How to use Pay Station in combination with Firebase authentication
If you have already implemented user authentication in your application using Firebase, you can generate a payment token on Firebase side and then pass it to the client side of the application to open the payment UI.
Using this integration option, you need to independently implement the logic for determining the user’s country and currency to pay for the purchase.
Integration flow:
- Sign up to Publisher Account and create a new project. You will need the ID of the created project in further steps.
- Set up a catalog:
- Create an item catalog on Xsolla side. You can add items manually or import them from Google Play or PlayFab.
- Implement getting and displaying the catalog on the client side of the application using the
Store library.
- Set up an item purchase:
- Create an order with user and item data on the client side of the application using the Firebase Cloud Function.
- Implement the opening of the payment UI on the client side of your application using the
Payments library.
To complete the integration and start accepting real payments, you need to sign a licensing agreement with Xsolla.
You can sign the licensing agreement at any integration step, but keep in mind that the review process can take up to 3 business days.
Use the sample web application as an example to implement the combined use of Firebase authentication and Pay Station. The source code for the sample web application is available on GitHub.
Create project
Sign up to Publisher Account
Publisher Account is the main tool to configure Xsolla features, as well as to work with analytics and transactions.
The data about the company and your application specified during registration will be used to create a draft licensing agreement with Xsolla and to generate recommendations on solutions that are suitable for you. You can change the data later, but providing the correct data when you sign up will speed up the process of signing the licensing agreement.
To sign up, go to Publisher Account and create an account.
The password from Publisher Account can consist of Latin letters, numerals, and special characters and must contain at least:
- 8 characters
- one digit
- one capital letter
- one lowercase letter
To ensure password security, we recommend:
- changing your password at least once every 90 days
- using a new password that does not match the last 4 passwords on your account
- using a unique password that does not match passwords used anywhere else
- not storing your password where it is easily accessible
- using password managers to store your password
Publisher account uses two-factor authentication and sends a confirmation code with each authentication attempt.
Create project in Publisher Account
If you have multiple applications, we recommend creating a separate project for each application. Based on the data specified during project creation, Xsolla generates recommendations on solutions that are suitable for you.
To create a new project:
- Open Publisher Account.
- In the side menu, click Create project.
- Enter your project name in English (required).
- Select one or several release platforms of your game (required).
- Add a link to your game. If your game doesn’t have a website yet, add a link to the source that includes information about the game (required).
- Select the game engine.
- Select the monetization options you use or plan to use.
- Specify if the game is already released. If the game hasn’t been released yet, specify the planned release date.
- Click Create project. You will see a page with the Xsolla products recommended for you.
During the integration process, you need to provide the project ID that can be found in your Publisher Account next to the project name.
Set up catalog
Create items in Publisher Account
You need to create a catalog on Xsolla side. You can add items manually or import them from Google Play or PlayFab. When importing from Google Play, you can import a maximum of 100 items at a time.
These instructions provide steps for basic setup of a virtual item. Later, you can add other items to your catalog (virtual currency, bundles, game keys), create item groups, set up promotional campaigns, regional prices, etc.
To add a virtual item with basic settings to the catalog:
- Open your project in Publisher Account.
- Click Store in the side menu.
- In the Virtual Items pane, click Connect.
- In the drop-down menu, select Create item.
- Set the basic settings of the item in the following fields:
- Image (optional)
- SKU (item unique ID)
- Item name
- Description (optional)
- Specify item price:
- Set the Price in real currency toggle to On.
- In the Default currency field, change the currency (optional) and specify the item price.
- If you changed the currency in the Default currency field, select the same currency in the Price in real currency field.
- Change the item status to Available.
- Click Create item.
Display catalog on client side of application
- Add the
Store library to your project. To do this, openbuild.gradle
and add the following line to the dependencies section:
implementation("com.xsolla.android:store:latest.release")
- On the client side of the application, add a UI to display the product catalog.
- Implement requesting for an item catalog from Xsolla servers.
XStore.getVirtualItems
method to get a list of virtual items is used. You can also get information about catalog items using other Example (
- kotlin
package com.xsolla.androidsample
import androidx.appcompat.app.AppCompatActivity
import android.os.Bundle
import android.widget.Toast
import androidx.recyclerview.widget.LinearLayoutManager
import androidx.recyclerview.widget.RecyclerView
import com.xsolla.android.store.XStore
import com.xsolla.android.store.callbacks.GetVirtualItemsCallback
import com.xsolla.android.store.entity.response.items.VirtualItemsResponse
import com.xsolla.androidsample.adapter.BuyItemAdapter
class StoreActivity : AppCompatActivity() {
private lateinit var itemsView: RecyclerView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_store)
XStore.init(<projectId>)
initUI()
loadVirtualItems()
}
private fun initUI() {
itemsView = findViewById(R.id.buy_recycler_view)
itemsView.layoutManager = LinearLayoutManager(this)
}
private fun loadVirtualItems() {
val parentActivity = this
XStore.getVirtualItems(object : GetVirtualItemsCallback {
override fun onSuccess(response: VirtualItemsResponse) {
itemsView.adapter = BuyItemAdapter(parentActivity, response.items.filter { item -> item.virtualPrices.isEmpty() && !item.isFree })
}
override fun onError(throwable: Throwable?, errorMessage: String?) {
showNotificationMessage(errorMessage ?: throwable?.javaClass?.name ?: "Error")
}
})
}
private fun showNotificationMessage(message: String) {
Toast.makeText(
baseContext,
message,
Toast.LENGTH_SHORT,
).show()
}
}
In the XStore.init()
initialization block of the script, specify the project ID that you can find in your Publisher Account next to the project name.
Set up item purchase
Create order using the Cloud Functions
To create an order with user and item data on Xsolla side, add a Cloud Function to the project that uses the Create payment token for purchase API call. This call will return a payment token, which is required to open the payment UI and make a purchase.
Limitations:
- You need to pass either the user country or the user’s IP address when requesting the payment token.
- If you don’t pass the currency in the token, it is determined by the country.
- If you pass the currency in the token, the user pays in this currency.
To add a Cloud Function to a project:
- Install the Firebase CLI (Command-Line Interface). To do this, run the CLI command:
npm install -g firebase-tools
- To link your project to the Firebase project, initialize the Firebase project by running the CLI command:
firebase init functions
- Follow the installer’s instructions to configure the settings:
- Select an existing codebase.
- Specify JavaScript as the language for creating Cloud Functions.
- Install dependencies.
- Open
functions/index.js
and modify it:
- javascript
// The Cloud Functions for Firebase SDK to create Cloud Functions and triggers.
const functions = require('firebase-functions/v1');
const projectId = <projectId>;
const apiKey = <apiKey>;
exports.getXsollaPaymentToken = functions.https.onRequest((req, res) => {
const requestBody = req.body;
if (!requestBody) {
res.status(400).send('Request body is missing');
return;
}
const userId = requestBody.data.uid;
const email = requestBody.data.email;
const sku = requestBody.data.sku;
const returnUrl = requestBody.data.returnUrl;
const payload = {
user: {
id: {value: userId},
name: {
value: email
},
email: {
value: email
},
country: {
value: 'US',
allow_modify: false
}
},
purchase: {
items: [
{
sku: sku,
quantity: 1
}
]
},
sandbox: true,
settings: {
language: 'en',
currency: 'USD',
return_url: returnUrl,
ui: {
theme: '63295aab2e47fab76f7708e3'
}
}
}
let url = "https://store.xsolla.com/api/v3/project/" + projectId.toString() + "/admin/payment/token";
fetch(
url,
{
method: "POST",
headers: {
'Content-Type': 'application/json',
Authorization: 'Basic ' + btoa(`${projectId}:${apiKey}`)
},
body: JSON.stringify(payload)
},
)
.then(xsollaRes => {
// Handle the response data
if (xsollaRes.ok) {
return xsollaRes.json();
} else {
throw new Error(`HTTP request failed with status ${xsollaRes.status} and statusText: ${xsollaRes.statusText}`)
}
})
.then(data => {
res.send(JSON.stringify(data));
})
.catch(error => {
res.send("Error = " + error);
});
});
exports.webhookFakeResponse = functions.https.onRequest((request, response) => {
response.status(200).send()
})
- In the script, specify the values for the variables:
projectId
— project ID that you can find in your Publisher Account next to the project name.
apiKey
— API key. It is shown in Publisher Account only once when it is created and must be stored on your side. You can create a new key in the following section:- Company settings > API keys
- Project settings > API keys
- To test the Cloud Function with the emulator, run the CLI command:
firebase emulators:start
- After running the Cloud Function, you can call the following methods on the client side of your application:
getXsollaPaymentToken
— returns the payment token for opening the payment interface.webhookFakeResponse
— sends the HTTP code200
in response to the Payment webhook. The method doesn’t contain purchase validation logic: use it only for testing. For the full list of webhooks and general information about working with them, refer to the webhooks documentation.
- To call methods locally, use the
https://localhost:5001/{firebase-project-id}/us-central1/getXsollaPaymentToken
andhttps://localhost:5001/{firebase-project-id}/us-central1/webhookFakeResponse
URLs, where{firebase-project-id}
is the Firebase project ID (Firebase console > Project Settings > Project ID).
- To deploy the Cloud Function in production, run the CLI command:
firebase deploy --only functions
- Once deployed in production, you can call methods via the
https://us-central1-{firebase-project-id}.cloudfunctions.net/getXsollaPaymentToken
andhttps://us-central1-{firebase-project-id}.cloudfunctions.net/webhookFakeResponse
URLs, where{firebase-project-id}
is the Firebase project ID (Firebase console > Project Settings > Project ID). For details on running the feature in production, refer to the Firebase documentation.
Set up launch of payment UI
- Add the
Payments library to your project. To do this, openbuild.gradle
and add the following line to the dependencies section:
implementation("com.xsolla.android:payments:latest.release")
- Open
AndroidManifest.xml
and add permission for internet access:
- xml
<uses-permission android:name="android.permission.INTERNET" />
- Add the logic for creating an order (calling the
XStore.getXsollaPaymentToken
method of the Cloud Function) and opening the payment UI with the received payment token (XPayments.createIntentBuilder()
class).
- To call the
getXsollaPaymentToken
method, provide one of the following URLs, where{firebase-project-id}
is the Firebase project ID (Firebase console > Project Settings > Project ID):- for local access —
https://localhost:5001/{firebase-project-id}/us-central1/getXsollaPaymentToken
- for access in the production —
https://us-central1-{firebase-project-id}.cloudfunctions.net/getXsollaPaymentToken
- for local access —
Example (
- kotlin
package com.xsolla.androidsample.adapter
import android.R.attr.duration
import android.os.Handler
import android.os.Looper
import android.view.LayoutInflater
import android.view.ViewGroup
import android.widget.Toast
import androidx.recyclerview.widget.RecyclerView
import com.bumptech.glide.Glide
import com.xsolla.android.payments.XPayments
import com.xsolla.android.payments.data.AccessToken
import com.xsolla.android.store.entity.response.items.VirtualItemsResponse
import com.xsolla.androidsample.R
import com.xsolla.androidsample.StoreActivity
import org.json.JSONObject
import java.io.BufferedReader
import java.io.BufferedWriter
import java.io.OutputStream
import java.io.OutputStreamWriter
import java.net.HttpURLConnection
import java.net.URL
class BuyItemAdapter(private val parentActivity: StoreActivity, private val items: List<VirtualItemsResponse.Item>) :
RecyclerView.Adapter<BuyItemViewHolder>() {
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): BuyItemViewHolder {
return BuyItemViewHolder( LayoutInflater.from(parent.context)
.inflate(R.layout.buy_item_sample, parent, false))
}
override fun onBindViewHolder(holder: BuyItemViewHolder, position: Int) {
val item = items[position]
Glide.with(holder.view).load(item.imageUrl).into(holder.itemImage)
holder.itemName.text = item.name
holder.itemDescription.text = item.description
var priceText: String
if(item.virtualPrices.isNotEmpty()) {
priceText = item.virtualPrices[0].getAmountRaw() + " " + item.virtualPrices[0].name
} else {
priceText = item.price?.getAmountRaw() + " " + item.price?.currency.toString()
}
holder.itemPrice.text = priceText
holder.itemButton.setOnClickListener {
Thread {
purchase(item.sku!!)
}.start()
}
}
private fun purchase(sku: String) {
val uid = parentActivity.intent.getStringExtra("uid")
val email = parentActivity.intent.getStringExtra("email")
val jsonBody = JSONObject()
jsonBody.put("data", JSONObject().apply {
put("uid", uid)
put("email", email)
put("sku", sku)
put("returnUrl", "app://xpayment." + parentActivity.packageName)
})
val connection = URL(https://localhost:5001/{firebase-project-id}/us-central1/getXsollaPaymentToken).openConnection() as HttpURLConnection
connection.requestMethod = "POST"
connection.setRequestProperty("Content-Type", "application/json")
connection.doOutput = true
val outputStream: OutputStream = connection.outputStream
val writer = BufferedWriter(OutputStreamWriter(outputStream))
writer.write(jsonBody.toString())
writer.flush()
writer.close()
val responseCode = connection.responseCode
if (responseCode == HttpURLConnection.HTTP_OK) {
val response = connection.inputStream.bufferedReader().use(BufferedReader::readText)
connection.disconnect()
val jsonObject = JSONObject(response)
val token = jsonObject.getString("token")
val orderId = jsonObject.getString("order_id")
val intent = XPayments.createIntentBuilder(parentActivity)
.accessToken(AccessToken(token))
.isSandbox(true)
.build()
parentActivity.startActivityForResult(intent, 1)
} else {
Handler(Looper.getMainLooper()).post {
showNotificationMessage("HTTP request failed with error: $responseCode")
}
}
}
override fun getItemCount() = items.size
private fun showNotificationMessage(message: String) {
Toast.makeText(
parentActivity,
message,
Toast.LENGTH_SHORT,
).show()
}
}
- Add the
onActivityResult()
method to process the payment result.
Example (
- kotlin
override fun onActivityResult(requestCode: Int, resultCode: Int, data: Intent?) {
super.onActivityResult(requestCode, resultCode, data)
if (requestCode == 1) {
val (status, _) = XPayments.Result.fromResultIntent(data)
when (status) {
XPayments.Status.COMPLETED -> showNotificationMessage("Payment completed")
XPayments.Status.CANCELLED -> showNotificationMessage("Payment canceled")
XPayments.Status.UNKNOWN -> showNotificationMessage("Payment error")
}
}
}
Set up order status tracking
Tracking the order status is required to ensure that the payment was successful and to grant items to the user.
Get order status on client side
To subscribe to order status changes on the client side of your application, call the XStore.getOrderStatus
method and pass the following parameters to the method:
listener
— a listener object of theOrderStatusListener
type.orderId
— the order ID received from the purchase via the shopping cart, one-click purchase, or purchase for virtual currency.
For detailed information about how the method works, refer to the Track order status section.
Get order status on server side
The SDK allows you to track the order status on the client side of your application. However, we recommend setting up the Payment webhook handler to receive order information in the back end of your application. This allows you to implement additional validation of completed purchases
For the full list of webhooks and general information about working with them, refer to the webhooks documentation.
To configure webhooks on Xsolla side:
- Open your project in Publisher Account.
- Click Project settings in the side menu and go to the Webhooks section.
- In the Webhook server field, enter the URL to which Xsolla will send webhooks.
For testing, you can specify https://us-central1-{firebase-project-id}.cloudfunctions.net/webhookFakeResponse
, where {firebase-project-id}
is the Firebase project ID (Firebase console > Project Settings > Project ID). In this case, Firebase simulates successful processing of the webhook. For a real project, you need to add purchase validation logic.
To test webhooks, you can also choose any dedicated site, such as webhook.site, or a platform, such as ngrok.
- Copy and save the value from the Secret key field. This key is generated by default and is used to sign webhooks. If you want to change it, click the update icon.
- Click Enable webhooks.
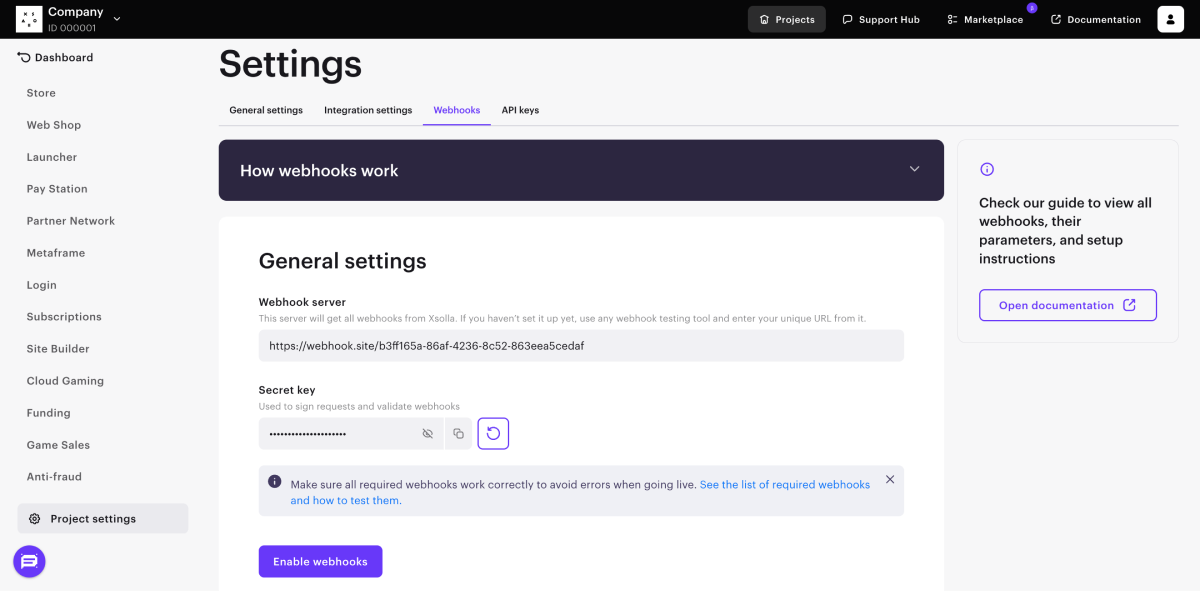
Found a typo or other text error? Select the text and press Ctrl+Enter.