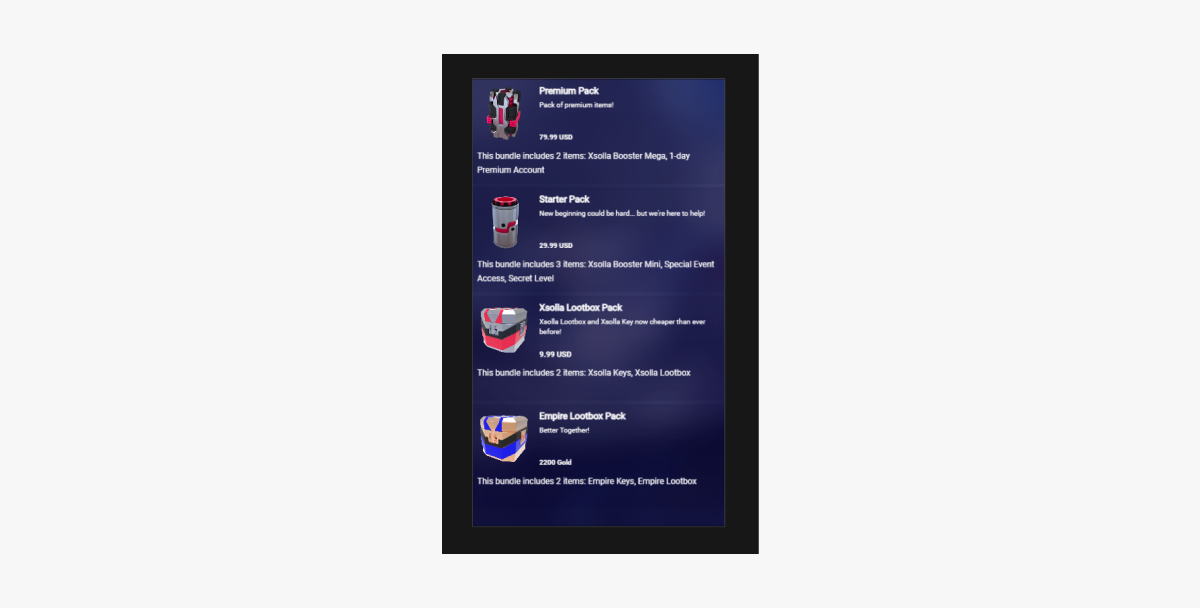
仮想通貨パッケージカタログの表示を実装する
仮想通貨パッケージのウィジェットを作成する
- プレハブを作成します。これを行うには、フォルダーのコンテキストメニューから
Create > Node Prefab を選択します。 - 作成したプレハブを開きます。
- 以下の画像に示すように、
UITransform
コンポーネントをプレハブのルートに追加し、コンテンツサイズを設定します。
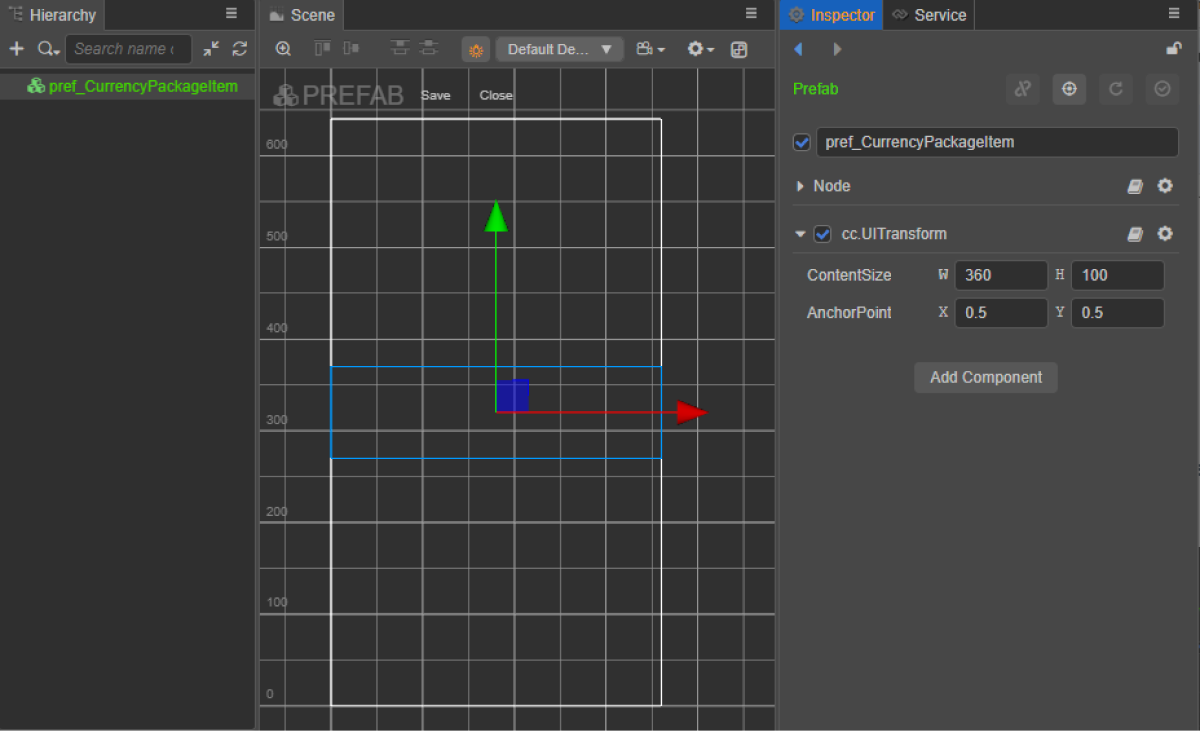
- 以下のUI要素をプレハブ子オブジェクトとして追加し、視覚化を構成します:
- ウィジェットの背景画像
- 通貨名
- 通貨説明
- 通貨価格
- 通貨イメージ
ウィジェット構造の例:
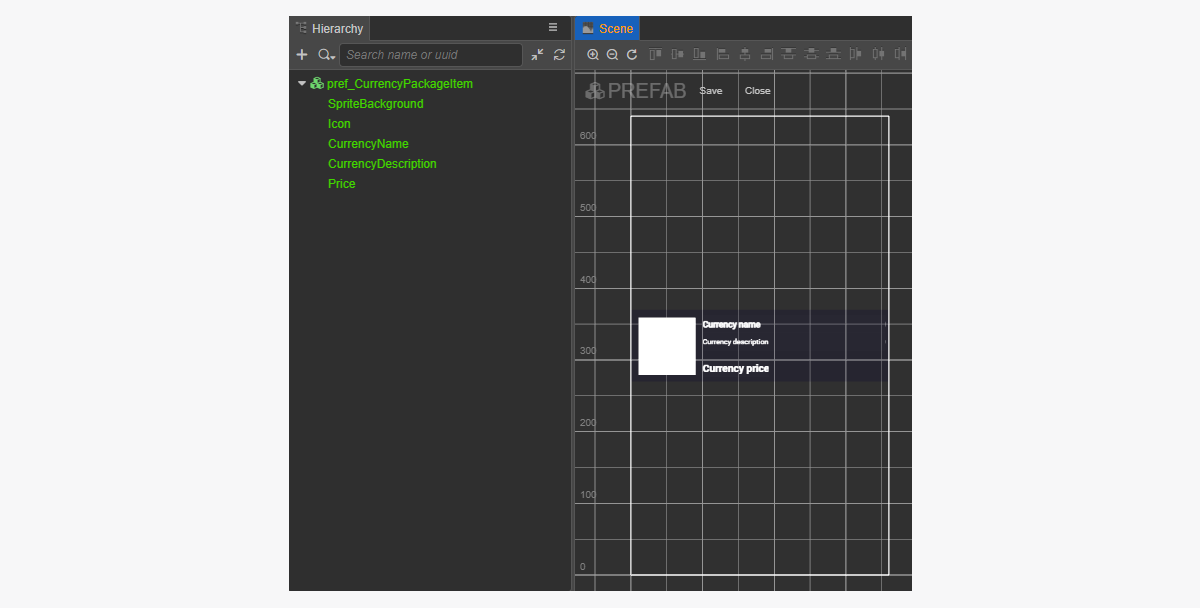
仮想通貨パッケージ用ウィジェットスクリプトを作成する
- CurrencyPackageItemComponentを作成し、以下のプロパティを追加します:
iconSprite
currencyNameLabel
currencyDescriptionLabel
priceLabel
- スクリプトの例に示すように、
init
メソッドと初期化ロジックをCurrencyPackageItemComponent
クラスに追加します。 - CurrencyPackageItemComponentコンポーネントをプレハブのルートノードにアタッチします。
- 図に示すように、プレハブ要素を
CurrencyPackageItemComponent
のプロパティにバインドします:
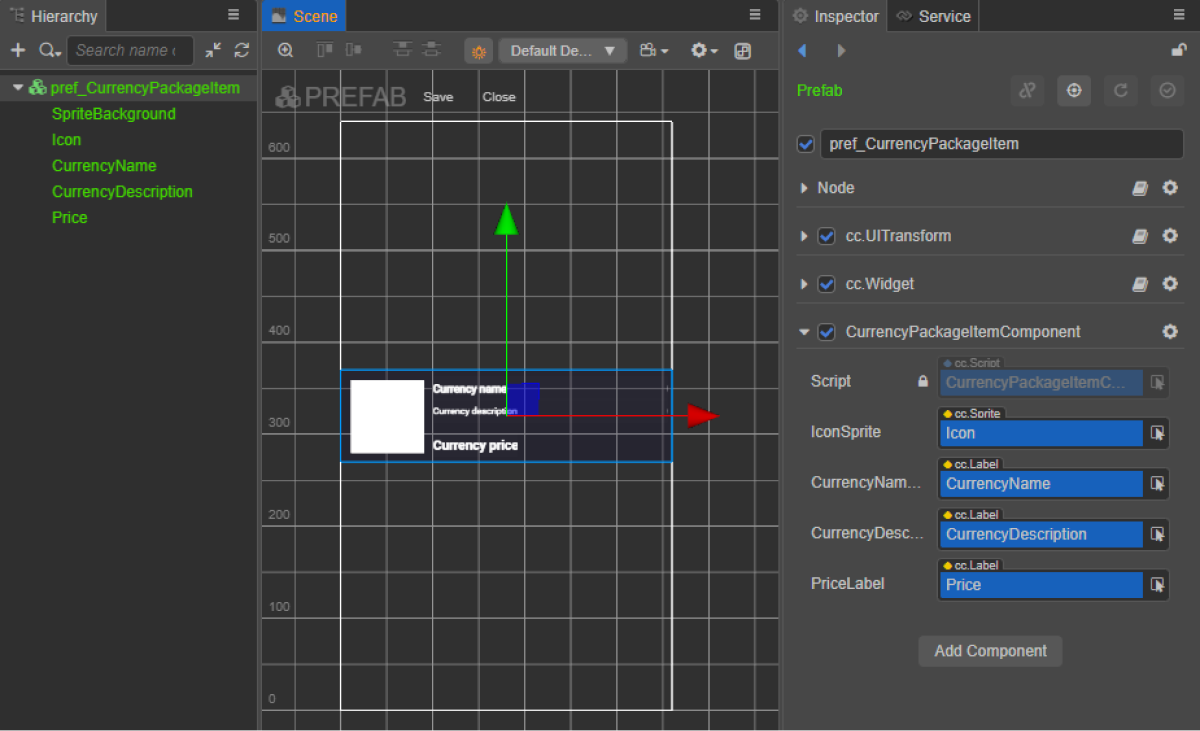
ウィジェットスクリプト(CurrencyPackageItemComponent)の例:
- typescript
import { _decorator, assetManager, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { VirtualCurrencyPackage } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
const { ccclass, property } = _decorator;
@ccclass('CurrencyPackageItemComponent')
export class CurrencyPackageItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
currencyNameLabel: Label;
@property(Label)
currencyDescriptionLabel: Label;
@property(Label)
priceLabel: Label;
init(data: VirtualCurrencyPackage) {
this.currencyNameLabel.string = data.name;
this.currencyDescriptionLabel.string = data.description;
if (data.virtual_prices.length > 0) {
this.priceLabel.string = data.virtual_prices[0].amount.toString() + ' ' + data.virtual_prices[0].name;
} else {
this.priceLabel.string = parseFloat(data.price.amount) + ' ' + data.price.currency;
}
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
}
}
ページのインターフェースを作成する
仮想通貨パッケージカタログページのシーンを作成し、それにScrollView
要素を追加します。
ページ構造の例:
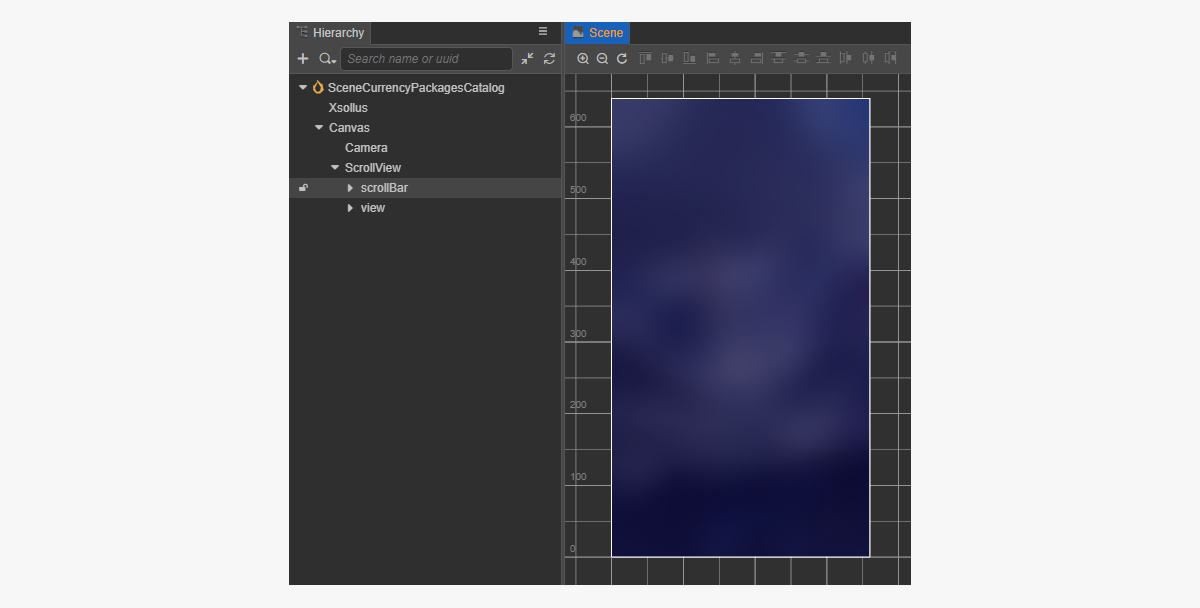
ScrollView
を作成したCurrencyPackageItem
プレハブと一致させるには、そのサイズを設定します:
ScrollView
ノードと内部のview
ノードのContentSize
パラメータの値を変更します。Layout
コンポーネントをcontent
ノードにバインドして設定します。この例では、次の設定が選択されています:Type == vertical
ResizeMode == Container
通貨パッケージカタログスクリプトコンポーネントを作成する
CurrencyPackagesCatalogComponent
を作成し、以下のプロパティを追加します:itemsScrollView
currencyPackageItemPrefab
- スクリプトの例に示すように、
start ライフサイクル関数と初期化ロジックをCurrencyPackagesCatalogComponent
クラスに追加します。 - CurrencyPackagesCatalogComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、プレハブ要素を
CurrencyPackagesCatalogComponent
のプロパティにバインドします:
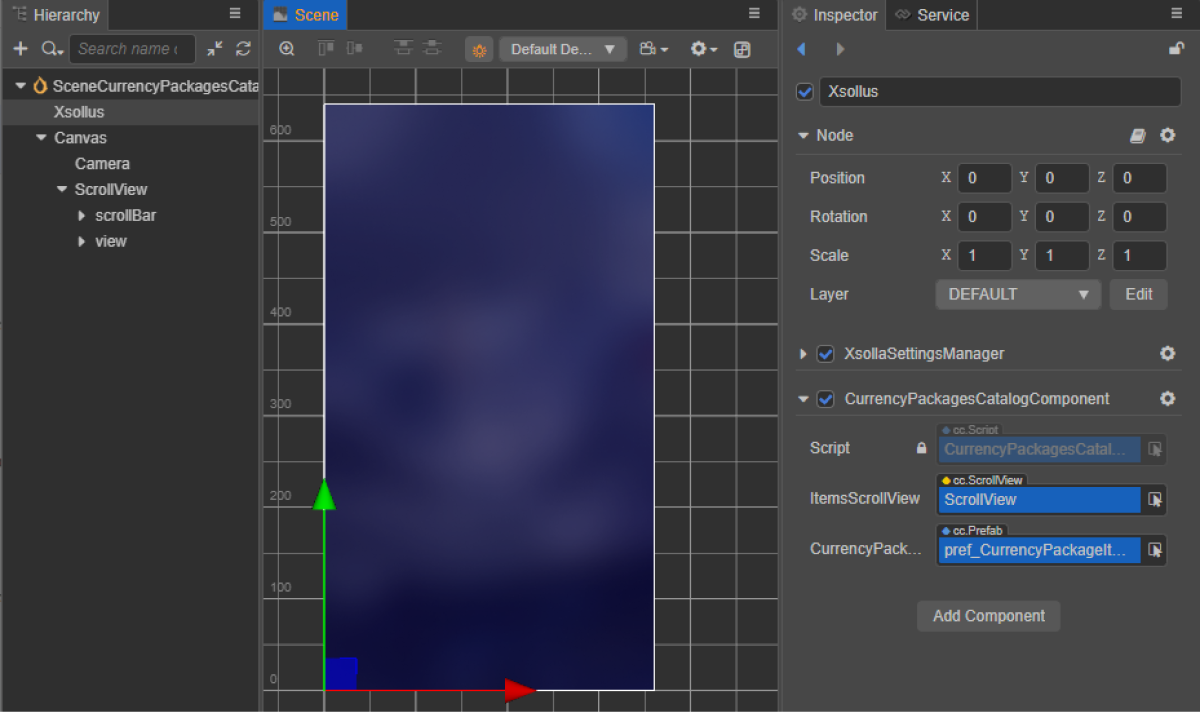
クラススクリプト(CurrencyPackagesCatalogComponent)の例:
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { CurrencyPackageItemComponent } from './CurrencyPackageItemComponent';
const { ccclass, property } = _decorator;
@ccclass('CurrencyPackagesCatalogComponent')
export class CurrencyPackagesCatalogComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
currencyPackageItemPrefab: Prefab;
start() {
XsollaCatalog.getVirtualCurrencyPackages(null, null, [], itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let currencyPackageItem = instantiate(this.currencyPackageItemPrefab);
this.itemsScrollView.content.addChild(currencyPackageItem);
currencyPackageItem.getComponent(CurrencyPackageItemComponent).init(itemsData.items[i]);
}
});
}
}
スクリプトの動作結果の例:
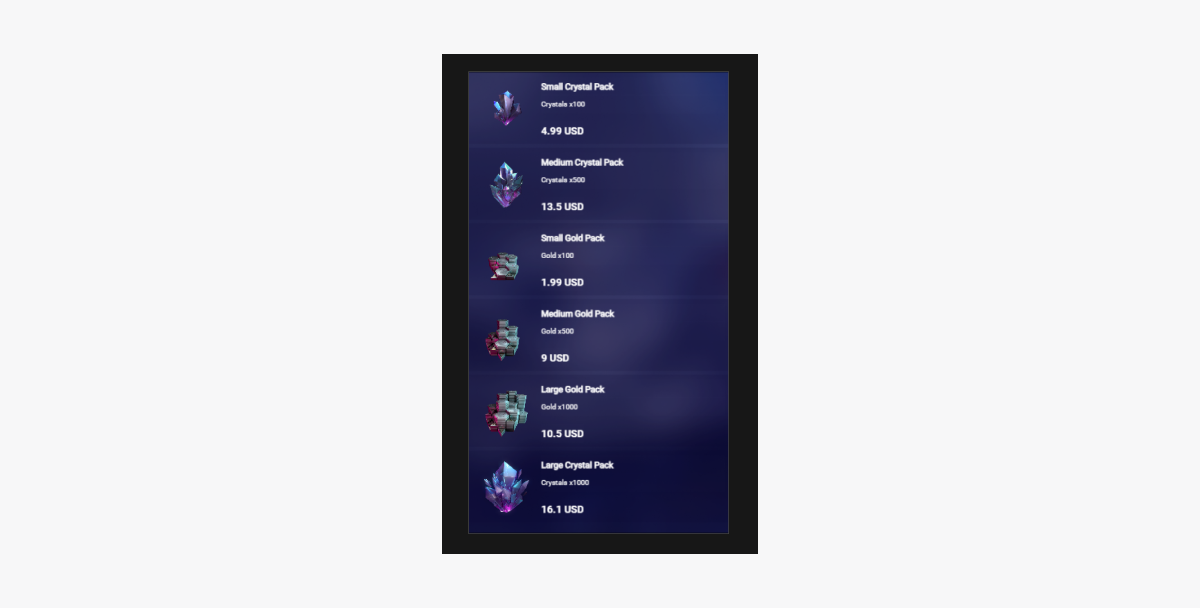
仮想アイテムを実際通貨で販売する
ここでは、SDKメソッドを使用して、仮想アイテムを使用した実際通貨のアイテム販売を実装する方法について説明します。
始める前に、カタログに仮想アイテムの表示を実装します。以下の例では、仮想アイテムの購入を実装する方法を説明します。他のアイテムタイプの設定も同様です。
このチュートリアルでは、以下のロジックの実装について説明します:
ログインするスクリプトの例では、デモアカウントの資格情報を使用します(ユーザー名:xsolla
、パスワード:xsolla
)。このアカウントはデモプロジェクトでのみ使用できます。
スクリプトサンプルには、カタログのアイテムをページごとに表示する(ページネーション)実装は含まれていません。getCatalog
SDKメソッドのoffset
およびlimit
パラメータを使用してページネーションを実装します。1 ページのアイテムの最大数は50です。カタログに50を超えるアイテムがある場合は、ページネーションが必要です。
アイテムウィジェットを完成する
アイテムウィジェットに直接販売ボタンを追加し、その視覚化を構成します。RC_StoreItemComponent
に変更されています。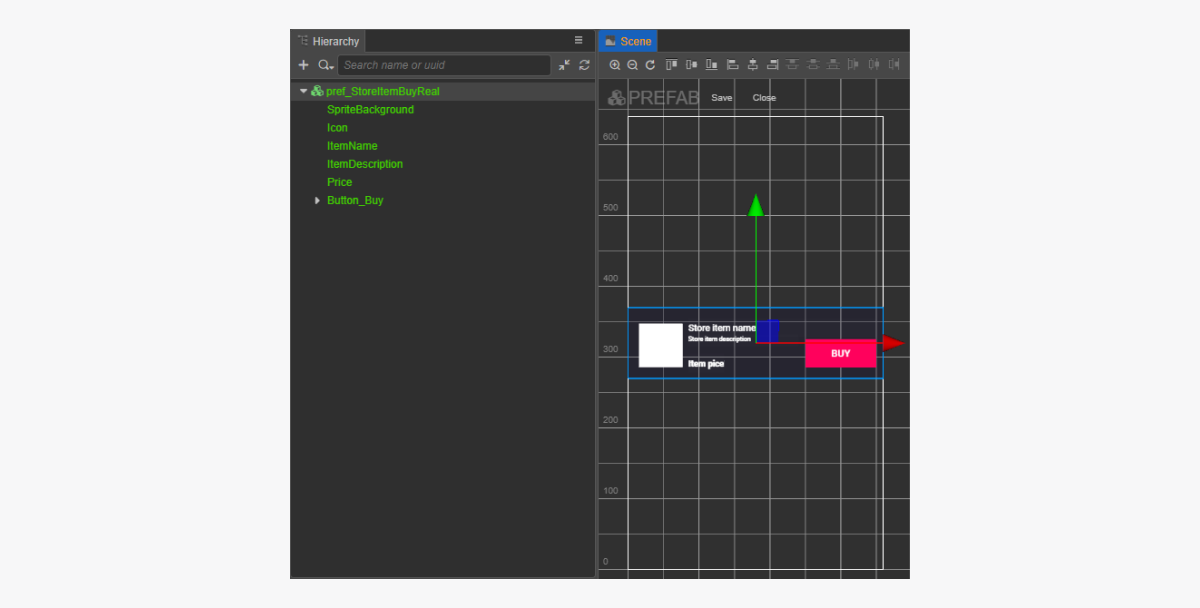
アイテムウィジェットスクリプトを完成する
- コードを使用してコールバック関数を直接販売ボタンにバインドするには、
buyButton
プロパティをRC_StoreItemComponent
に追加します。 - メソッドを
BuyButton
をクリックしたときに呼び出されるRC_StoreItemComponent
クラスを追加し、スクリプトの例に示すように、クリックイベントを処理するロジックを追加します。 - 以下のいずれかの方法でコールバックを直接販売ボタンにバインドします:
- 下の図に示すように、
Inspector パネルを使用する。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
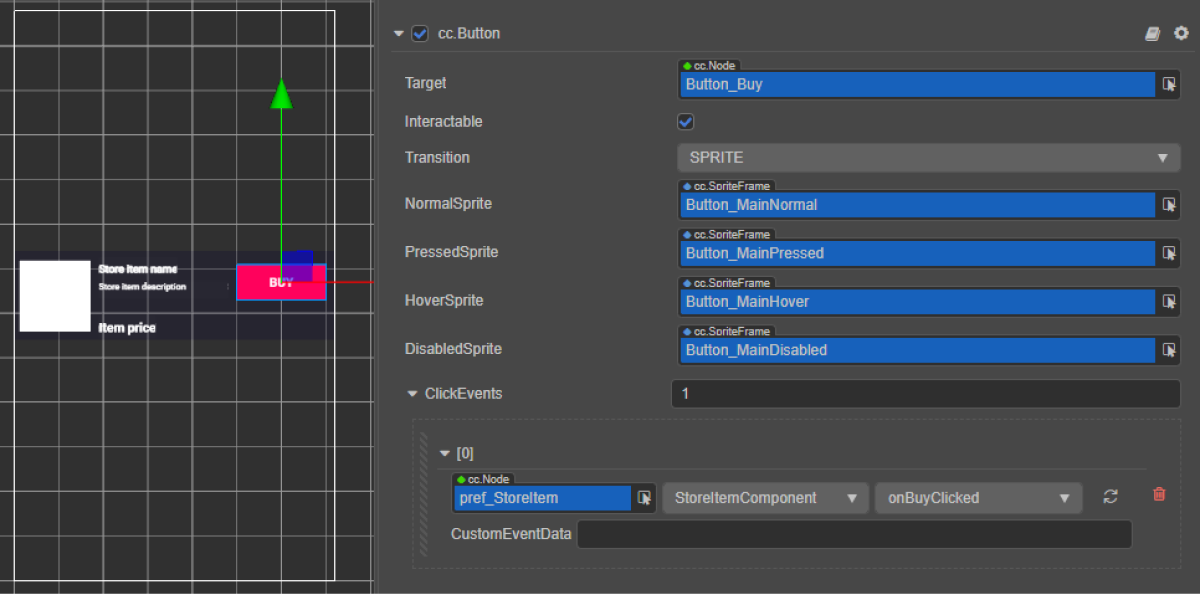
コード経由でコールバックをバインドします:
- typescript
start() {
this.buyButton.node.on(Button.EventType.CLICK, this.onBuyClicked, this);
}
ウィジェットスクリプト(RC_StoreItemComponent)の例:
- typescript
import { _decorator, assetManager, Button, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { StoreItem, XsollaCatalog } from '../../api/XsollaCatalog';
import { TokenStorage } from '../../common/TokenStorage';
import { OrderTracker } from '../../common/OrderTracker';
import { XsollaPayments } from '../../api/XsollaPayments';
const { ccclass, property } = _decorator;
export namespace sellForRealMoneyItem {
@ccclass('RC_StoreItemComponent')
export class RC_StoreItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
itemNameLabel: Label;
@property(Label)
itemDescriptionLabel: Label;
@property(Label)
priceLabel: Label;
@property(Button)
buyButton: Button;
private _data: StoreItem;
start() {
this.buyButton.node.on(Button.EventType.CLICK, this.onBuyClicked, this);
}
init(data: StoreItem) {
this._data = data;
this.itemNameLabel.string = data.name;
this.itemDescriptionLabel.string = data.description;
if (data.virtual_prices.length > 0) {
this.priceLabel.string = data.virtual_prices[0].amount.toString() + ' ' + data.virtual_prices[0].name;
} else {
this.priceLabel.string = parseFloat(data.price.amount) + ' ' + data.price.currency;
}
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Cant load image with url ${data.image_url}`);
}
});
}
onBuyClicked() {
XsollaCatalog.fetchPaymentToken(TokenStorage.getToken().access_token, this._data.sku, 1, undefined, undefined, undefined, undefined, undefined, result => {
OrderTracker.checkPendingOrder(result.token, result.orderId, () => {
console.log('success purchase!');
}, error => {
console.log(`Order checking failed - Status code: ${error.status}, Error code: ${error.code}, Error message: ${error.description}`);
});
XsollaPayments.openPurchaseUI(result.token);
}, error => {
console.log(error.description);
});
}
}
}
アイテムカタログスクリプトコンポーネントを完了する
RC_ItemsCatalogComponent
に変更されています。スクリプトの例に示すように、有効な認可トークンを取得するためのロジックをRC_ItemsCatalogComponent
クラスのstart
メソッドに追加します。
クラススクリプト(RC_ItemsCatalogComponent)の例:
- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { RC_StoreItemComponent } from './RC_StoreItemComponent';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
import { TokenStorage } from 'db://xsolla-commerce-sdk/scripts/common/TokenStorage';
const { ccclass, property } = _decorator;
@ccclass('RC_ItemsCatalogComponent')
export class RC_ItemsCatalogComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
storeItemPrefab: Prefab;
start() {
XsollaAuth.authByUsernameAndPassword('xsolla', 'xsolla', false, token => {
TokenStorage.saveToken(token, false);
XsollaCatalog.getCatalog(null, null, [], itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let storeItem = instantiate(this.storeItemPrefab);
this.itemsScrollView.content.addChild(storeItem);
storeItem.getComponent(RC_StoreItemComponent).init(itemsData.items[i]);
}
});
});
}
}
スクリプトの動作結果の例:
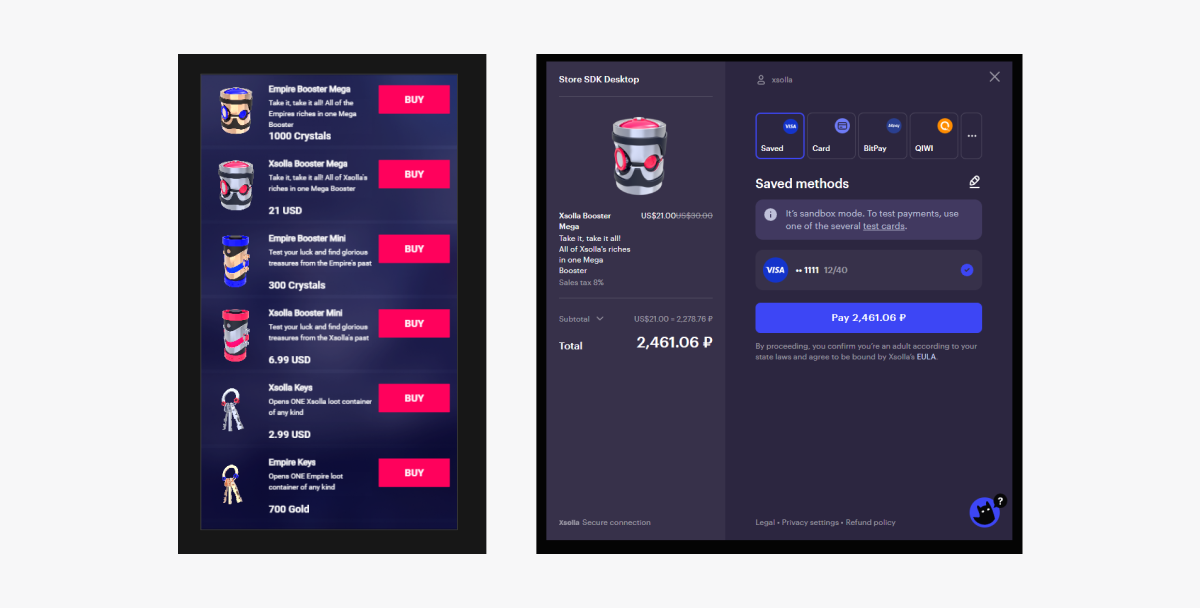
仮想アイテムを仮想通貨で販売する
ここでは、SDKメソッドを使用して、仮想アイテムを使用した仮想通貨のアイテム販売を実装する方法について説明します。
始める前に、カタログに仮想アイテムを表示する機能を実装します。以下の例では、仮想アイテムの購入を実装する方法を説明します。他のアイテムタイプの設定も同様です。
このチュートリアルでは、以下のロジックの実装について説明します:
ログインするスクリプトの例では、デモアカウントの資格情報を使用します(ユーザー名:xsolla
、パスワード:xsolla
)。このアカウントはデモプロジェクトでのみ使用できます。
スクリプトサンプルには、カタログのアイテムをページごとに表示する(ページネーション)実装は含まれていません。getCatalog
SDKメソッドのoffset
およびlimit
パラメータを使用してページネーションを実装します。1ページのアイテムの最大数は50です。カタログに50を超えるアイテムがある場合は、ページネーションが必要です。
アイテムウィジェットを完成する
アイテムウィジェットに直接販売ボタンを追加し、その視覚化を構成します。VC_StoreItemComponent
に変更されています。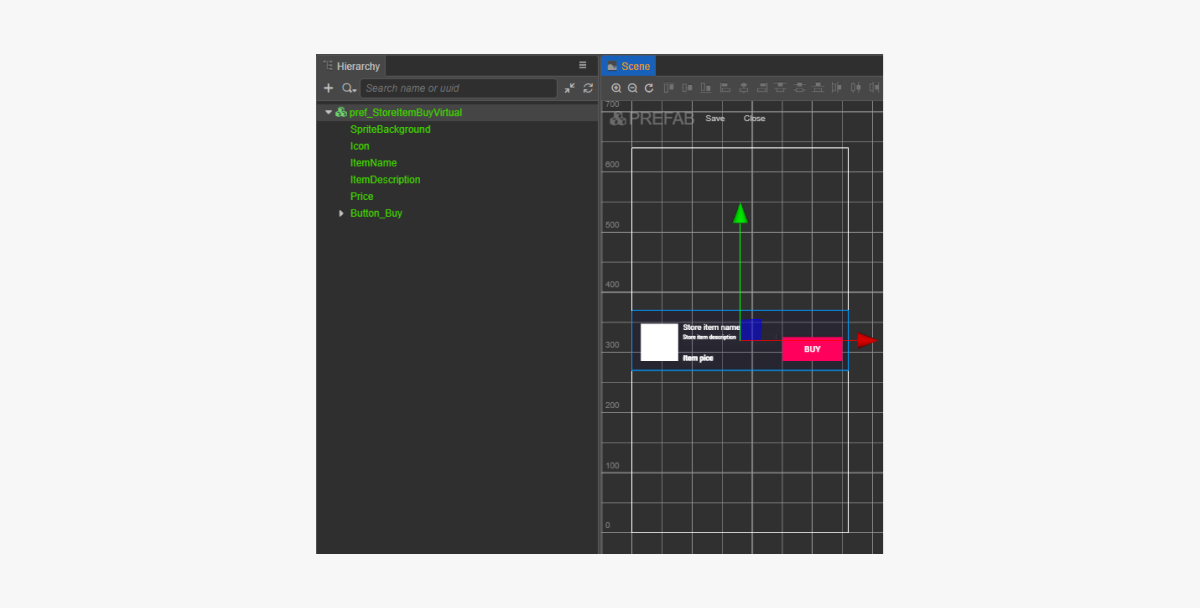
アイテムウィジェットスクリプトを完成する
- コードを使用してコールバック関数を直接販売ボタンにバインドするには、
buyButton
プロパティをVC_StoreItemComponent
に追加します。 - メソッドを
BuyButton
をクリックしたときに呼び出されるVC_StoreItemComponent
クラスを追加し、スクリプトの例に示すように、クリックイベントを処理するロジックを追加します。 - 以下のいずれかの方法でコールバックを直接販売ボタンにバインドします:
- 下の図に示すように、
Inspector パネルを使用する。 - 以下のコードブロックをページスクリプトに挿入する。
- 下の図に示すように、
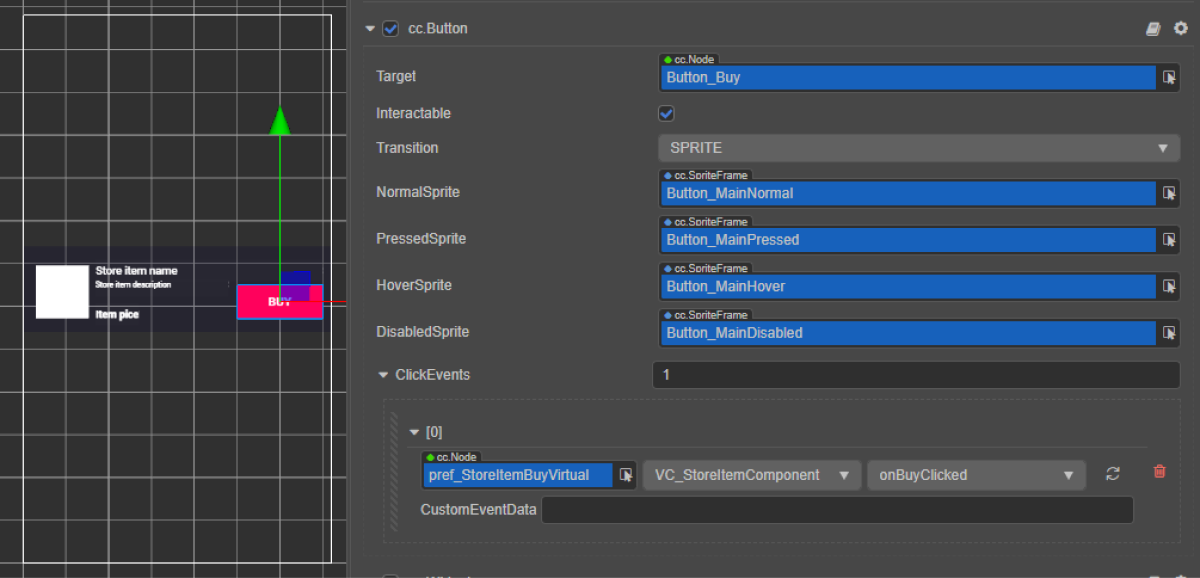
コード経由でコールバックをバインドします:
- typescript
start() {
this.buyButton.node.on(Button.EventType.CLICK, this.onBuyClicked, this);
}
ウィジェットスクリプト(VC_StoreItemComponent)の例:
- typescript
import { _decorator, assetManager, Button, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { StoreItem, XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { TokenStorage } from 'db://xsolla-commerce-sdk/scripts/common/TokenStorage';
import { OrderTracker } from 'db://xsolla-commerce-sdk/scripts/common/OrderTracker';
const { ccclass, property } = _decorator;
@ccclass('VC_StoreItemComponent')
export class VC_StoreItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
itemNameLabel: Label;
@property(Label)
itemDescriptionLabel: Label;
@property(Label)
priceLabel: Label;
@property(Button)
buyButton: Button;
private _data: StoreItem;
start() {
this.buyButton.node.on(Button.EventType.CLICK, this.onBuyClicked, this);
}
init(data: StoreItem) {
this._data = data;
this.itemNameLabel.string = data.name;
this.itemDescriptionLabel.string = data.description;
if (data.virtual_prices.length > 0) {
this.priceLabel.string = data.virtual_prices[0].amount.toString() + ' ' + data.virtual_prices[0].name;
} else {
this.priceLabel.string = parseFloat(data.price.amount) + ' ' + data.price.currency;
}
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
}
onBuyClicked() {
XsollaCatalog.purchaseItemForVirtualCurrency(TokenStorage.getToken().access_token, this._data.sku, this._data.virtual_prices[0].sku, orderId => {
OrderTracker.checkPendingOrder(TokenStorage.getToken().access_token, orderId, () => {
console.log('success purchase!');
}, error => {
console.log(`Order checking failed - Status code: ${error.status}, Error code: ${error.code}, Error message: ${error.description}`);
});
}, error => {
console.log(error.description);
});
}
}
アイテムカタログスクリプトコンポーネントを完了する
VC_ItemsCatalogComponent
に変更されています。スクリプトの例に示すように、有効な認可トークンを取得するためのロジックをVC_ItemsCatalogComponent
クラスのstart
メソッドに追加します。
クラススクリプト(VC_ItemsCatalogComponent)の例:
- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaCatalog } from 'db://xsolla-commerce-sdk/scripts/api/XsollaCatalog';
import { VC_StoreItemComponent } from './VC_StoreItemComponent';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
import { TokenStorage } from 'db://xsolla-commerce-sdk/scripts/common/TokenStorage';
const { ccclass, property } = _decorator;
@ccclass('VC_ItemsCatalogComponent')
export class VC_ItemsCatalogComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
storeItemPrefab: Prefab;
start() {
XsollaAuth.authByUsernameAndPassword('xsolla', 'xsolla', false, token => {
TokenStorage.saveToken(token, false);
XsollaCatalog.getCatalog(null, null, [], itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let storeItem = instantiate(this.storeItemPrefab);
this.itemsScrollView.content.addChild(storeItem);
storeItem.getComponent(VC_StoreItemComponent).init(itemsData.items[i]);
}
});
});
}
}
スクリプトの動作結果の例:
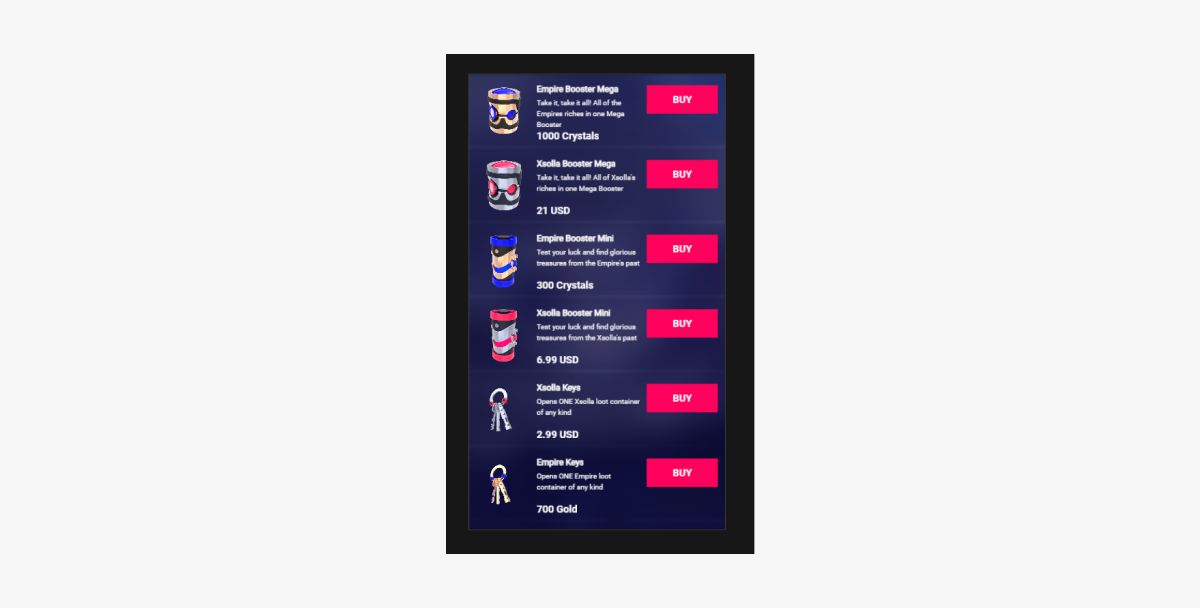
仮想通貨残高の表示
このチュートリアルでは、SDKメソッドを使って、アプリ内で仮想通貨の残高を表示する方法を紹介します。
残高表示用のウィジェットを作成する
- プレハブを作成します。これを行うには、フォルダーのコンテキストメニューから
Create > Node Prefab を選択します。 - 作成したプレハブを開きます。
- 以下の画像に示すように、
UITransform
コンポーネントをプレハブのルートに追加し、コンテンツサイズを設定します。
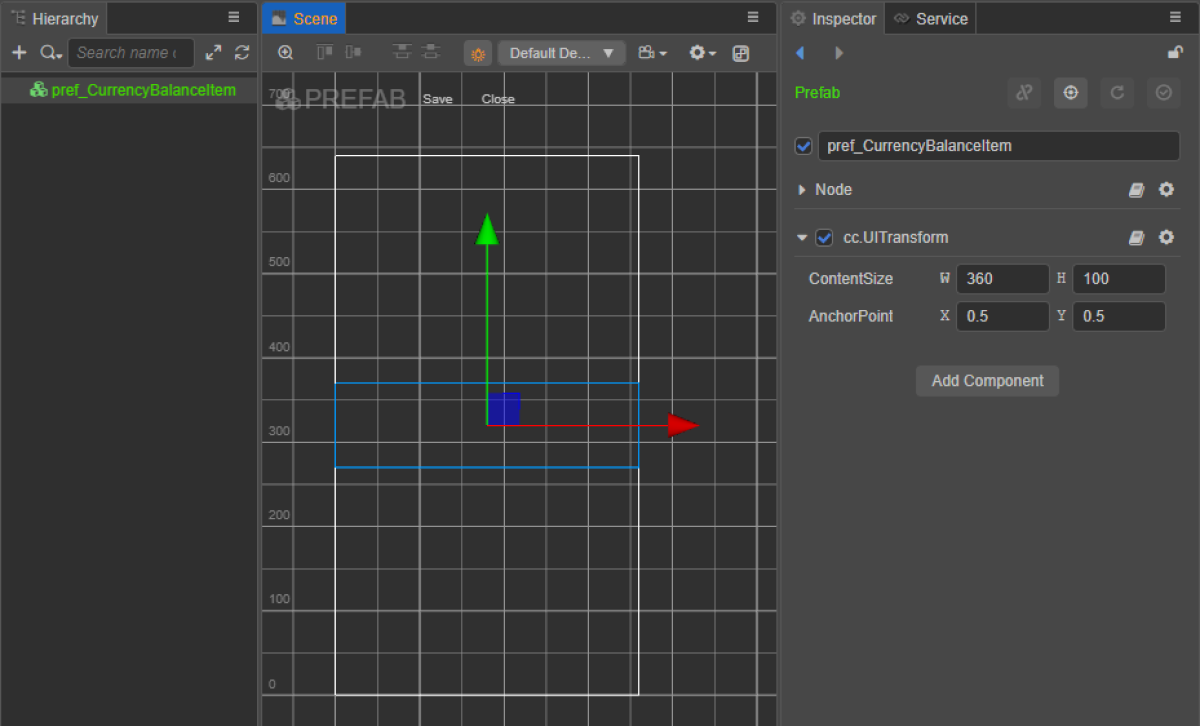
- 以下のUI要素をプレハブ子オブジェクトとして追加し、視覚化を構成します:
- ウィジェットの背景画像
- 通貨名
- 通貨数量
- 通貨イメージ
ウィジェット構造の例:
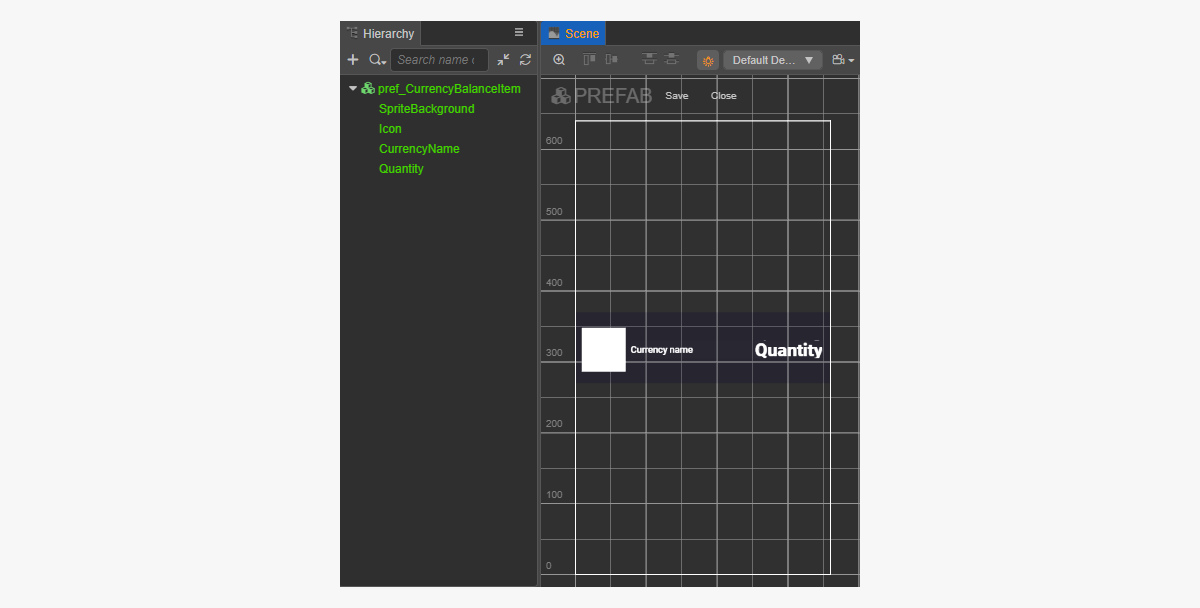
残高表示のためのウィジェットスクリプトを作成する
- CurrencyBalanceItemComponentを作成し、以下のプロパティを追加します:
iconSprite
currencyNameLabel
quantityLabel
- スクリプトの例に示すように、
init
メソッドと初期化ロジックをCurrencyBalanceItemComponent
クラスに追加します。 - CurrencyBalanceItemComponentコンポーネントをプレハブのルートノードにアタッチします。
- 図に示すように、プレハブ要素を
CurrencyBalanceItemComponent
のプロパティにバインドします:
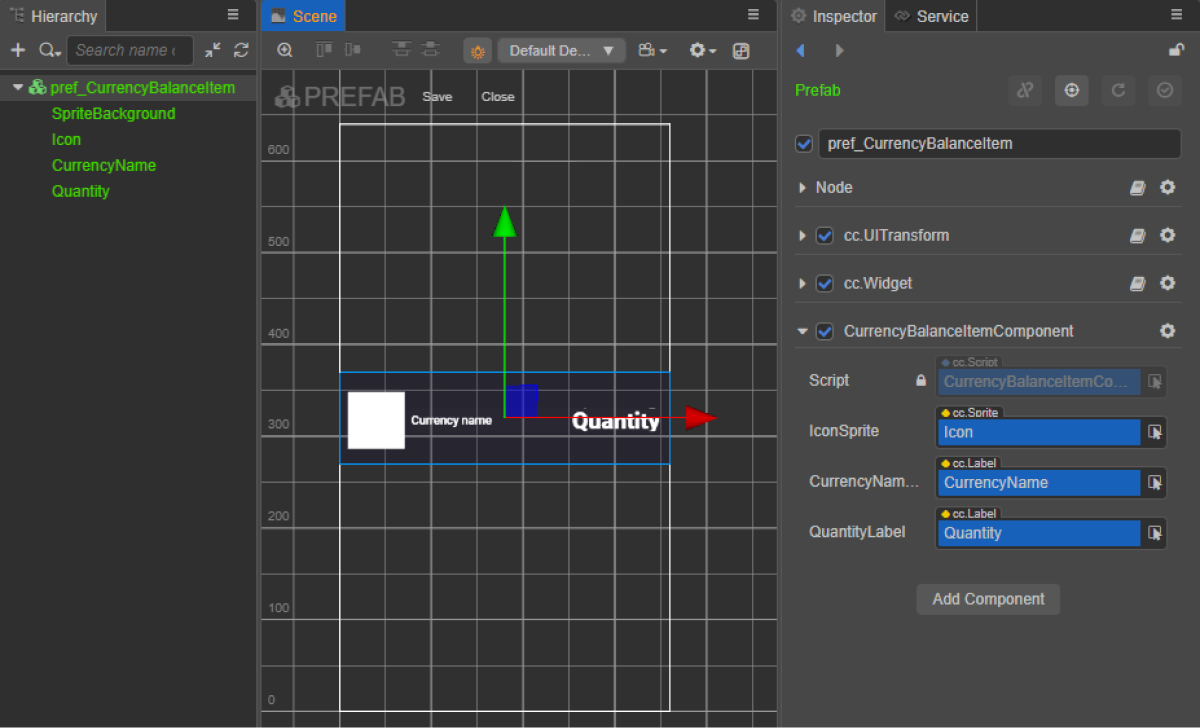
ウィジェットスクリプト(CurrencyBalanceItemComponent)の例:
- typescript
import { _decorator, assetManager, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { VirtualCurrencyBalance } from 'db://xsolla-commerce-sdk/scripts/api/XsollaInventory';
const { ccclass, property } = _decorator;
@ccclass('CurrencyBalanceItemComponent')
export class CurrencyBalanceItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
currencyNameLabel: Label;
@property(Label)
quantityLabel: Label;
init(data: VirtualCurrencyBalance) {
this.currencyNameLabel.string = data.name;
this.quantityLabel.string = data.amount.toString();
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
}
}
ページのインターフェースを作成する
仮想通貨残高ページのシーンを作成し、それにScrollView
要素を追加します。
ページ構造の例:
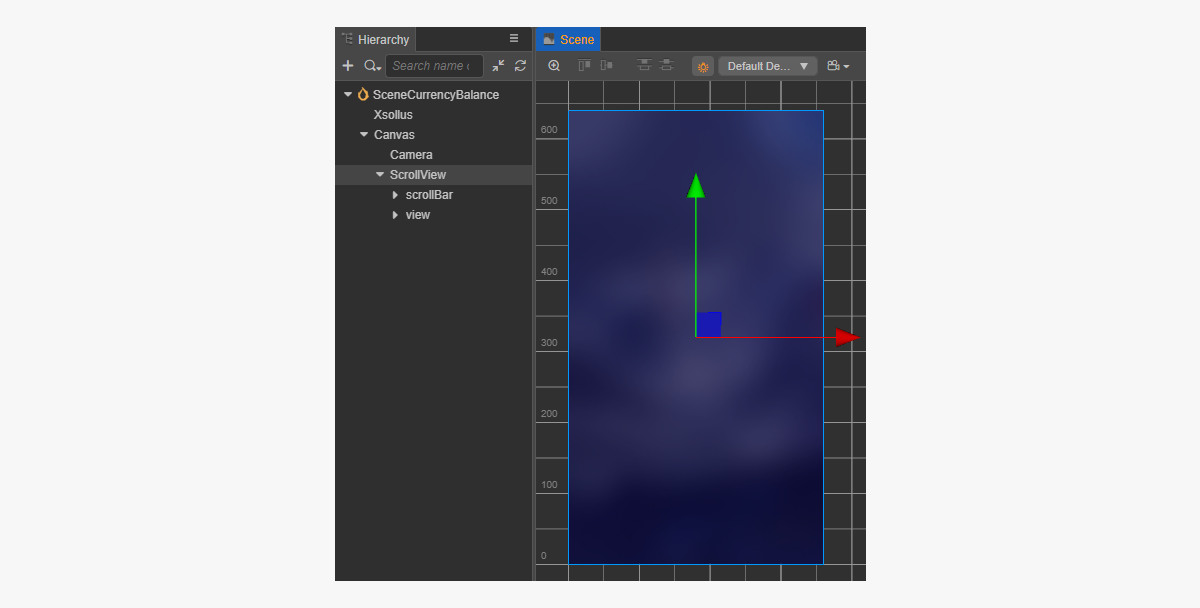
ScrollView
を作成したCurrencyBalanceItem
プレハブと一致させるには、そのサイズを設定します:
ScrollView
ノードと内部のview
ノードのContentSize
パラメータの値を変更します。Layout
コンポーネントをcontent
ノードにバインドして設定します。この例では、次の設定が選択されています:Type == vertical
ResizeMode == Container
通貨残高スクリプトコンポーネントを作成する
- CurrencyBalanceComponentを作成し、以下のプロパティを追加します:
itemsScrollView
currencyBalanceItemPrefab
- スクリプトの例に示すように、
start ライフサイクル関数と初期化ロジックをCurrencyBalanceComponent
クラスに追加します。 CurrencyBalanceComponent をシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加したXsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。- 図に示すように、プレハブ要素を
CurrencyBalanceItemComponent
のプロパティにバインドします:
xsolla
、パスワード:xsolla
)。このアカウントはデモプロジェクトでのみ使用できます。- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
import { XsollaInventory } from 'db://xsolla-commerce-sdk/scripts/api/XsollaInventory';
import { CurrencyBalanceItemComponent } from './CurrencyBalanceItemComponent';
const { ccclass, property } = _decorator;
@ccclass('CurrencyBalanceComponent')
export class CurrencyBalanceComponent extends Component {
@property(ScrollView)
itemsList: ScrollView;
@property(Prefab)
currencyBalanceItemPrefab: Prefab;
start() {
XsollaAuth.authByUsernameAndPassword('xsolla', 'xsolla', false, token => {
XsollaInventory.getVirtualCurrencyBalance(token.access_token, null, itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let currencyBalanceItem = instantiate(this.currencyBalanceItemPrefab);
this.itemsList.content.addChild(currencyBalanceItem);
currencyBalanceItem.getComponent(CurrencyBalanceItemComponent).init(itemsData.items[i]);
}
});
});
}
}
スクリプトの動作結果の例:
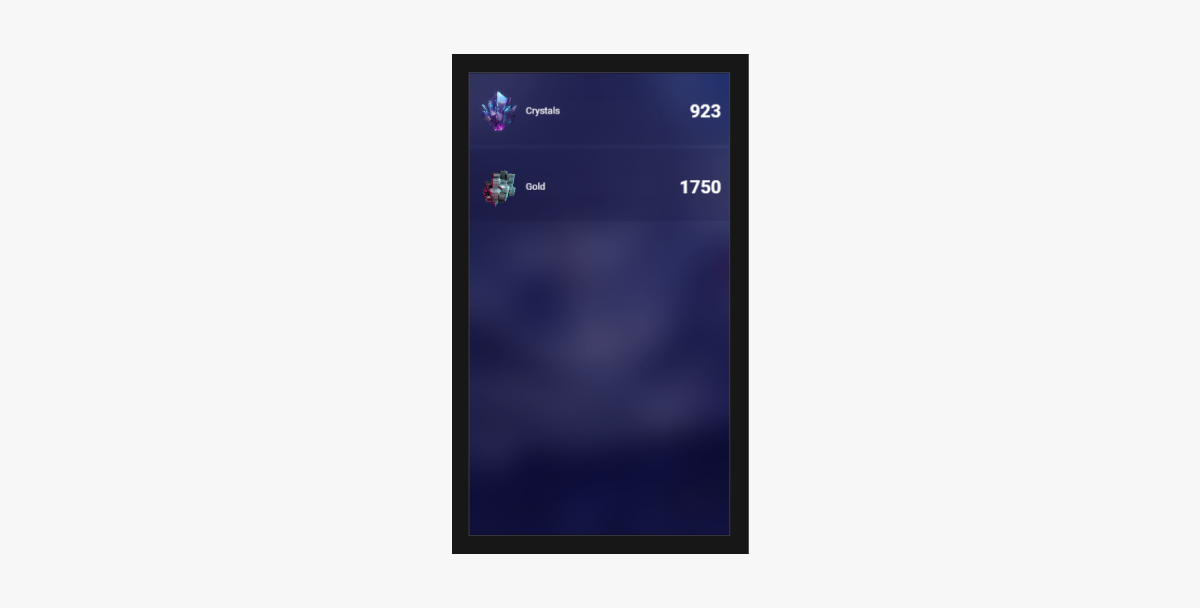
インベントリでアイテムの表示
このチュートリアルでは、SDKのメソッドを使ってユーザーのインベントリにアイテムを表示する方法を紹介します。
アイテムウィジェットを作成する
- プレハブを作成します。これを行うには、フォルダーのコンテキストメニューから
Create > Node Prefab を選択します。 - 作成したプレハブを開きます。
- 以下の画像に示すように、
UITransform
コンポーネントをプレハブのルートに追加し、コンテンツサイズを設定します。
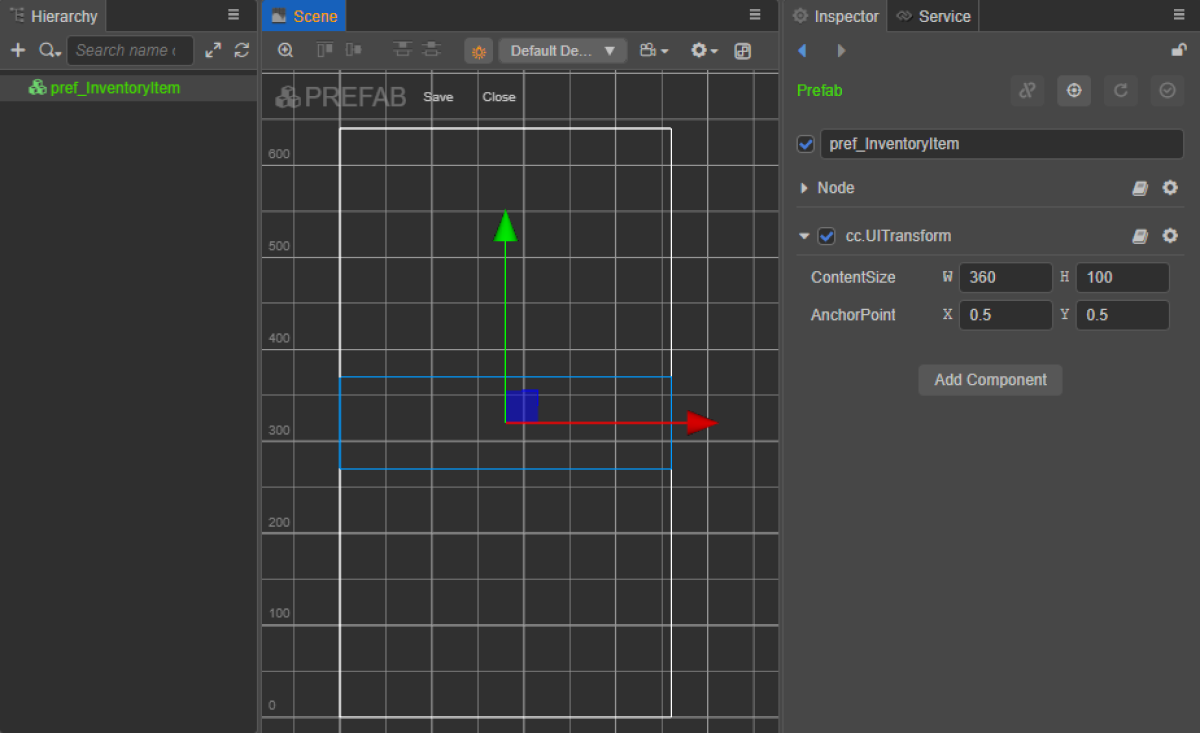
- 以下のUI要素をプレハブ子オブジェクトとして追加し、視覚化を構成します:
- ウィジェットの背景画像
- インベントリアイテム名
- インベントリアイテム説明
- アイテム数量
- アイテムイメージ
ウィジェット構造の例:
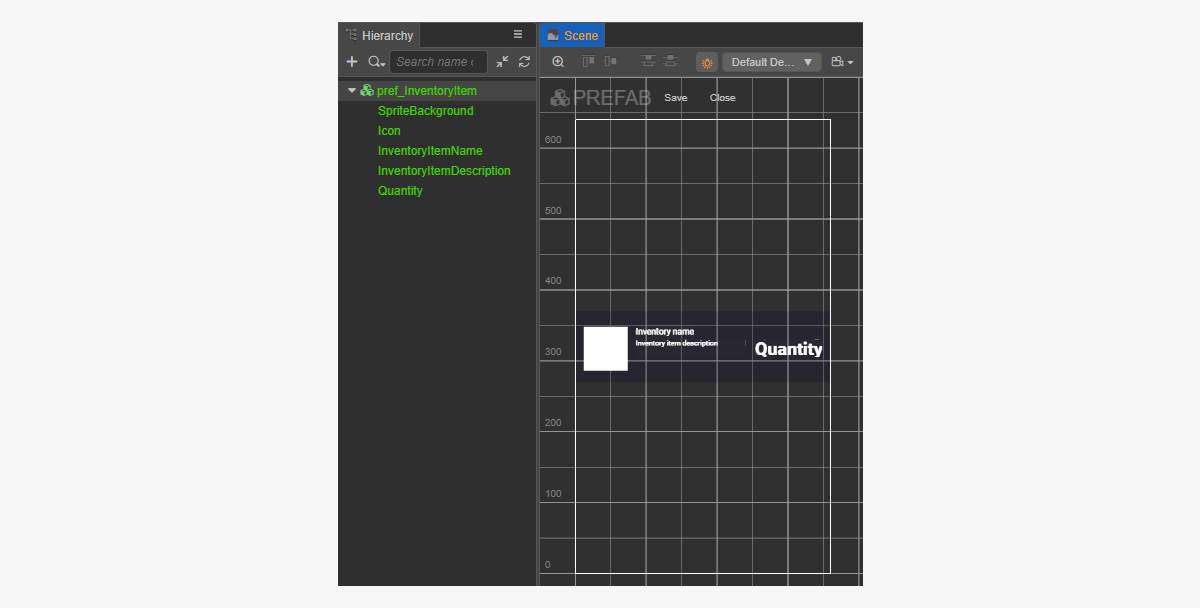
アイテムウィジェットスクリプトを作成する
- InventoryItemComponentを作成し、以下のプロパティを追加します:
iconSprite
itemNameLabel
itemDescriptionLabel
quantityLabel
- スクリプトの例に示すように、
init
メソッドと初期化ロジックをInventoryItemComponent
クラスに追加します。 - InventoryItemComponentコンポーネントをプレハブのルートノードにアタッチします。
- 図に示すように、プレハブ要素を
InventoryItemComponent
のプロパティにバインドします:
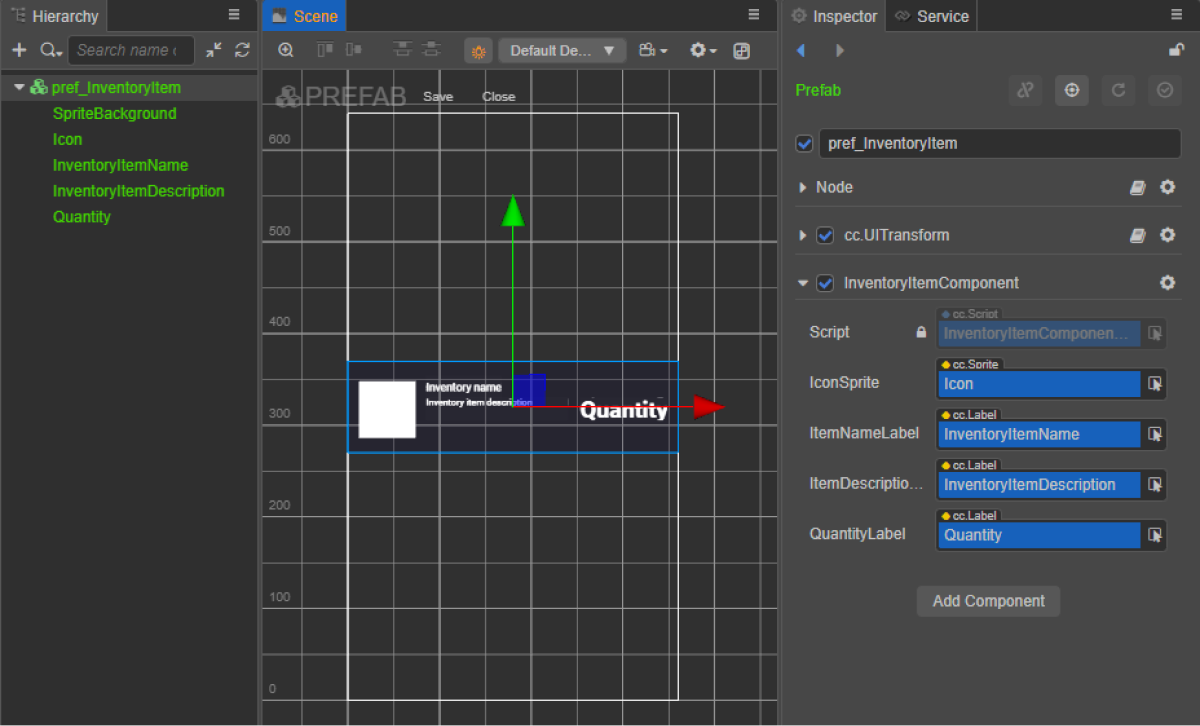
ウィジェットスクリプト(InventoryItemComponent)の例:
- typescript
import { _decorator, assetManager, Component, ImageAsset, Label, Sprite, SpriteFrame, Texture2D } from 'cc';
import { InventoryItem } from 'db://xsolla-commerce-sdk/scripts/api/XsollaInventory';
const { ccclass, property } = _decorator;
@ccclass('InventoryItemComponent')
export class InventoryItemComponent extends Component {
@property(Sprite)
iconSprite: Sprite;
@property(Label)
itemNameLabel: Label;
@property(Label)
itemDescriptionLabel: Label;
@property(Label)
quantityLabel: Label;
init(data: InventoryItem) {
this.itemNameLabel.string = data.name;
this.itemDescriptionLabel.string = data.description;
this.quantityLabel.string = data.quantity.toString();
assetManager.loadRemote<ImageAsset>(data.image_url, (err, imageAsset) => {
if(err == null) {
const spriteFrame = new SpriteFrame();
const texture = new Texture2D();
texture.image = imageAsset;
spriteFrame.texture = texture;
this.iconSprite.spriteFrame = spriteFrame;
} else {
console.log(`Can’t load image with URL ${data.image_url}`);
}
});
}
}
ページのインターフェースを作成する
インベントリページのシーンを作成し、それに ScrollView
要素を追加します。
ページ構造の例:
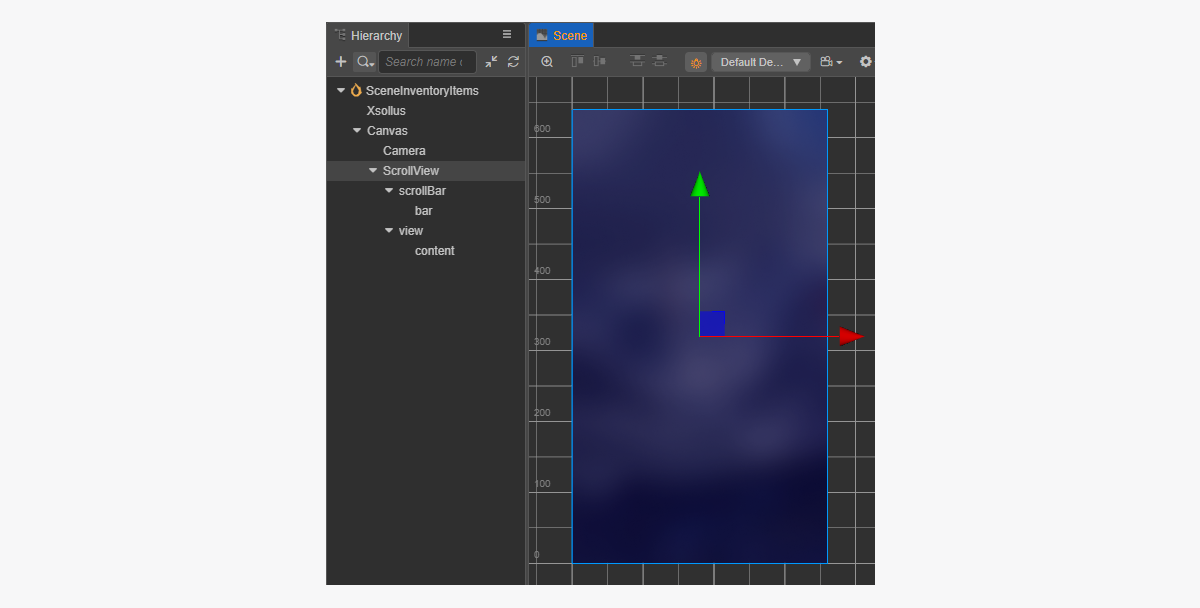
ScrollView
を作成したInventoryItem
プレハブと一致させるには、そのサイズを設定します:
ScrollView
ノードと内部のview
ノードのContentSize
パラメータの値を変更します。Layout
コンポーネントをcontent
ノードにバインドして設定します。この例では、次の設定が選択されています:Type == vertical
ResizeMode == Container
インベントリーページスクリプトコンポーネントを作成する
- InventoryItemsComponentを作成し、以下のプロパティを追加します:
itemsScrollView
inventoryItemPrefab
- スクリプトの例に示すように、
start ライフサイクル関数と初期化ロジックをInventoryItemsComponent
クラスに追加します。 - InventoryItemsComponentをシーン上のノードに追加します。新しいノードを追加することも、SDK初期化中に追加した
XsollaSettingsManager
コンポーネントで既存のノードを使用することもできます。 - 図に示すように、プレハブ要素を
InventoryItemsComponent
のプロパティにバインドします:
xsolla
、パスワード:xsolla
)。このアカウントはデモプロジェクトでのみ使用できます。- typescript
import { _decorator, Component, instantiate, Prefab, ScrollView } from 'cc';
import { XsollaAuth } from 'db://xsolla-commerce-sdk/scripts/api/XsollaAuth';
import { XsollaInventory } from 'db://xsolla-commerce-sdk/scripts/api/XsollaInventory';
import { InventoryItemComponent } from './InventoryItemComponent';
const { ccclass, property } = _decorator;
@ccclass('InventoryItemsComponent')
export class InventoryItemsComponent extends Component {
@property(ScrollView)
itemsScrollView: ScrollView;
@property(Prefab)
inventoryItemPrefab: Prefab;
start() {
XsollaAuth.authByUsernameAndPassword('xsolla', 'xsolla', false, token => {
XsollaInventory.getInventory(token.access_token, null, itemsData => {
for (let i = 0; i < itemsData.items.length; ++i) {
let inventoryItem = instantiate(this.inventoryItemPrefab);
this.itemsScrollView.content.addChild(inventoryItem);
inventoryItem.getComponent(InventoryItemComponent).init(itemsData.items[i]);
}
});
});
}
}
スクリプトの動作結果の例:
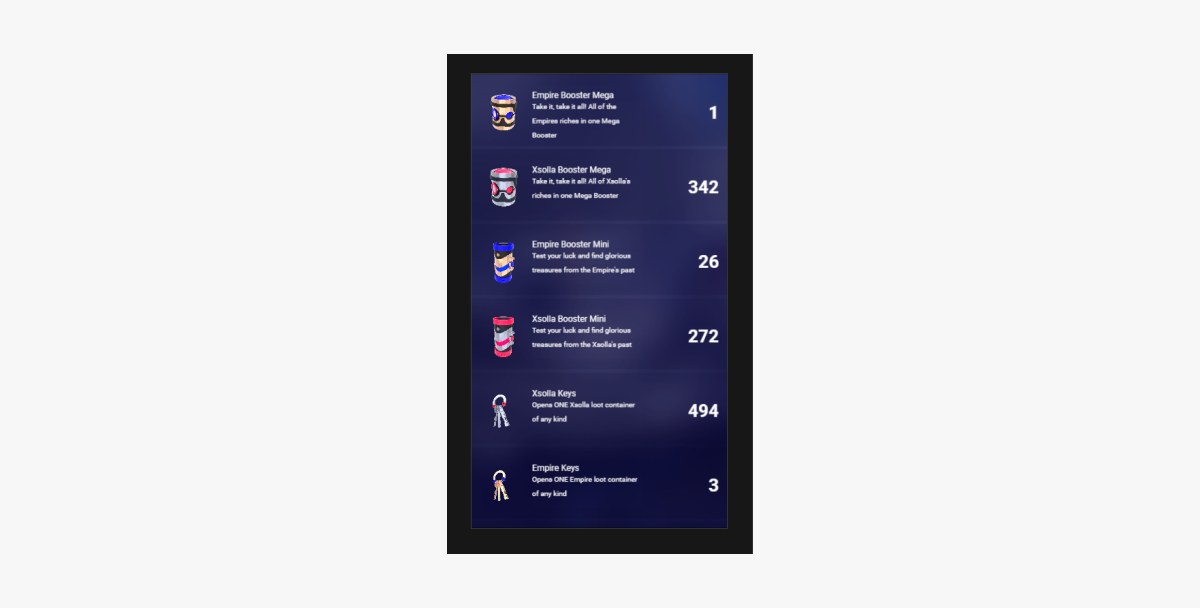
誤字脱字などのテキストエラーを見つけましたか? テキストを選択し、Ctrl+Enterを押します。